public class CircleAndSphereWhileLoop 3 { public static final doubl MAX_RADIUS = 500.0; 14 %3D 15 public static void main(String[] args) 16 17 Scanner in = new Scanner(System.in); 18 19 // Step 2: Read a double value as radius using prompt 20 // "Enter the radius (between 0.0 and 500.0, exclusive): 21 22 23 // Step 3: While the input radius is not in the ragne (0.0, 500.0) 24 // Display a message on one line (ssuming input value -1) "The input number -1.00 is out of range. Read a double value as radius using the same promt 25 26 // 27 28 29 double circumference = 2 * Math.PI * radius; double area = Math.PI * radius * radius; double surfaceArea = 4 Math.PI Math.pow(radius, 2); double volume = (4 / 3.0) * Math.PI * Math.pow(radius, 3); 30 31 32 33 %3D 34 // Step 4: Display the radius, circle circumference, circle area, 35 // sphere surface area, and sphere volume on separate lines with 6 decimal digits 36 37 // Sample output // The radius // The circle circumference: 3141.529822. // The circle area // The sphere surface area : 3141466.991100. //The sphere volume 38 : 499.990000. 39 40 : 785366.747785. 41 42 : 523567360.300077. 43 44 45
public class CircleAndSphereWhileLoop 3 { public static final doubl MAX_RADIUS = 500.0; 14 %3D 15 public static void main(String[] args) 16 17 Scanner in = new Scanner(System.in); 18 19 // Step 2: Read a double value as radius using prompt 20 // "Enter the radius (between 0.0 and 500.0, exclusive): 21 22 23 // Step 3: While the input radius is not in the ragne (0.0, 500.0) 24 // Display a message on one line (ssuming input value -1) "The input number -1.00 is out of range. Read a double value as radius using the same promt 25 26 // 27 28 29 double circumference = 2 * Math.PI * radius; double area = Math.PI * radius * radius; double surfaceArea = 4 Math.PI Math.pow(radius, 2); double volume = (4 / 3.0) * Math.PI * Math.pow(radius, 3); 30 31 32 33 %3D 34 // Step 4: Display the radius, circle circumference, circle area, 35 // sphere surface area, and sphere volume on separate lines with 6 decimal digits 36 37 // Sample output // The radius // The circle circumference: 3141.529822. // The circle area // The sphere surface area : 3141466.991100. //The sphere volume 38 : 499.990000. 39 40 : 785366.747785. 41 42 : 523567360.300077. 43 44 45
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![15
public static void main(String[] args)
16
17
Scanner in = new Scanner(System.in);
18
19
// Step 2: Read a double value as radius using prompt
20
//
"Enter the radius (between 0.0 and 500.0, exclusive):
21
22
23
// Step 3: While the input radius is not in the ragne (0.0, 500.0)
24
25
//
//
Display a message on one line (ssuming input value -1)
"The input number -1.00 is out of range.
Read a double value as radius using the same promt
26
27
28
29
double circumference
double area = Math.PI * radius * radius;
double surfaceArea = 4 * Math.PI * Math.pow(radius, 2);
double volume = (4 / 3.0) * Math.PI * Math.pow(radius, 3);
30
= 2 * Math.PI * radius;
31
%3D
32
%3D
33
%3D
34
// Step 4: Display the radius, circle circumference, circle area,
35
//
sphere surface area, and sphere volume on separate
lines with 6 decimal digits
36
37
// Sample output
// The radius
// The circle circumference: 3141.529822.
// The circle area
38
39
: 499.990000.
40
: 785366.747785.
41
// The sphere surface area : 3141466.991100.
// The sphere volume
42
43
:523567360.300077.
44
45
46
47}
48](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F170cb9b5-2292-4ecc-9f24-3c60291ff66d%2F760f03a6-eded-4616-8e0f-8502655ba347%2Fwdgnppn_processed.jpeg&w=3840&q=75)
Transcribed Image Text:15
public static void main(String[] args)
16
17
Scanner in = new Scanner(System.in);
18
19
// Step 2: Read a double value as radius using prompt
20
//
"Enter the radius (between 0.0 and 500.0, exclusive):
21
22
23
// Step 3: While the input radius is not in the ragne (0.0, 500.0)
24
25
//
//
Display a message on one line (ssuming input value -1)
"The input number -1.00 is out of range.
Read a double value as radius using the same promt
26
27
28
29
double circumference
double area = Math.PI * radius * radius;
double surfaceArea = 4 * Math.PI * Math.pow(radius, 2);
double volume = (4 / 3.0) * Math.PI * Math.pow(radius, 3);
30
= 2 * Math.PI * radius;
31
%3D
32
%3D
33
%3D
34
// Step 4: Display the radius, circle circumference, circle area,
35
//
sphere surface area, and sphere volume on separate
lines with 6 decimal digits
36
37
// Sample output
// The radius
// The circle circumference: 3141.529822.
