Provide full C++ Code: Your code must have the following three files: Playlist.hpp - Class declaration for a linked list of Nodes (very similar to lab 08 but with some changes to methods, as described below) with following private data members: private: Node* first; Node* last; int size; Playlist.cpp - Class definition main.cpp - main() function Checkpoint A Build a playlist (of songs) by reading data members of several songs from a file and printing them. Implement the following methods: PlayList(); //Default constructor PlayList(string filename); //Parameterized constructor that reads data members of song from a file and builds the PlayList ~PlayList(); //Deletes all nodes of the linked list bool InsertNodeLast(Node *myNewNode); //Inserts myNewNode at the end of the linked list bool InsertNodeFirst(Node *myNewNode); //Inserts myNewNode at the start of the linked list bool DeleteFirst(); //Deletes the first node of the linked list int Size() const { return size; } friend ostream& operator<< (ostream& out, const PlayList& LL); //Prints the linked list PlayList& operator=(const PlayList& other); //Overload the assignment operator such that the current PlayList is reverse of the other PlayLis Notes: InsertNodeLast and InsertNodeFirst are very similar to InsertFirst and InsertLast from Lab 08 except that these functions get a Node (to be inserted) as a parameter. DeleteFirst should be identical to that of Lab 08 The parameterized constructor needs to read data from the file (for each song), create a new node, and call InsertNodeLast to insert the node in the list. The destructor deletes all nodes by repeatedly calling DeleteFirst (exactly the same as lab 08) The implementation of << operator can be inferred from the desired output (see below) The assignment operator is virtually the same as that in lab 08 except for two important changes Instead of inserting nodes from the other list sequentially, it needs to insert them in the reverse order (which method can be used for that?). This does not extend to the case when the current playlist is the same as the other playlist. In other words, if the current and the other playlist are the same, simply return. It must create a new node (copying attributes from the node in the other list) before inserting it into the list Testing and output Running it should give the following output: "Peg" by Steely Dan is 237 seconds long. "All For You" by Janet Jackson is 391 seconds long. "Black Eagle" by Janet Jackson is 197 seconds long. Reversing the play list "Black Eagle" by Janet Jackson is 197 seconds long. "All For You" by Janet Jackson is 391 seconds long. "Peg" by Steely Dan is 237 seconds long.
Provide full C++ Code:
Your code must have the following three files:
- Playlist.hpp - Class declaration for a linked list of Nodes (very similar to lab 08 but with some changes to methods, as described below) with following private data members:
private:
Node* first;
Node* last;
int size;
- Playlist.cpp - Class definition
- main.cpp - main() function
Checkpoint A
Build a playlist (of songs) by reading data members of several songs from a file and printing them.
Implement the following methods:
PlayList(); //Default constructor
PlayList(string filename); //Parameterized constructor that reads data members of song from a file and builds the PlayList
~PlayList(); //Deletes all nodes of the linked list
bool InsertNodeLast(Node *myNewNode); //Inserts myNewNode at the end of the linked list
bool InsertNodeFirst(Node *myNewNode); //Inserts myNewNode at the start of the linked list
bool DeleteFirst(); //Deletes the first node of the linked list
int Size() const { return size; }
friend ostream& operator<< (ostream& out, const PlayList& LL); //Prints the linked list
PlayList& operator=(const PlayList& other); //Overload the assignment operator such that the current PlayList is reverse of the other PlayLis
Notes:
- InsertNodeLast and InsertNodeFirst are very similar to InsertFirst and InsertLast from Lab 08 except that these functions get a Node (to be inserted) as a parameter.
- DeleteFirst should be identical to that of Lab 08
- The parameterized constructor needs to read data from the file (for each song), create a new node, and call InsertNodeLast to insert the node in the list.
- The destructor deletes all nodes by repeatedly calling DeleteFirst (exactly the same as lab 08)
- The implementation of << operator can be inferred from the desired output (see below)
- The assignment operator is virtually the same as that in lab 08 except for two important changes
- Instead of inserting nodes from the other list sequentially, it needs to insert them in the reverse order (which method can be used for that?).
- This does not extend to the case when the current playlist is the same as the other playlist. In other words, if the current and the other playlist are the same, simply return.
- It must create a new node (copying attributes from the node in the other list) before inserting it into the list
- Instead of inserting nodes from the other list sequentially, it needs to insert them in the reverse order (which method can be used for that?).
Testing and output
Running it should give the following output:
"Peg" by Steely Dan is 237 seconds long.
"All For You" by Janet Jackson is 391 seconds long.
"Black Eagle" by Janet Jackson is 197 seconds long.
Reversing the play list
"Black Eagle" by Janet Jackson is 197 seconds long.
"All For You" by Janet Jackson is 391 seconds long.
"Peg" by Steely Dan is 237 seconds long.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

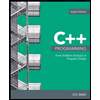
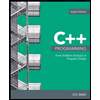