Could you help me the following question? The Node structure is defined as follows (we will use integers for data elements): public class Node { public int data; public Node next; public Node previous; } The Double Ended Doubly Linked List (DEDLL) class definition is as follows: public class DEDLL { public int currentSize; public Node head; public Node tail; } This design is a modification of the standard linked list. In the standard that we discussed in class, there is only a head node that represents the first node in the list and each node only contains a reference to the next node in the list until the chain of nodes reach null. In this DEDLL, each node also has a reference to the previous node. The DEDLL also contains a reference to the tail of the list that represents the last node in the list. For example, a DEDLL with 4 elements may have nodes with values 5, 2, 6, and 8 from head to tail. This means the head node points to 5 and the tail node points to 8. For node 5, its next pointer points to 2 and its previous pointer points to null. For node 2, its next is 6, and its previous is 5. Similarly for node 6, its next is 8 and its previous is 2. Finally, for node 8, its next points to null while its previous points to 6. Other observations with this structure: When the DEDLL is empty, both head and tail point to null When the DEDLL has only 1 node, the head and tail point to the same node Part 1) The challenge with this exercise is that with each of these functions, you must make sure each node’s next and previous pointers are correctly maintained and the head and tail pointers are referring to the correct ends of the list. Here are the functions to complete for the DEDLL: /* Basic functions */ public DEDLL() public void addFront(int n) public void addEnd(int n) public Node searchForward(int n) public Node searchReverse(int n) public boolean updateForward(int o, int n) public boolean updateReverse(int o, int n) public boolean deleteForward(int n) public boolean deleteReverse(int n) public void printSize() public void printForward() public void printReverse() /* Extended functions */ public void removeDuplicates() public void deleteAll() Basic print functions: Size: displays the number of elements in the DEDLL Forward: displays all Node data values starting from head and traversing each element in “next” order to tail. If the list is empty, it prints “Empty List” Reverse: displays all Node data values starting from tail and traversing each element in “previous” order to head. If the list is empty, it prints “Empty List” Extended functions: removeDuplicates() Go through the DEDLL and remove nodes that have duplicate values as other nodes. For example, if the list contains node values of 4 – 5 – 6 – 5 – 4 – 1 – 6 - 6 – 3 – 4, removeDuplicates will change the list to 4 – 5 – 6 – 1 – 3 Part 2) Write a main test program that adequately tests all these functions. The test program can be hard coded or it can be interactive to allow the user to perform any of these functions at any time. When developing and testing this project, make function calls that cover as many situations as you can see and manage. This means calling all of your functions at various times repeatedly to ensure they in different situations. Remember also that there are different scenarios to test such as: Insert, delete, etc., on empty lists Insert, delete, etc., on lists with only 1 Node Insert, delete, etc., on lists with more than 1 Node There are a lot of tests to perform. Your grade will be based not only on developing accurate function code, but also how detailed your test cases are to ensure you’ve done them properly.
Could you help me the following question?
The Node structure is defined as follows (we will use integers for data elements):
public class Node
{
public int data;
public Node next;
public Node previous;
}
The Double Ended Doubly Linked List (DEDLL) class definition is as follows:
public class DEDLL
{
public int currentSize;
public Node head;
public Node tail;
}
This design is a modification of the standard linked list. In the standard that we discussed in class, there is only a head node that represents the first node in the list and each node only contains a reference to the next node in the list until the chain of nodes reach null. In this DEDLL, each node also has a reference to the previous node. The DEDLL also contains a reference to the tail of the list that represents the last node in the list.
For example, a DEDLL with 4 elements may have nodes with values 5, 2, 6, and 8 from head to tail. This means the head node points to 5 and the tail node points to 8. For node 5, its next pointer points to 2 and its previous pointer points to null. For node 2, its next is 6, and its previous is 5. Similarly for node 6, its next is 8 and its previous is 2. Finally, for node 8, its next points to null while its previous points to 6.
Other observations with this structure:
- When the DEDLL is empty, both head and tail point to null
- When the DEDLL has only 1 node, the head and tail point to the same node
Part 1) The challenge with this exercise is that with each of these functions, you must make sure each node’s next and previous pointers are correctly maintained and the head and tail pointers are referring to the correct ends of the list. Here are the functions to complete for the DEDLL:
/* Basic functions */
public DEDLL()
public void addFront(int n)
public void addEnd(int n)
public Node searchForward(int n)
public Node searchReverse(int n)
public boolean updateForward(int o, int n)
public boolean updateReverse(int o, int n)
public boolean deleteForward(int n)
public boolean deleteReverse(int n)
public void printSize()
public void printForward()
public void printReverse()
/* Extended functions */
public void removeDuplicates()
public void deleteAll()
Basic print functions:
- Size: displays the number of elements in the DEDLL
- Forward: displays all Node data values starting from head and traversing each element in “next” order to tail. If the list is empty, it prints “Empty List”
- Reverse: displays all Node data values starting from tail and traversing each element in “previous” order to head. If the list is empty, it prints “Empty List”
Extended functions:
removeDuplicates()
- Go through the DEDLL and remove nodes that have duplicate values as other nodes. For example, if the list contains node values of 4 – 5 – 6 – 5 – 4 – 1 – 6 - 6 – 3 – 4, removeDuplicates will change the list to 4 – 5 – 6 – 1 – 3
Part 2) Write a main test program that adequately tests all these functions. The test program can be hard coded or it can be interactive to allow the user to perform any of these functions at any time. When developing and testing this project, make function calls that cover as many situations as you can see and manage. This means calling all of your functions at various times repeatedly to ensure they in different situations. Remember also that there are different scenarios to test such as:
- Insert, delete, etc., on empty lists
- Insert, delete, etc., on lists with only 1 Node
- Insert, delete, etc., on lists with more than 1 Node
There are a lot of tests to perform. Your grade will be based not only on developing accurate function code, but also how detailed your test cases are to ensure you’ve done them properly.
Program notes:
- Use the java programming
- For search, update, and delete when there are duplicate data values, the code should act on the first value encountered. For example, if your DEDLL from head to tail was 7 – 5 – 6 – 5 – 6 – 3 – 4 – 2, where head is 7 and tail is 2, “searchForward(6)” would return the 3rd node while “searchReverse(6) would return the 5th

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

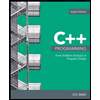
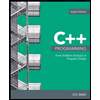