Provide an explanation that justifies the running time of each function in terms of n, where n is the number of students in the list. You can assume the name of each student is of length O(1). HERE ARE THE FUNCTIONS FOR YOU TO EXPLAIN THE RUNNING TIME OF: FUNCTION 1: bool insert_student(int id, char name[], struct slist* lst) { char* studentName = find_student(id, lst); if (studentName == NULL) { // no student with the given id is found, // so we can perform insertion here // creating a new student node struct snode* new_node = (struct snode*) malloc(sizeof(struct snode*)); new_node->id = id; new_node->name = (char*) malloc(strlen(name)*sizeof(char)); strcpy(new_node->name, name); new_node->next = NULL; // if the first node is null if (lst->front == NULL) { lst->front = new_node; } else { struct snode* prevNode = NULL; struct snode* nextNode = lst->front; bool is_node_inserted = false; do { if (id < nextNode->id) { if (prevNode == NULL) { // new node will be the front node new_node->next = nextNode; lst->front = new_node; } else { // node will be inserted between prevNode // and nextNode prevNode->next = new_node; new_node->next = nextNode; } is_node_inserted = true; break; } prevNode = nextNode; nextNode = nextNode->next; } while (nextNode != NULL); if (!is_node_inserted) { // then this node is the last node // prevNode will hold the last node of the list prevNode->next = new_node; } } return true; } else { // student with the same id found // no insertion is possible return false; } } FUNCTION 2: bool remove_student(int id, struct slist* lst) { char* foundName = find_student(id, lst); if (foundName != NULL) { // the student is present then we can remove the student if (lst->front->id == id) { // if the first node is the id to be removed // then set node as first node // and remove the first node struct snode* tempNode = lst->front; lst->front = tempNode->next; free(tempNode); } else { // getting the current node and the next node struct snode* currentNode = lst->front; struct snode* nextNode = currentNode->next; // this bool value is required in case when the last node // is the node to be deleted bool was_student_deleted = false; while (nextNode->next != NULL){ // if the id of next node matches the id to be found if (nextNode->id == id) { // then remove the next node // and set the next of next node as // the next of the current node struct snode* tempNode = nextNode; currentNode->next = tempNode->next; was_student_deleted = true; free(tempNode); break; } currentNode = nextNode; nextNode = nextNode->next; } FUNCTION 3: void free_list(struct slist* lst) { free(lst->front); free(lst); } FUNCTION 4: bool check_for_sorted_list(struct slist* out) { struct snode* currentNode = out->front; int currentId = -1; while (currentNode != NULL) { if (currentId > currentNode->id) { return false; } else { currentId = currentNode->id; } currentNode = currentNode->next; } return true; } FUNCTION 5: struct slist* create_list() { struct slist* new_list = (struct slist*) malloc(sizeof(struct slist*)); new_list->front = NULL; return new_list; } FUNCTION 6: if (!was_student_deleted) { struct snode* tempNode = currentNode->next; currentNode->next = NULL; free(tempNode); } } return true; } else { // the student is not present return false; } } FUNCTION 7: char * find_student(int id, struct slist * lst){ struct snode* nodecurrent = lst -> front; while (nodecurrent != NULL) { // id matches nodecurrent if (nodecurrent -> id == id) { return nodecurrent -> name; } nodecurrent = nodecurrent -> next; } return NULL; }
Provide an explanation that justifies the running time of each function in terms of n, where n is the number of students in the list. You can assume the name of each student is of length O(1).
