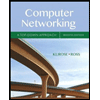
I've been asking about this poker game that I have been trying to compile in c++
Main.cpp
#include <iostream>
#include <string>
#include <fstream>
#include <iomanip>
#include <sstream>
#include "card.h"
#include "deck.h"
#include "hand.h"
using namespace std;
int main()
{
string repeat = "Y";
Deck myDeck;
Hand myHand;
string exchangeCards;
while (repeat == "Y" || repeat == "y")
{
cout << endl;
myHand.newHand(myDeck);
myHand.print();
cout << endl;
cout << "Would you like to exchange any cards? [Y / N]: ";
getline(cin, exchangeCards);
while (exchangeCards != "Y" && exchangeCards != "y" && exchangeCards != "X" && exchangeCards != "n")
{
cout << "Please enter Y or N only: ";
getline(cin, exchangeCards);
}
if(exchangeCards == "Y" || exchangeCards == "y")
{
myHand.exchangeCards(myDeck);
}
cout << endl;
myHand.print();
cout << endl;
myDeck.reset(); // Resets the deck for a new game
cout << "Play again? [Y / N]: ";
getline(cin, repeat);
while (repeat != "Y" && repeat != "y" && repeat != "N" && repeat != "n")
{
cout << "Please enter Y or N only: ";
getline(cin, repeat);
}
}
return 0;
}
Deck.h
#ifndef DECK_H
#define DECK_H
#include <vector>
#include <cstdlib> // srand(), rand()
#include <ctime> // time()
#include "card.h" // Include card header file here
using namespace std;
class Deck
{
public:
Deck();
void resetDeck();
void printUndealtDeck();
void printDealtDeck();
const int getSizeUndealtDeck();
const int getSizeDealtDeck();
Card dealCard(); // Is the dealCard() here an accessor or mutator function???
private:
vector<Card> m_undealtDeck; // Undealt cards
vector<Card> m_dealtDeck; // Dealt cards
};
#endif
hand.h:
#ifndef HAND_H
#define HAND_H
#include <iostream>
#include "card.h" // Include card header file here
#include "deck.h" // Include deck header file here
using namespace std;
const int NUM_CARDS_ON_HAND = 5;
class Hand
{
public:
void newCard(Deck& deck, int location);
void newHand(Deck& deck);
void exchangeCards(Deck& deck);
void print();
private:
Card m_hand[NUM_CARDS_ON_HAND]; // A hand consists of 5 cards
};
#endif
card.h:
#ifndef CARD_H
#define CARD_H
#include <iostream>
#include <string>
using namespace std;
const string pips[] = {"Ace", "Two", "Three", "Four", "Five",
"Six", "Seven", "Eight", "Nine", "Ten",
"Jack", "Queen", "King"};
const string suits[] = {"Hearts", "Spades", "Clubs", "Diamonds"};
class Card
{public:
int get();
void set(int value);
string getPip();
string getSuit();
void print();
private:
int m_cardValue;
};
#endif
Deck.cpp:
#include "deck.h"
Deck::Deck() //--- default class constructor
{ srand(time(0));
for (int i=0; i < 52; i++)
{Card cardInstance;
cardInstance.set(i)
m_undealtDeck.push_back(cardInstance); // should it be .get here()???
}
}
void Deck::reset()
{ while (getSizeDealtDeck() != 0)
{
int last_index = getSizeDealtDeck()-1;
m_undealtDeck.push_back(m_dealtDeck[last_index]);
m_dealtDeck.pop_back();
}
}
Card Deck::dealCard() // --- Deal a single card
{ int random_index = rand() % getSizeUndealtDeck(); // gets random index in undealt deck
Card random_card; // creates a card instance
random_card.set(m_undealtDeck[random_index].get()); // set the random card to the correct value
m_dealtDeck.push_back(random_card); // pushes the random card to the dealt deck
m_undealtDeck[random_index] = m_undealtDeck.back(); // puts the back index value to the random index
m_undealtDeck.pop_back(); // gets rid of the back value
return random_card;
const int Deck::getSizeUndealtDeck() // --- return size of undealt deck
{ return m_undealtDeck.size();
}
const int Deck::getSizeDealtDeck() // --- return size of dealt deck
{ return m_dealtDeck.size();
}
void Deck::printUndealtDeck() // loops through the undealt deck and prints it
