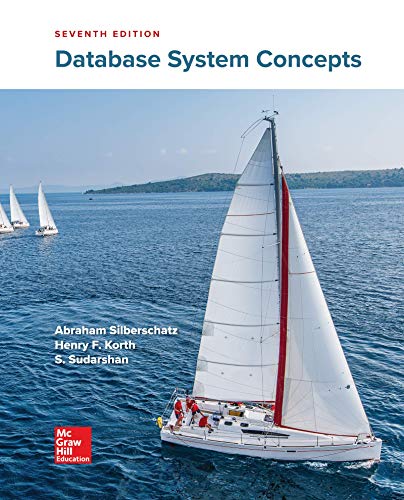
Concept explainers
Application: Apply
The following questions ask you to manually apply an algorithm introduced in the lecture and demonstrate your understanding by describing intermediate states of the computation.
Question 5 (Sorting)
Write down the list content at the end of each iteration of the main loop (ie. end of each pass) of Insertion Sort for the following list:
[14,12,7,4,18,9]
Use one line for each pass.
Question 6 (Graph Traversal)
Apply Depth First Search (DFS) to traverse the following graph. Start your traversal from vertex 0, and write down the order in which vertices will be visited during the traversal. (image for this question also attached)
Question 7 (Gaussian elimination) (image for this question also attached)
Apply forward elimination to this system of linear equations to put it into the upper triangular form.
⎛⎝⎜⎜1 1 2225−12−1⎞⎠⎟⎟⎛⎝⎜⎜? ? ?⎞⎠⎟⎟=⎛⎝⎜⎜6 3 13⎞⎠⎟⎟(12−1 122 25−1)(� � �)=(6 3 13)
Write each row operation you use to solve the problem.
Find the values of x, y, and z using back substitution.
Write the matrix and the right-hand side after each row operation, for example, the initial matrix and right-hand side can be represented as:
A = [ [1, 2, -1],
[1, 2, 2],
[2, 5, -1] ]
b = [6, 3,13]
Note: Do NOT write code. Show the values in A and b after each row operation and then find the values of x, y and z using back substitution.
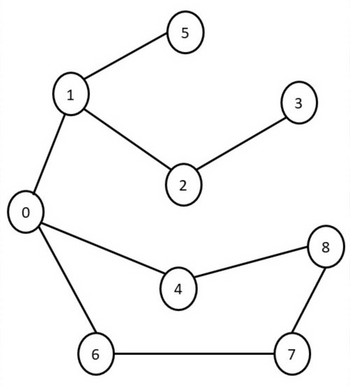
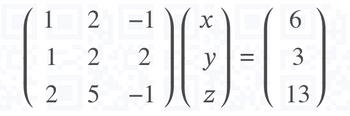

Step by stepSolved in 4 steps with 1 images

May I ask for the answer and explanation for question 7?
May I ask for the answer and explanation for question 7?
- Sort the following lists using the Bubble Sort algorithm provide Step-by-step answers? a) Original list: [5, 2, 8, 1,6] b) Original list: [8, 3, 1, 5, 4]arrow_forwardDescribe how to search a list using sequential search.arrow_forwardFor each of the following Python list methods indicate whether the method mutates the list. Write "yes" if the method mutates the list or "no" if the method does not mutate the list. append sort reverse index insert count remove poparrow_forward
- **Cenaage Python** Question: A sequential search of a sorted list can halt when the target is less than a given element in the list. Modify the program to stop when the target becomes less than the current value being compared. In a sorted list, this would indicate that the target is not in the list and searching the remaining values is unnecessary. CODE: def sequentialSearch(target, lyst): """Returns the position of the target item if found, or -1 otherwise. The lyst is assumed to be sorted in ascending order.""" position = 0 while position < len(lyst): if target == lyst[position]: return position position += 1 return -1 def main(): """Tests with three lists.""" print(sequentialSearch(3, [0, 1, 2, 3, 4])) print(sequentialSearch(3, [0, 1, 2])) # Should stop at second position. print(sequentialSearch(3, [0, 4, 5, 6])) if __name__ == "__main__": main()arrow_forwardPerform the following operations utilizing c++ code.N.b array size to be used is ten.a)enqueue() i.e enqueue the list; Two,three,four,five,six,seven and eight and after display enqued list.b)dequeue() i.e delete or remove any two elements in the queue in a) above and after display the updated queue after deletion.c)ISfull() i.e Display if the queue is Full or not.d)Isempty() i.e Display if the queue is Empty or not.e)print() i.e print the final list of the elements of the Queue after performing tasks of a) - d).arrow_forwardWrite in C Language. - sort "Algorithm" word by using insertion sort. (do in Simple code) - sort "Algortihm" word by using merge sort. (do in Intermediate code)arrow_forward
- Sort the list “A, L, G, O, R, I, T, H, M” in alphabetical order by Selection sort .arrow_forwardFill-In Blanks of the following question: At the end of every iteration of gets at the end of the list. Answer: sort the heaviest elementarrow_forwardO b. Remove the O. Remove the second element in the list O d. Remove the element before the last element in the list QUESTION 6 The following code inserts an element at the beginning of the list public void insertBegin(T e) { Node temp = new Node (e); head = temp; temp.Next = head; size++; } O True O False QUESTION 7 what does the following code do?arrow_forward
- using python in google colabarrow_forwardSort the following list using the Selection Sort algorithm . Show the list after each iteration of the outer for loop (after each complete pass through the list) IMPORTANT: Separate each value by a comma and only one space after each comma and no space after the last value. You will have seven iterations of the list for your answer. 38, 60, 43, 5, 70, 58, 15, 10arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
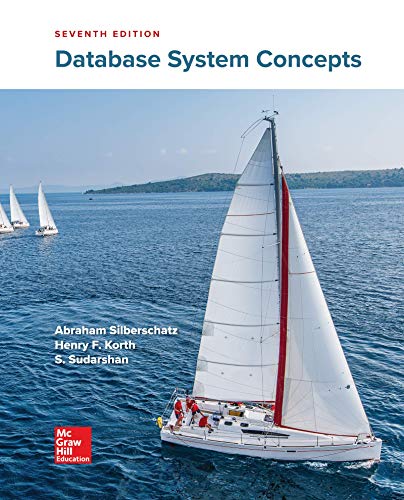
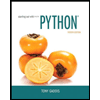
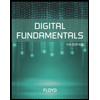
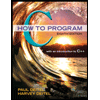
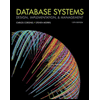
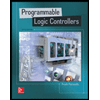