HERE ARE THE FUNCTIONS FOR YOU TO EXPLAIN THE RUNNING TIME OF:
FUNCTION 1:
bool insert_student(int id, char name[], struct slist* lst) {
char* studentName = find_student(id, lst);
if (studentName == NULL) {
// no student with the given id is found,
// so we can perform insertion here
// creating a new student node
struct snode* new_node = (struct snode*) malloc(sizeof(struct snode*));
new_node->id = id;
new_node->name = (char*) malloc(strlen(name)*sizeof(char));
strcpy(new_node->name, name);
new_node->next = NULL;
// if the first node is null
if (lst->front == NULL) {
lst->front = new_node;
} else {
struct snode* prevNode = NULL;
struct snode* nextNode = lst->front;
bool is_node_inserted = false;
do {
if (id < nextNode->id) {
if (prevNode == NULL) {
// new node will be the front node
new_node->next = nextNode;
lst->front = new_node;
} else {
// node will be inserted between prevNode
// and nextNode
prevNode->next = new_node;
new_node->next = nextNode;
}
is_node_inserted = true;
break;
}
prevNode = nextNode;
nextNode = nextNode->next;
} while (nextNode != NULL);
if (!is_node_inserted) {
// then this node is the last node
// prevNode will hold the last node of the list
prevNode->next = new_node;
}
}
return true;
} else {
// student with the same id found
// no insertion is possible
return false;
}
}
FUNCTION 2:
bool remove_student(int id, struct slist* lst) {
char* foundName = find_student(id, lst);
if (foundName != NULL) {
// the student is present then we can remove the student
if (lst->front->id == id) {
// if the first node is the id to be removed
// then set node as first node
// and remove the first node
struct snode* tempNode = lst->front;
lst->front = tempNode->next;
free(tempNode);
} else {
// getting the current node and the next node
struct snode* currentNode = lst->front;
struct snode* nextNode = currentNode->next;
// this bool value is required in case when the last node
// is the node to be deleted
bool was_student_deleted = false;
while (nextNode->next != NULL){
// if the id of next node matches the id to be found
if (nextNode->id == id) {
// then remove the next node
// and set the next of next node as
// the next of the current node
struct snode* tempNode = nextNode;
currentNode->next = tempNode->next;
was_student_deleted = true;
free(tempNode);
break;
}
currentNode = nextNode;
nextNode = nextNode->next;
}
FUNCTION 3:
void free_list(struct slist* lst) {
free(lst->front);
free(lst);
}
FUNCTION 4:
bool check_for_sorted_list(struct slist* out) {
struct snode* currentNode = out->front;
int currentId = -1;
while (currentNode != NULL) {
if (currentId > currentNode->id) {
return false;
} else {
currentId = currentNode->id;
}
currentNode = currentNode->next;
}
return true;
}
FUNCTION 5:
struct slist* create_list() {
struct slist* new_list = (struct slist*) malloc(sizeof(struct slist*));
new_list->front = NULL;
return new_list;
}
FUNCTION 6:
if (!was_student_deleted) {
struct snode* tempNode = currentNode->next;
currentNode->next = NULL;
free(tempNode);
}
}
return true;
} else {
// the student is not present
return false;
}
}
FUNCTION 7:
char * find_student(int id, struct slist * lst){
struct snode* nodecurrent = lst -> front;
while (nodecurrent != NULL) {
// id matches nodecurrent
if (nodecurrent -> id == id) {
return nodecurrent -> name;
}
nodecurrent = nodecurrent -> next;
}
return NULL;
}

Step by step
Solved in 2 steps

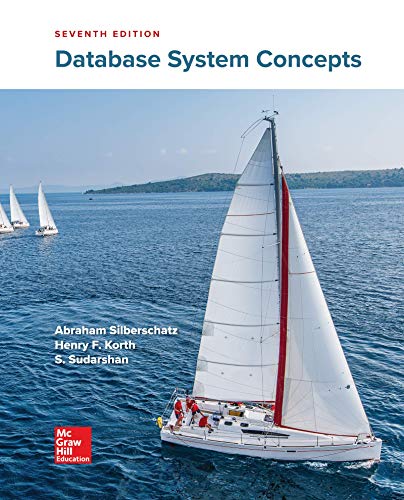
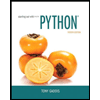
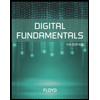
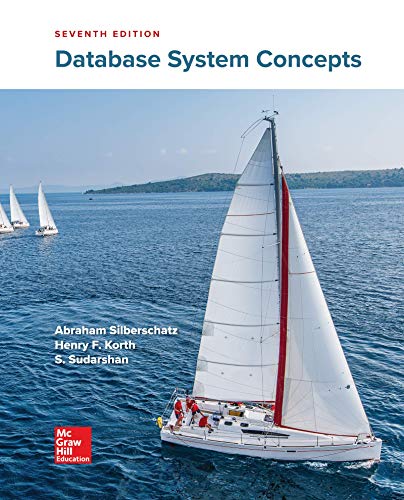
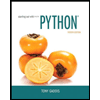
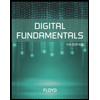
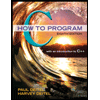
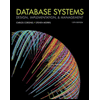
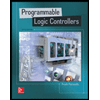