public class TestWord { public static void main(String[] args) { /* Word w1 = new Word("Go Cubs Go", "Cub"); System.out.println("New Word object"); System.out.println("Superclass word: " + w1.getSentence()); System.out.println("Subclass word: " + w1.getNoVowelsWord()); System.out.println("Is word a substring of sentence? " + w1.isSubstring()); System.out.println(); Word w2 = new Word("Applepie", "Apple"); System.out.println("New Word object"); System.out.println("Superclass word: " + w2.getSentence()); System.out.println("Subclass word: " + w2.getNoVowelsWord()); System.out.println("Is word a substring of sentence? " + w2.isSubstring()); System.out.println(); System.out.println("w1 and w2 are the same? " + w1.equals(w2)); */ } }
public class TestWord { public static void main(String[] args) { /* Word w1 = new Word("Go Cubs Go", "Cub"); System.out.println("New Word object"); System.out.println("Superclass word: " + w1.getSentence()); System.out.println("Subclass word: " + w1.getNoVowelsWord()); System.out.println("Is word a substring of sentence? " + w1.isSubstring()); System.out.println(); Word w2 = new Word("Applepie", "Apple"); System.out.println("New Word object"); System.out.println("Superclass word: " + w2.getSentence()); System.out.println("Subclass word: " + w2.getNoVowelsWord()); System.out.println("Is word a substring of sentence? " + w2.isSubstring()); System.out.println(); System.out.println("w1 and w2 are the same? " + w1.equals(w2)); */ } }
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 2PE
Related questions
Concept explainers
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Question
public class TestWord
{
public static void main(String[] args)
{
/*
Word w1 = new Word("Go Cubs Go", "Cub");
System.out.println("New Word object");
System.out.println("Superclass word: " + w1.getSentence());
System.out.println("Subclass word: " + w1.getNoVowelsWord());
System.out.println("Is word a substring of sentence? " + w1.isSubstring());
System.out.println();
Word w2 = new Word("Applepie", "Apple");
System.out.println("New Word object");
System.out.println("Superclass word: " + w2.getSentence());
System.out.println("Subclass word: " + w2.getNoVowelsWord());
System.out.println("Is word a substring of sentence? " + w2.isSubstring());
System.out.println();
System.out.println("w1 and w2 are the same? " + w1.equals(w2));
*/
}
}
![Test Word.java
public static void main(String[] args)
{
Word w1 = new Word ("Go Cubs Go", "Cub");
System.out.println("New Word object");
System.out.println("Superclass
System.out.println("Subclass
System.out.println("Is word a substring of sentence?" + w1.isSubstring());
System.out.println();
}
word: + w1.getSentence());
word: + w1.getNoVowelsword());
Word w2 = new Word ("Applepie", "Apple");
System.out.println("New Word object");
System.out.println("Superclass word: + w2.getSentence());
System.out.println("Subclass
word: + w2.getNoVowelsword());
System.out.println("Is word a substring of sentence?"+w2.isSubstring());
System.out.println();
System.out.println("w1 and w2 are the same?"+w1.equals(w2));
Sample run:
New Word object
Superclass word: Go Cubs Go
Subclass word: Cb