// The circle area
38
39
: 499.990000.
40
: 785366.747785.
41
// The sphere surface area : 3141466.991100.
// The sphere volume
42
43
:523567360.300077.
44
45
46
47}
48
![12 public class CircleAndSphereWhileLoop
13 {
public static final doublğ MAX_RADIUS
14
500.0;
15
public static void main(String[] args)
16
17
Scanner in = new Scanner(System.in);
18
19
// Step 2: Read a double value as radius using prompt
20
//
"Enter the radius (between 0.0 and 500.0, exclusive):
21
22
23
// Step 3: While the input radius is not in the ragne (0.0, 500.0)
24
Display a message on one line (ssuming input value -1)
"The input number -1.00 is out of range.
Read a double value as radius using the same promt
25
//
//
26
27
28
29
double circumference = 2 * Math.PI * radius;
double area = Math.PI * radius * radius;
double surfaceArea = 4 * Math.PI * Math.pow(radius, 2);
double volume = (4 / 3.8) * Math.PI * Math.pow(radius, 3);
30
31
%3D
32
33
%3D
34
// Step 4: Display the radius, circle circumference, circle area,
//
35
sphere surface area, and sphere volume on separate
lines with 6 decimal digits
36
37
//
// Sample output
// The radius
// The circle circumference: 3141.529822.
// The circle area
// The sphere surface area : 3141466.991100.
// The sphere volume
38
: 499.990000.
39
40
: 785366.747785.
41
42
43
:523567360.300077.
44
45](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F170cb9b5-2292-4ecc-9f24-3c60291ff66d%2F760f03a6-eded-4616-8e0f-8502655ba347%2Fkrhjh_processed.jpeg&w=3840&q=75)
Transcribed Image Text:12 public class CircleAndSphereWhileLoop
13 {
public static final doublğ MAX_RADIUS
14
500.0;
15
public static void main(String[] args)
16
17
Scanner in = new Scanner(System.in);
18
19
// Step 2: Read a double value as radius using prompt
20
//
"Enter the radius (between 0.0 and 500.0, exclusive):
21
22
23
// Step 3: While the input radius is not in the ragne (0.0, 500.0)
24
Display a message on one line (ssuming input value -1)
"The input number -1.00 is out of range.
Read a double value as radius using the same promt
25
//
//
26
27
28
29
double circumference = 2 * Math.PI * radius;
double area = Math.PI * radius * radius;
double surfaceArea = 4 * Math.PI * Math.pow(radius, 2);
double volume = (4 / 3.8) * Math.PI * Math.pow(radius, 3);
30
31
%3D
32
33
%3D
34
// Step 4: Display the radius, circle circumference, circle area,
//
35
sphere surface area, and sphere volume on separate
lines with 6 decimal digits
36
37
//
// Sample output
// The radius
// The circle circumference: 3141.529822.
// The circle area
// The sphere surface area : 3141466.991100.
// The sphere volume
38
: 499.990000.
39
40
: 785366.747785.
41
42
43
:523567360.300077.
44
45
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
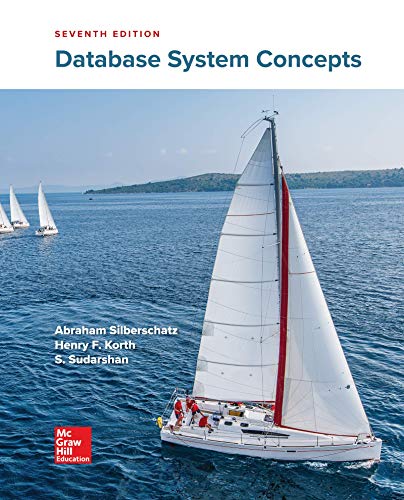
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
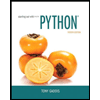
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
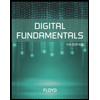
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
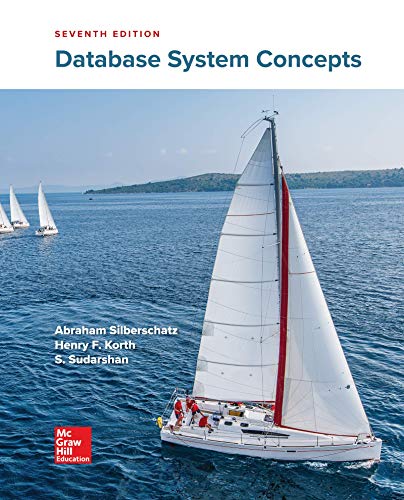
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
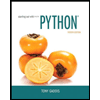
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
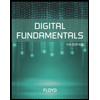
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
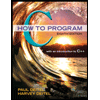
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
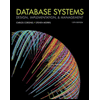
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
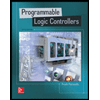
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education