Q 4.1 I have provided the code I have written. All I need you to do is to rewrite the code. I need you to rewrite the comments, rename all the variables, and shift the code. It should look different once you have edited it. I would like to see another format for this code. It is in C-Programming. I think it shouldn’t take so long. Take your time, please! I really appreciate any help you can provide! #include #include #include // create a struct to represent the node in linked list typedef struct node { int data; struct node *next; }Node; // function declaration Node* insert(Node *head, int n); Node* delete(Node* head, int n); int length(Node* head); void print(Node *head); int contains(Node *head, int n); void deleteList(Node *head); int main(void) { Node *head = NULL; // create an empty linked list pointed by head int data; char command; // loop that continues till user doesn't type an unknown command do { fflush(stdout); // read the command and the data scanf(" %c %d",&command,&data); // check if command is not valid, then exit from loop if((tolower(command) != 'i') && (tolower(command) != 'd')) break; // insert data in linked list if(tolower(command) == 'i') head = insert(head,data); else // delete data from linked list head = delete(head,data); // print the length of linked list printf("%d : ",length(head)); print(head); // print the linked list printf("\n"); }while((tolower(command) == 'i') || (tolower(command) == 'd')); deleteList(head); // delete the nodes of linked list return EXIT_SUCCESS; } // function to insert n in sorted manner and return the updated head Node* insert(Node *head, int n) { if(contains(head,n) == 1) // check if n is present in linked list, then do nothing return head; Node *node = (Node*)malloc(sizeof(Node)); // create a new node // check if memory allocation is successful if(node != NULL) { // set data and next pointer of node node->data = n; node->next = NULL; // if empty list, set head = node if(head == NULL) head = node; else { Node *curr = head; Node *prev = NULL; // loop to insert n in correct position while(curr != NULL) { // if curr's data > n,insert it before curr if(curr->data > node->data) { if(prev == NULL) { node->next = head; head = node; }else { node->next = curr; prev->next = node; } return head; } prev = curr; curr = curr->next; } prev->next = node; // insert n at end } } return head; } // function to delete n from linked list and return the updated head Node* delete(Node* head, int n) { // if list is not empty if(head != NULL) { // if data at head = n, set head to node next to head if(head->data == n) { Node *temp = head; head = head->next; free(temp); // delete old head }else { Node *curr = head; Node *prev = NULL; // loop to get the node corresponding to n and delete it while(curr != NULL) { if(curr->data == n) { prev->next = curr->next; free(curr); return head; } prev = curr; curr = curr->next; } } } return head; } // function to count and return the number of nodes present in the linked list int length(Node* head) { int count = 0; Node *curr = head; // loop over the list, counting the nodes while(curr != NULL) { count++; curr = curr->next; } return count; } // function to print the contents of the linked list void print(Node *head) { Node *curr = head; // loop over the list , printing the data while(curr != NULL) { printf("%d ",curr->data); curr = curr->next; } } // function to return 1, if n is present in linked list else 0 int contains(Node *head, int n) { Node *curr = head; // loop over list, searching for n while(curr != NULL) { if(curr->data == n) return 1; curr = curr->next; } return 0; } // function to delete the memory allocated to linked list void deleteList(Node *head) { Node *curr; while(head != NULL) { curr = head; head = head->next; free(curr);
Q 4.1
I have provided the code I have written. All I need you to do is to rewrite the code. I need you to rewrite the comments, rename all the variables, and shift the code. It should look different once you have edited it. I would like to see another format for this code. It is in C-Programming. I think it shouldn’t take so long. Take your time, please! I really appreciate any help you can provide!