Is word a substring of sentence? false
New Word object
Superclass word: Applepie
Subclass word: ppl
Is word a substring of sentence? true
w1 and w2 are the same? false](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7cde3d06-db35-40f7-86ff-b51b5431a565%2F539876a9-6442-4092-a24e-6477c60b61d8%2Fie7hjo_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Test Word.java
public static void main(String[] args)
{
Word w1 = new Word ("Go Cubs Go", "Cub");
System.out.println("New Word object");
System.out.println("Superclass
System.out.println("Subclass
System.out.println("Is word a substring of sentence?" + w1.isSubstring());
System.out.println();
}
word: + w1.getSentence());
word: + w1.getNoVowelsword());
Word w2 = new Word ("Applepie", "Apple");
System.out.println("New Word object");
System.out.println("Superclass word: + w2.getSentence());
System.out.println("Subclass
word: + w2.getNoVowelsword());
System.out.println("Is word a substring of sentence?"+w2.isSubstring());
System.out.println();
System.out.println("w1 and w2 are the same?"+w1.equals(w2));
Sample run:
New Word object
Superclass word: Go Cubs Go
Subclass word: Cb
Is word a substring of sentence? false
New Word object
Superclass word: Applepie
Subclass word: ppl
Is word a substring of sentence? true
w1 and w2 are the same? false
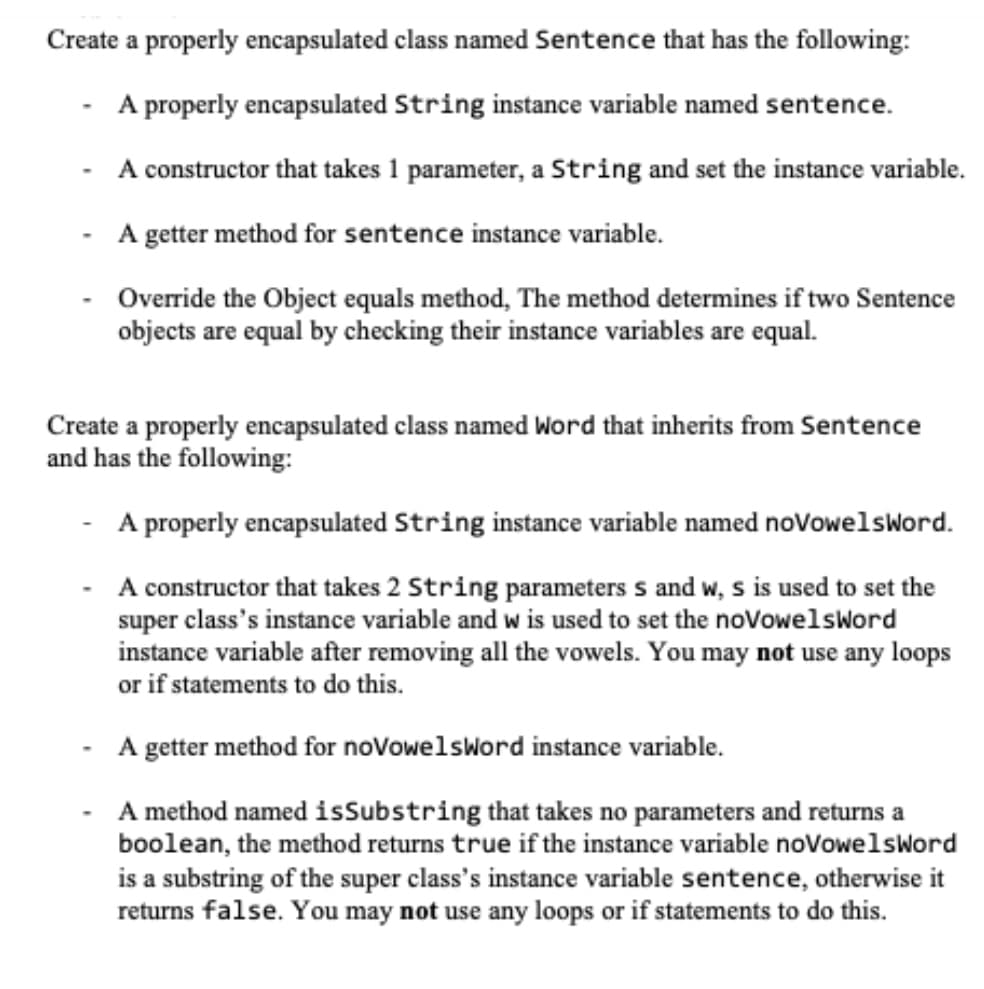
Transcribed Image Text:Create a properly encapsulated class named Sentence that has the following:
A properly encapsulated String instance variable named sentence.
A constructor that takes 1 parameter, a String and set the instance variable.
A getter method for sentence instance variable.
Override the Object equals method, The method determines if two Sentence
objects are equal by checking their instance variables are equal.
-
Create a properly encapsulated class named Word that inherits from Sentence
and has the following:
A properly encapsulated String instance variable named novowelsword.
A constructor that takes 2 String parameters s and w, s is used to set the
super class's instance variable and w is used to set the novowelsWord
instance variable after removing all the vowels. You may not use any loops
or if statements to do this.
A getter method for noVowelsword instance variable.
A method named isSubstring that takes no parameters and returns a
boolean, the method returns true if the instance variable noVowelsword
is a substring of the super class's instance variable sentence, otherwise it
returns false. You may not use any loops or if statements to do this.
Expert Solution

Step 1
Answer:
We have done code in java programming language and we have also attached code and code screenshot as well as output so we will see in the more details explanation.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
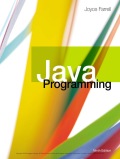
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
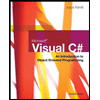
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
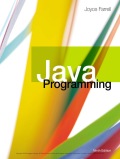
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
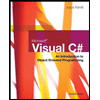
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,