{
for (int i=0; i < getSizeUndealtDeck(); i++)
{
m_undealtDeck[i].print();
cout << endl;
}
}
void Deck::printDealtDeck() // loops through the dealt deck
{
for (int i=0; i < getSizeDealtDeck(); i++)
{
m_dealtDeck[i].print();
cout << endl;
}
}
Hand.cpp
#include "pokerHand.h"
void Hand::newCard(Deck& deck, int location)
{
Card new_card = deck.dealCard();
m_hand[location] = new_card;
}
void Hand::newHand(Deck& deck)
{
for (int i=0; i < NUM_CARDS_ON_HAND; i++)
{
Hand::newCard(deck, i);
}
}
void Hand::exchangeCards(Deck& deck)
{
int num_of_exchanges;
cout << "How many cards would you like to exchange (1-5): "; // gets number of cards to be exchanged
cin >> num_of_exchanges;
while (num_of_exchanges > 5 || num_of_exchanges < 1) // validates input
{
cout << "Please enter a number (1-5): ";
cin >> num_of_exchanges;
}
for (int i=0; i < num_of_exchanges; i++) // looping through number of exchanges
{
int position = i; // positioned to be changed is i
if (num_of_exchanges != NUM_CARDS_ON_HAND) // if number of exchanges is less than total hand count
{
cout << "Enter a position in hand of card to exchange: ";
cin >> position; // gets position to be replaced
while (position > 5 || position < 1) // validates input
{
cout << "Please enter a number (1-5): ";
cin >> position;
}
position--;
}
Hand::newCard(deck, position); // replaces card at position
}
}
void Hand::print()
{for (int i=0; i < NUM_CARDS_ON_HAND; i++)
{cout << "Card " << i+1 << " is the ";
m_hand[i].print();
cout << endl;
}}
Card.cpp:
![H *card.cpp - Code:Blocks 20.03
File Edit View Search Project Build Debug Fortran wxSmith Tools Tools+ Plugins DoxyBlocks Settings Help
D E G: A C: II X
E C:
E Card:
get) : void
/** *<
+ + 2 I Aa *
Q Q S C
Start here x *deck.cpp x *hand.cpp x *main.cpp x *deck.h X *hand.h x *card.h x *card.cpp x
#include "card.h"
2
void Card: :get ()
3
return m cardValue;
5
6
void Card: :set (int newCardVal)
8
m cardvalue = newCardVal;
9
10
string Card:: getPip ()
11
12
return pips [m_cardValue $ 13];
13
14
string Card:: getSuit ()
15
16
return suits [m_cardValue / 13];
17
18
void Card: :print ()
19
20
int suit number = m cardValue / 13;
21
int pip number = m cardValue % 13;
22
cout << pips [pip number] << " of " << suits[suit number];
23
}
24
C\Users\Micai\Documents\Assignment2\card.cpp
C/C++
Windows (CR+LF) WINDOWS-1252 Line 5, Col 2, Pos 66
Insert
Modified
Read/Write
default](https://content.bartleby.com/qna-images/question/e6b9d87e-6e1d-4edc-b4b6-dbb55c2a248d/a554b8eb-4d89-408a-8db7-000ae83b991d/7yzkai_thumbnail.png)
![H *main.cpp - Code:Blocks 20.03
File Edit View Search Project Build
Debug Fortran wxSmith ITools Tools+ Plugins DoxyBlocks Settings Help
D E G: A C: II X
E C:
E <global>
v main0 : int
** *<
Q Q S C
Start here X *deck.cpp x *hand.cpp x *main.cpp x *deck.h x *hand.h X *card.h x *card.cpp x
32
while (exchangeCards != "y" && exchangeCards != "y" && exchangeCards != "X" && exchangeCards != "n")
33
{
34
cout << "Please enter Y or N only: ";
35
getline (cin, exchangeCards);
36
}
37
|| exchangeCards == "y")
38
{
39
myHand.exchangeCards (myDeck) ;
40
41
cout << endl;
42
myHand.print ();
43
cout << endl;
44 O
// Resets the deck for a new game
myDeck.reset ();
cout << "Play again? [Y / N]: ";
getline (cin, repeat);
45
46
47
while (repeat !- "Y" && repeat
!= "y" && repeat != "N" && repeat != "n")
48
{
49
cout << "Please enter Y or N only: ";
50
getline (cin, repeat);
Logs & others
1 2 Cccc X
Ở Build log X
Build messages X CppCheck/Vera++ X 2 CppCheck/Vera++ messages X Cscope X
Debugger X 2 DoxyBlocks x F Fortran info x
t Closed files list X
O Thread
File
Line
Message
=== Build file: "no target" in "no project" (compiler: unknown)
===
C:\Users\Mica...
In function 'int main ()':
C:\Users\Mica...
