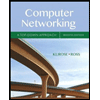
Using Java:
1 Description of the Program In this assignment, you are asked to implement a graphical application that displays a list of courses. Briefly, this GUI will take the inputs (i.e., course information), store them in an array list, and display course information in the output area. Figure 1 shows the layout of the CourseDisplay GUI. Figure 1: A screenshot of the CourseDisplay GUI. 2 Details of the program You should have three Java files: 1. CourseDisplayFrame class that extends JFrame class (The main part of your program). 2. CourseDisplayViewer class, which contains the main method where a CourseDisplay object will be created, and the GUI will pop up (Suggested frame width of 450, and frame height of 400). 3. Course class, which is the class that models the Course objects (This file will be given to you). 1 In your CourseDisplayFrame class file, you should have the followings: • Input area: – A text label and a text field (suggested width of 30) for course code; – A text label and a text field (suggested width of 30) for course name; – A text label and a text field (suggested width of 30) for course credit; – A text label and a text field (suggested width of 30) for course grade; • Buttons: – One button (AddCourse) used for adding a course into your course list, and append this course in the output area; – One button (SortByCode) used for sorting the course list by course code. The so the output should be displayed as in Figure 2. Figure 2: A screenshot of the sorted Courses by course code. – One button (SortByName) used for sorting the course list by course name. The output should be displayed as in Figure 3. – One button (SortByGrade) used for sorting the course list by course grade. The output should be displayed as in Figure 4. – One button (ResetInput) used for resetting all input fields; • Output area: – One text area (suggested row of 10 and column of 35) that displays course information; You may initialize the text area by including the headings (Code, Name, Credit, and Grade)
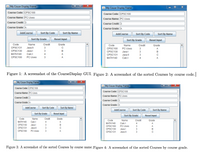

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

- The functionality of each file is described below:Class BankAccount This class has two instance variables:namebalanceCreate a constructor that takes two parameters to initialize the instance variables name and balance. The name must not be an empty string. The name also must not include any numbers or symbols. The balance must be zero or more to be used.If the name was an empty string a Value Error will be raised with the message “name cannot be empty”If the name contained numbers or symbols a Value Error will be raised with the message “name cannot include numbers or symbols”If the name was valid, it will be formatted properly and assigned to the instance variable. The valid name consists of alphabetical characters lowercase or upper case, may include space, underscore or hyphen.The name may consist of first, middle and last name.One way to validate the name is using Regular Expressions.Proper name format is the first character uppercase and the rest of the name lowercase character.…arrow_forwardDefine a class named BoatRace that contains the following information about a Boat Race: race_name: string race_id: int distance: int racers: List of Boat objects Write a constructor that allows the programmer to create an object of type BoatRace with a race_name, race_id, list of racers objects, and distance. The constructor will only take in one parameter, a string representing the name of a CSV file. The file will have the following format: Each row will always have exactly two columns. The first row will always contain the name of the race. The second row will always contain the id number for the race. The third row will always contain the distance for the race. All remaining rows contain information about the boats involved in the race: the first column will be the name of the boat, and the second column is that boat’s top speed. For example, the race in the file below has two boats: The Fire Ball with top speed 12, and The Leaf with top speed 100. Name,The Big One…arrow_forwardHappyTunes is an application for downloading music files. Each time a file is purchased, a transaction record is created that includes the music genre and price paid. The available genres are Classical, Easy Listening, Jazz, Pop, Rock, and Other. Develop an application that accepts input data for each transaction and displays a report that lists each of the music genres, along with a count of the number of downloads in each of the following price categories: Over $10.00 $6.00 through $9.99 $3.00 through $5.99 Under $3.00arrow_forward
- Design an application that declares an array of 20 AutomobileLoan objects. Prompt the user for data for each object, and then display all the values.arrow_forwardThe file Names.txt is located in the folder input_files within your project. The content of that file is shown in the following figure Java: import java.io.FileInputStream;import java.io.FileNotFoundException;import java.util.ArrayList;import java.util.Scanner;public class Question2 {public static void main(String[] args) throws FileNotFoundException {/*** Part a* Finish creating an ArrayList called NameList that stores the names in the file Names.txt.*/ArrayList<String> NameList;/*** Part b* Replace null on the right-hand-side of the declaration of the FileInputStream object named inputStream* so that it is initialized correctly to the Names.txt file located in the folder specified in the question description*/FileInputStream inputStream = null;Scanner scnr = new Scanner(inputStream); //Do not modify this line of code/*** Part c* Using a loop and the Scanner object provided, read the names from Names.txt* and store them in NameList created in Part a.*//*** Part d* Reorder the…arrow_forwardPython Required information id fname lname company address city ST zip 103 Art Venere 8 W Cerritos Ave #54 Bridgeport NJ 8014 104 Lenna Paprocki Feltz Printing 639 Main St Anchorage AK 99501arrow_forward
- PLEASE COMMENT CODE In a python program, create a new file and call it “ tracking”. Write to it four lines each contains information about an order like this: 1-00654-021 Dell charger Toronto-WEST 99-49-ZAD011-76540-022 ASUS battery Milton-EAST 34-56-CBH561-09239-026 HP HD Scarborough-NORTH 12-98-AZC451-12349-029 Mac FD North York-LAWRENCE 34-49-ZWL01Add the file two more lines: 1-34567-055 Lenovo SSD Milton-ON 34-09-MT04 1-90432-091 Lenovo battery Oakville-ON 78-KL98 Define a function that searches for a brand (e.g. Dell, ASUS, etc.). Test the function in your program.arrow_forwardAssignment Submission Instructions:This is an individual assignment – no group submissions are allowed. Submit a script file that contains the SELECT statements by assigned date. The outline of the script file lists as follows:/* ******************************************************************************** * Name: YourNameGoesHere * * Class: CST 235 * * Section: * * Date: * * I have not received or given help on this assignment: YourName * ***********************************************************************************/USE RetailDB;####### Tasks: Write SQL Queries ######### -- Task 1 (Customer Information):-- List your SELECT statement below. Make sure the SQL script file can be run successfully in MySQL and show the outcome of the code on MySQLarrow_forwardcreateDatabaseOfProfiles(String filename) This method creates and populates the database array with the profiles from the input file (profile.txt) filename parameter. Each profile includes a persons' name and two DNA sequences. 1. Reads the number of profiles from the input file AND create the database array to hold that number profiles. 2. Reads the profiles from the input file. 3. For each person in the file 1. creates a Profile object with the information from file (see input file format below). 2. insert the newly created profile into the next position in the database array (instance variable).arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
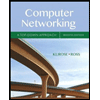
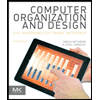
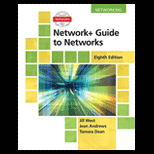
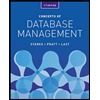
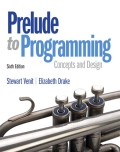
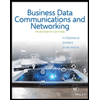