Python: A group of statisticians at a local college has asked you to create a set of functions that compute the median and mode of a set of numbers. Define these functions, median and mode, in a module named stats.py. Also include a function named mean, which computes the average of a set of numbers. Each function should expect a list of numbers as an argument and return a single number. Each function should return 0 if the list is empty. Include a main function that tests the three statistical functions using the following list defined in main: lyst = [3, 1, 7, 1, 4, 10] An example of the program output is shown below: List: [3, 1, 7, 1, 4, 10] Mode: 1 Median: 3.5 Mean: 4.33333333333333 This is the code they gave me, but something is wrong or something is missing. from statistics import median def mode(lyst): fv = {} for v in lyst: fv[v] = fv.get(v, 0) + 1 mf = max(fv.values()) md = [key for key, v in fv.items() if v == mf] return md[0] lyst = [3,1,7,1,4,10] print ("List:",lyst) print("Mode: ",mode(lyst)) total = 0 Median = median(lyst) print("Median: ",Median) for i in lyst: total = total +i mean = total/len(lyst) print("Mean: ",mean)
Python:
A group of statisticians at a local college has asked you to create a set of functions that compute the median and mode of a set of numbers. Define these functions, median and mode, in a module named stats.py. Also include a function named mean, which computes the average of a set of numbers. Each function should expect a list of numbers as an argument and return a single number. Each function should return 0 if the list is empty. Include a main function that tests the three statistical functions using the following list defined in main:
lyst = [3, 1, 7, 1, 4, 10]
An example of the program output is shown below:
List: [3, 1, 7, 1, 4, 10]
Mode: 1
Median: 3.5
Mean: 4.33333333333333
This is the code they gave me, but something is wrong or something is missing.
from statistics import median
![Tasks
6.67
out of
10.00
Program produces correct output given input
Checks
2 out of 3 checks passed. Review the results below for more details.
Custom Test Complete
mode method test
Custom Test • Complete
median method test
Custom Test Incomplete
mean method test
Test Output
List: [3, 1, 7, 1, 4, 10]
Mode: 1
Median: 3.5
Mean: 4.333333333333333
Traceback (most recent call last):
File "/root/sandboxlaf25af9/nt-test-670e0c7f", line 3, in <module>
assert (stats.mean([3, 1, 7, 1, 4, 10]) == 4.333333333333333)
TypeError: 'float' object is not callable
Test Contents
import stats
assert (stats.mean([3, 1, 7, 1, 4, 10]) == 4.333333333333333)
assert (stats.mean([1, 1, 2, 3, 5, 8, 13, 21]) == 6.75)
assert (stats.mean([10, 13, 78, 69, 45, 23, 32, 23]) == 36.625)
>
>
stats.py
+
1 from statistics import median
2
3 def mode(lyst):
4
fv = {}
5
6
7
8
9
10
11
12
for v in lyst:
fv[v] = fv.get(v, 0) + 1
mf = max(fv.values())
md= [key for key, v in fv.items() if v == mf]
return md[0]
13
14
15
16
17
18
19 lyst [3,1,7,1,4,10]
20
21
22 print ("List: ",lyst)
23 print("Mode: ",mode (lyst))
24
25 total = 0
26
27 Median = median(lyst)
28
=
29 print("Median: ",Median)
30
31 for i in lyst:
32
33
total total +1
34
35 mean = total/len(lyst)
36
37 print("Mean: ", mean)
38
> Terminal
+
List: [3, 1, 7, 1, 4, 10]
Mode: 1
Median: 3.5
Mean: 4.333333333333333
38](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F2b5ce93e-94f9-49fd-8fc5-aabefed0184c%2F2595010e-e849-4f6d-853e-babb81537d84%2Fhzjuzr_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

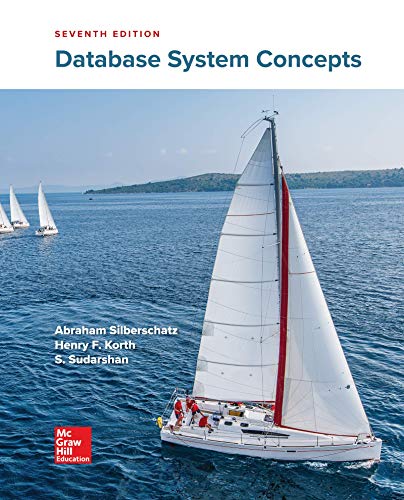
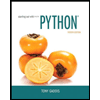
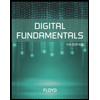
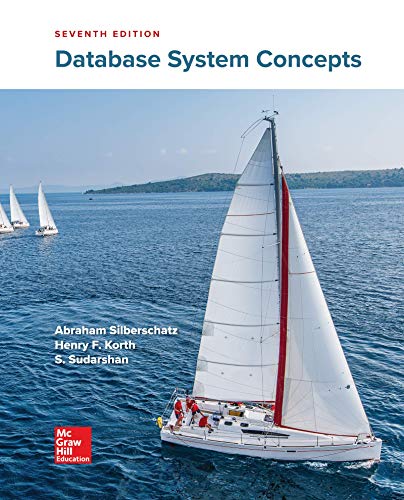
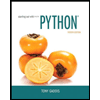
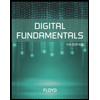
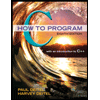
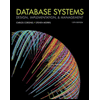
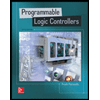