44
error: 'resetDeck' was not declared in this scope
=== Build failed: 1 error (s), 0 warning (s) (0 minute (s), 2 second (s))
===
%3%D3D3D
C:\Users\Micai\Documents\Assignment2\main.cpp
C/C++
Windows (CR+LF) WINDOWS-1252 Line 45, Col 25, Pos 1199
Insert
Modified
Read/Write
default](https://content.bartleby.com/qna-images/question/e6b9d87e-6e1d-4edc-b4b6-dbb55c2a248d/a554b8eb-4d89-408a-8db7-000ae83b991d/6u7hf9n_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- >> IN C PROGRAMMING LANGUAGE ONLY << COPY OF DEFAULT CODE, ADD SOLUTION INTO CODE IN C #include <stdio.h>#include <stdlib.h>#include <string.h> #include "GVDie.h" int RollSpecificNumber(GVDie die, int num, int goal) {/* Type your code here. */} int main() {GVDie die = InitGVDie(); // Create a GVDie variabledie = SetSeed(15, die); // Set the GVDie variable with seed value 15int num;int goal;int rolls; scanf("%d", &num);scanf("%d", &goal);rolls = RollSpecificNumber(die, num, goal); // Should return the number of rolls to reach total.printf("It took %d rolls to get a \"%d\" %d times.\n", rolls, num, goal); return 0;}arrow_forwardIn C++: Print a table that prompts the user to enter the current tuition and displays a table that displayshow many years the tuition will be doubled if the tuition is increased by 5%, 6%, and up to 10%annually. Here is a sample run: Enter the current year tuition: 20000 Rate Number of Years 5.0% 15 6.0% 12 7.0% 11 8.0% 10 9.0% 9 10.0% 8 1. Add comments throughout the program explaining your code.2. Use meaningful names for variable names.3. Submit program in .cpp filearrow_forwardin C++ , change the code to do the same purpose and same output : Source Code: #include<iostream>#include<fstream>#include<string> using namespace std; void displayPlain();void displayHex(); void executeCommand(int choice){ switch (choice) { case 1: displayPlain(); break; case 2: displayHex(); break; case 9: exit(0); default: cout<<"Invalid Choice Entered!!\n"; }} void displayMenu(){ cout<<"\n===============Menu==========================\n\n"; cout<<"1. Select 1 to see file data in plain text\n"; cout<<"2. Select 2 to see file data in hexadecimal\n"; cout<<"3. Select 9 to quit\n\n";} int main(){ int choice; do{ displayMenu(); cout<<"choice: "; cin>>choice; executeCommand(choice); }while(choice != 9); return 0;} void displayPlain(){ fstream file; file.open("q4File.txt", ios::in); string line;…arrow_forward
- I've been asking about this poker game that I have been trying to compile in c++. Main.cpp #include <iostream>#include <string>#include <fstream>#include <iomanip>#include <sstream>#include "card.h"#include "deck.h"#include "hand.h"using namespace std;int main(){string repeat = "Y";Deck myDeck;Hand myHand;string exchangeCards;while (repeat == "Y" || repeat == "y"){cout << endl;myHand.newHand(myDeck);myHand.print();cout << endl;cout << "Would you like to exchange any cards? [Y / N]: ";getline(cin, exchangeCards);while (exchangeCards != "Y" && exchangeCards != "y" && exchangeCards != "X" && exchangeCards != "n"){cout << "Please enter Y or N only: ";getline(cin, exchangeCards);}if(exchangeCards == "Y" || exchangeCards == "y"){myHand.exchangeCards(myDeck);}cout << endl;myHand.print();cout << endl;myDeck.reset(); // Resets the deck for a new gamecout << "Play again? [Y / N]: ";getline(cin,…arrow_forwardPlease help in C++ Use a counter controlled for looparrow_forwardHello, I was making a hangman simulation in C++. The code runs, but not fully. Could you identify the error and fix it? #include<iostream>#include<cstring> // for string class functions#include<fstream>#include <cctype>using namespace std; int main(){// define variable to get the response from user "Yes" or "No"string response;// Define index variableint w = 0;// define number of words that need to be guessed by the user assume 4const int WORDS = 4;// loopdo{// we will define the hangman bodyconst char body[] = "o/|\\|\\";// here we define the wordsstring words[WORDS] = {"MACAW", "SADDLE", "TOASTER", "XENOICIDE"};// fetch size or lengthstring xword(words[w].length(),'*');// define iterator to fetch the wordsstring::iterator i, ix = xword.begin();// define number of words to prompt for the userchar letters[26]={"\0"};// Now we define the variables which will be used in the simulationint n =0, xcount = xword.length();bool found = false, solved = false, hung =…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
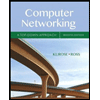
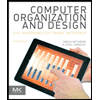
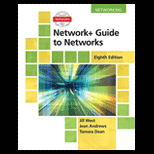
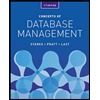
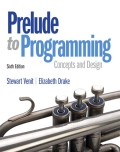
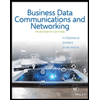