PYTHON LAB: Inserting an integer in descending order (doubly-linked list) Given main.py and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insert_in_descending_order() method to insert new IntNodes into the IntList in descending order. Ex. If the input is: 3 4 2 5 1 6 7 9 8 the output is: 9 8 7 6 5 4 3 2 1 _____________________________________________________________________________________ Main.py: from IntNode import IntNode from IntList import IntList if __name__ == "__main__": int_list = IntList() input_line = input() input_strings = input_line.split(' ') for num_string in input_strings: # Convert from string to integer num = int(num_string) # Insert into linked list in descending order new_node = IntNode(num) int_list.insert_in_descending_order(new_node) int_list.print_int_list() IntNode.py class IntNode: def __init__(self, initial_data, next = None, prev = None): self.data = initial_data self.next = next self.prev = prev IntList.py class IntList: def __init__(self): self.head = None self.tail = None def append(self, new_node): if self.head == None: self.head = new_node self.tail = new_node else: self.tail.next = new_node new_node.prev = self.tail self.tail = new_node def prepend(self, new_node): if self.head == None: self.head = new_node self.tail = new_node else: new_node.next = self.head self.head.prev = new_node self.head = new_node def insert_after(self, current_node, new_node): if self.head is None: self.head = new_node self.tail = new_node elif current_node is self.tail: self.tail.next = new_node new_node.prev = self.tail self.tail = new_node else: successor_node = current_node.next new_node.next = successor_node new_node.prev = current_node current_node.next = new_node successor_node.prev = new_node def remove(self, current_node): successor_node = current_node.next predecessor_node = current_node.prev if successor_node is not None: successor_node.prev = predecessor_node if predecessor_node is not None: predecessor_node.next = successor_node if current_node is self.head: self.head = successor_node if current_node is self.tail: self.tail = predecessor_node def print_int_list(self): current_node = self.head while current_node != None: print(current_node.data, end=" ") current_node = current_node.next def insert_in_descending_order(self, new_node): # TODO: Write insert_in_descending_order method
PYTHON LAB: Inserting an integer in descending order (doubly-linked list)
Given main.py and an IntNode class, complete the IntList class (a linked list of IntNodes) by writing the insert_in_descending_order() method to insert new IntNodes into the IntList in descending order.
Ex. If the input is:
3 4 2 5 1 6 7 9 8the output is:
9 8 7 6 5 4 3 2 1Main.py:
from IntNode import IntNode
from IntList import IntList
if __name__ == "__main__":
int_list = IntList()
input_line = input()
input_strings = input_line.split(' ')
for num_string in input_strings:
# Convert from string to integer
num = int(num_string)
# Insert into linked list in descending order
new_node = IntNode(num)
int_list.insert_in_descending_order(new_node)
int_list.print_int_list()
IntNode.py
class IntNode:
def __init__(self, initial_data, next = None, prev = None):
self.data = initial_data
self.next = next
self.prev = prev
IntList.py
class IntList:
def __init__(self):
self.head = None
self.tail = None
def append(self, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
else:
self.tail.next = new_node
new_node.prev = self.tail
self.tail = new_node
def prepend(self, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
else:
new_node.next = self.head
self.head.prev = new_node
self.head = new_node
def insert_after(self, current_node, new_node):
if self.head is None:
self.head = new_node
self.tail = new_node
elif current_node is self.tail:
self.tail.next = new_node
new_node.prev = self.tail
self.tail = new_node
else:
successor_node = current_node.next
new_node.next = successor_node
new_node.prev = current_node
current_node.next = new_node
successor_node.prev = new_node
def remove(self, current_node):
successor_node = current_node.next
predecessor_node = current_node.prev
if successor_node is not None:
successor_node.prev = predecessor_node
if predecessor_node is not None:
predecessor_node.next = successor_node
if current_node is self.head:
self.head = successor_node
if current_node is self.tail:
self.tail = predecessor_node
def print_int_list(self):
current_node = self.head
while current_node != None:
print(current_node.data, end=" ")
current_node = current_node.next
def insert_in_descending_order(self, new_node):
# TODO: Write insert_in_descending_order method

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

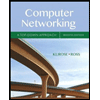
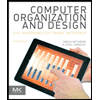
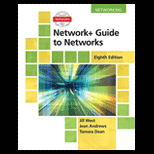
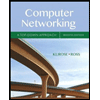
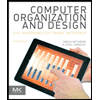
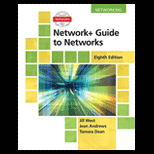
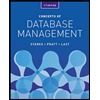
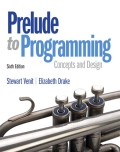
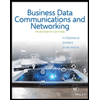