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
// create a struct to represent the node in linked list
typedef struct node
{
int data;
struct node *next;
}Node;
// function declaration
Node* insert(Node *head, int n);
Node* delete(Node* head, int n);
int length(Node* head);
void print(Node *head);
int contains(Node *head, int n);
void deleteList(Node *head);
int main(void) {
Node *head = NULL; // create an empty linked list pointed by head
int data;
char command;
// loop that continues till user doesn't type an unknown command
do
{
fflush(stdout);
// read the command and the data
scanf(" %c %d",&command,&data);
// check if command is not valid, then exit from loop
if((tolower(command) != 'i') && (tolower(command) != 'd'))
break;
// insert data in linked list
if(tolower(command) == 'i')
head = insert(head,data);
else // delete data from linked list
head = delete(head,data);
// print the length of linked list
printf("%d : ",length(head));
print(head); // print the linked list
printf("\n");
}while((tolower(command) == 'i') || (tolower(command) == 'd'));
deleteList(head); // delete the nodes of linked list
return EXIT_SUCCESS;
}
// function to insert n in sorted manner and return the updated head
Node* insert(Node *head, int n)
{
if(contains(head,n) == 1) // check if n is present in linked list, then do nothing
return head;
Node *node = (Node*)malloc(sizeof(Node)); // create a new node
// check if memory allocation is successful
if(node != NULL)
{
// set data and next pointer of node
node->data = n;
node->next = NULL;
// if empty list, set head = node
if(head == NULL)
head = node;
else
{
Node *curr = head;
Node *prev = NULL;
// loop to insert n in correct position
while(curr != NULL)
{
// if curr's data > n,insert it before curr
if(curr->data > node->data)
{
if(prev == NULL)
{
node->next = head;
head = node;
}else
{
node->next = curr;
prev->next = node;
}
return head;
}
prev = curr;
curr = curr->next;
}
prev->next = node; // insert n at end
}
}
return head;
}
// function to delete n from linked list and return the updated head
Node* delete(Node* head, int n)
{
// if list is not empty
if(head != NULL)
{
// if data at head = n, set head to node next to head
if(head->data == n)
{
Node *temp = head;
head = head->next;
free(temp); // delete old head
}else
{
Node *curr = head;
Node *prev = NULL;
// loop to get the node corresponding to n and delete it
while(curr != NULL)
{
if(curr->data == n)
{
prev->next = curr->next;
free(curr);
return head;
}
prev = curr;
curr = curr->next;
}
}
}
return head;
}
// function to count and return the number of nodes present in the linked list
int length(Node* head)
{
int count = 0;
Node *curr = head;
// loop over the list, counting the nodes
while(curr != NULL)
{
count++;
curr = curr->next;
}
return count;
}
// function to print the contents of the linked list
void print(Node *head)
{
Node *curr = head;
// loop over the list , printing the data
while(curr != NULL)
{
printf("%d ",curr->data);
curr = curr->next;
}
}
// function to return 1, if n is present in linked list else 0
int contains(Node *head, int n)
{
Node *curr = head;
// loop over list, searching for n
while(curr != NULL)
{
if(curr->data == n)
return 1;
curr = curr->next;
}
return 0;
}
// function to delete the memory allocated to linked list
void deleteList(Node *head)
{
Node *curr;
while(head != NULL)
{
curr = head;
head = head->next;
free(curr);
}
}
//end of program

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

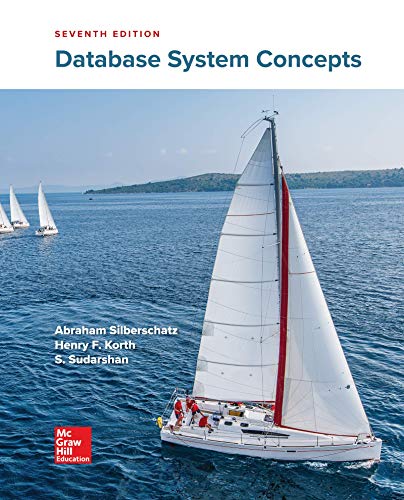
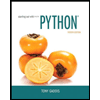
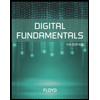
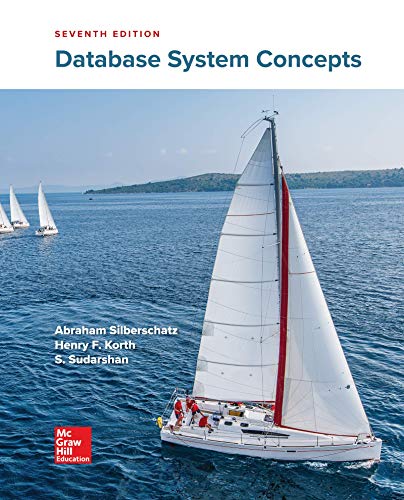
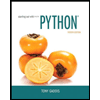
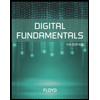
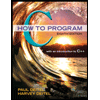
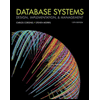
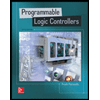