Python Activity (see pic about class Vector3D): Code for copy: class Vector3D: def __init__(self, x, y, z): # def __add__(self, other): # return None def __neg__(self): # return None def __sub__(self, other): # return None def __mul__(self, other): # return None def main(): testcases = int(input()) for t in range(testcases): line_in = input().split() op = line_in[0].strip() vec_vals = [int(x) for x in line_in[1:]] # TODO: a Write routine that processes a line in the input # op - string # - operation to do with the provided vectors # - can only be one from the set: {add, sub, neg, mul_s, mul} # vec_vals - list of integers # - numbers that follow the op in the input line # - can only have a length of 3, 4, or 6 if __name__ == '__main__': main()
Python Activity (see pic about class Vector3D):
Code for copy:
class Vector3D:
def __init__(self, x, y, z):
#
def __add__(self, other):
#
return None
def __neg__(self):
#
return None
def __sub__(self, other):
#
return None
def __mul__(self, other):
#
return None
def main():
testcases = int(input())
for t in range(testcases):
line_in = input().split()
op = line_in[0].strip()
vec_vals = [int(x) for x in line_in[1:]]
# TODO: a Write routine that processes a line in the input
# op - string
# - operation to do with the provided
# - can only be one from the set: {add, sub, neg, mul_s, mul}
# vec_vals - list of integers
# - numbers that follow the op in the input line
# - can only have a length of 3, 4, or 6
if __name__ == '__main__':
main()
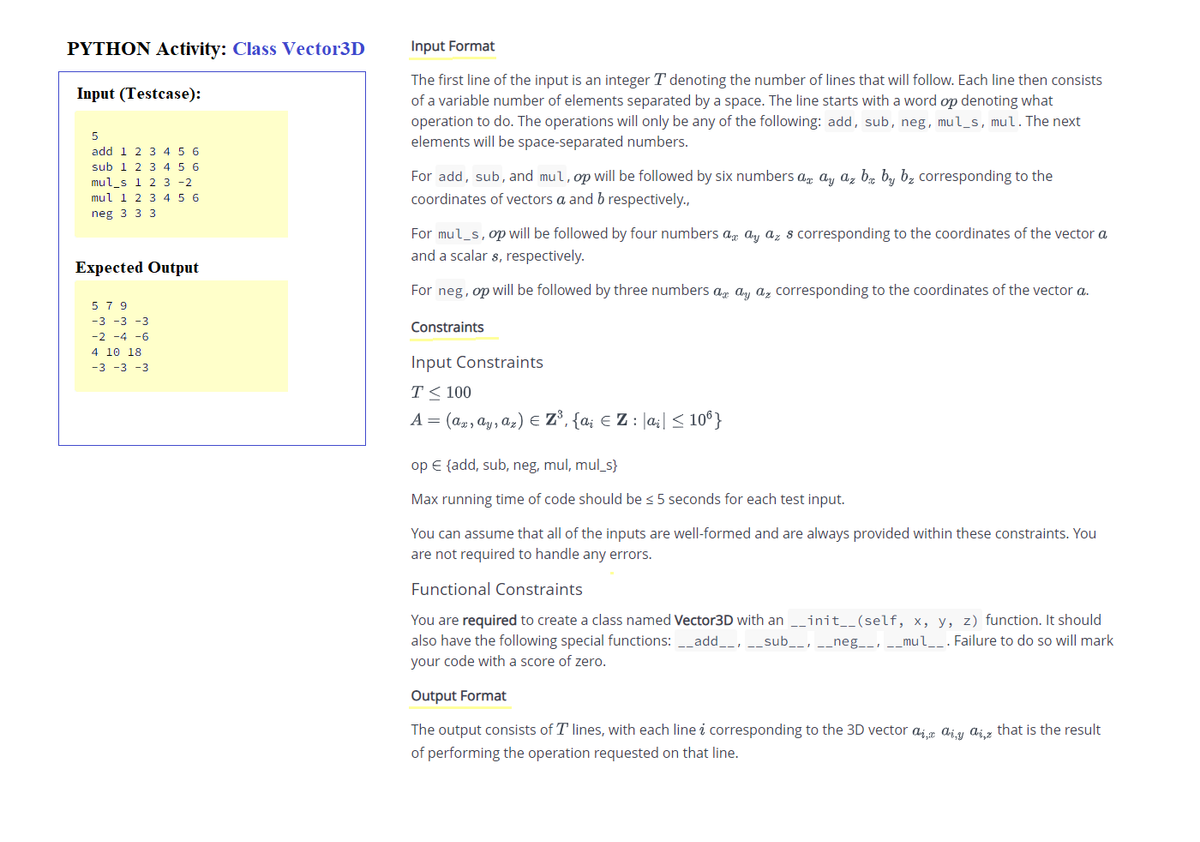

Step by step
Solved in 2 steps with 1 images

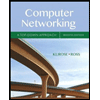
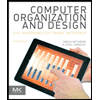
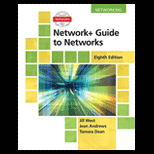
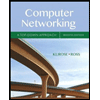
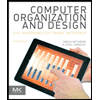
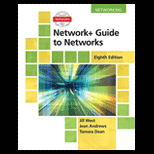
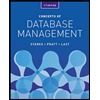
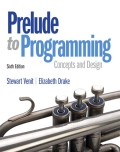
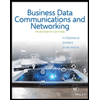