Python question Question 18 (Basic problem) A list of tuples named cereal_data contains calorie and price details of different cereals. Each tuple contains three elements. The first element of each tuple is the cereal name, stored as a string. The second element is the calorie content and the third element is the price per 100g. The calorie content and the prices are stored as floats. Write a function chosen_cereals(cereal_data,dollar_amount,cal_amount), which returns a list of cereal names which costs less than the dollar_amount and contains less calories than cal_amount. Your function should return None if no records are available that meet the given criteria. See the examples given below (doctests). Note: Your function should work with any database given. def chosen_cereals(cereal_data,dollar_amount,cal_amount): """ Input: A list of tuples with the cereal name, calorie content and the price per 100g. Output: A list of cereal names that costs less than the dollar_amount and contains less calories than cal_amount. Examples: >>> database = [('cherion',110,1.25),('milco',156,2.80),('wheatabits',92,0.85),\ ('cocopix',205,3.60),('corn fun',123,2.30),('yumbix',167,3.15)] >>> print(chosen_cereals(database,2.00,100)) ['wheatabits'] >>> print(chosen_cereals(database,3.20,150)) ['cherion', 'wheatabits', 'corn fun'] >>> print(chosen_cereals(database,1.50,75)) None >>> print(chosen_cereals(database,0.75,150)) None """
Python question
Question 18 (Basic problem)
A list of tuples named cereal_data contains calorie and price details of different cereals. Each tuple contains three elements. The first element of each tuple is the cereal name, stored as a string. The second element is the calorie content and the third element is the price per 100g. The calorie content and the prices are stored as floats.
Write a function chosen_cereals(cereal_data,dollar_amount,cal_amount), which returns a list of cereal names which costs less than the dollar_amount and contains less calories than cal_amount. Your function should return None if no records are available that meet the given criteria. See the examples given below (doctests).
Note: Your function should work with any
def chosen_cereals(cereal_data,dollar_amount,cal_amount):
"""
Input: A list of tuples with the cereal name, calorie content
and the price per 100g.
Output: A list of cereal names that costs less than the dollar_amount
and contains less calories than cal_amount.
Examples:
>>> database = [('cherion',110,1.25),('milco',156,2.80),('wheatabits',92,0.85),\
('cocopix',205,3.60),('corn fun',123,2.30),('yumbix',167,3.15)]
>>> print(chosen_cereals(database,2.00,100))
['wheatabits']
>>> print(chosen_cereals(database,3.20,150))
['cherion', 'wheatabits', 'corn fun']
>>> print(chosen_cereals(database,1.50,75))
None
>>> print(chosen_cereals(database,0.75,150))
None
"""

Step by step
Solved in 4 steps with 2 images

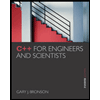
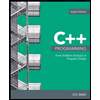
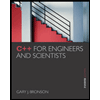
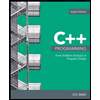