Q3: Continue of previous question (20pt) 1. Copy the previous program to a new file. 2. Convert Vehicle to abstract class. 3. Override output function of Car and Boat class. Use following main() to test your function. int main(){ Vehicle *a; a = new Car("Honda", 2000); a->output(); // Brand:Honda Weight: 2000 a = new Boat("Baja", 100); a->output(); // Brand:Baja hullLength: 100 } Output from main: Brand: Honda Weight: 2000 Brand: Baja hullLength: 100
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
my code is displayed below, just need help with this question in c++.
#include <iostream>
using namespace std;
class Vehicle{
private:
string *brand;
public:
Vehicle(){
brand = new string;
*brand = "TBD";
}
Vehicle(string b){
brand = new string;
*brand = b;
}
void output(){
cout << "Brand: " << *brand << endl;
}
void setBrand(string b){
*brand = b;
}
string getBrand(){return *brand;}
};
class Car:public Vehicle{
private:
int *weight;
public:
Car():Vehicle(){
weight = new int;
*weight = 0;
}
Car(string b, int w):Vehicle(b){
weight = new int;
*weight = w;
}
void output(){
cout << "Brand: " << getBrand() << " Weight: " << *weight << endl;
}
void setWeight(int w){
*weight = w;
}
int getWeight(){return *weight;}
};
class Boat:public Vehicle{
private:
int *hullLength;
public:
Boat():Vehicle(){
hullLength = new int;
*hullLength = 0;
}
Boat(string b, int h):Vehicle(b){
hullLength = new int;
*hullLength = h;
}
void output(){
cout << "Brand: " << getBrand() << " Hull Length: " << *hullLength << endl;
}
void sethullLength(int h){
*hullLength = h;
}
int gethullLength(){return *hullLength;}
};
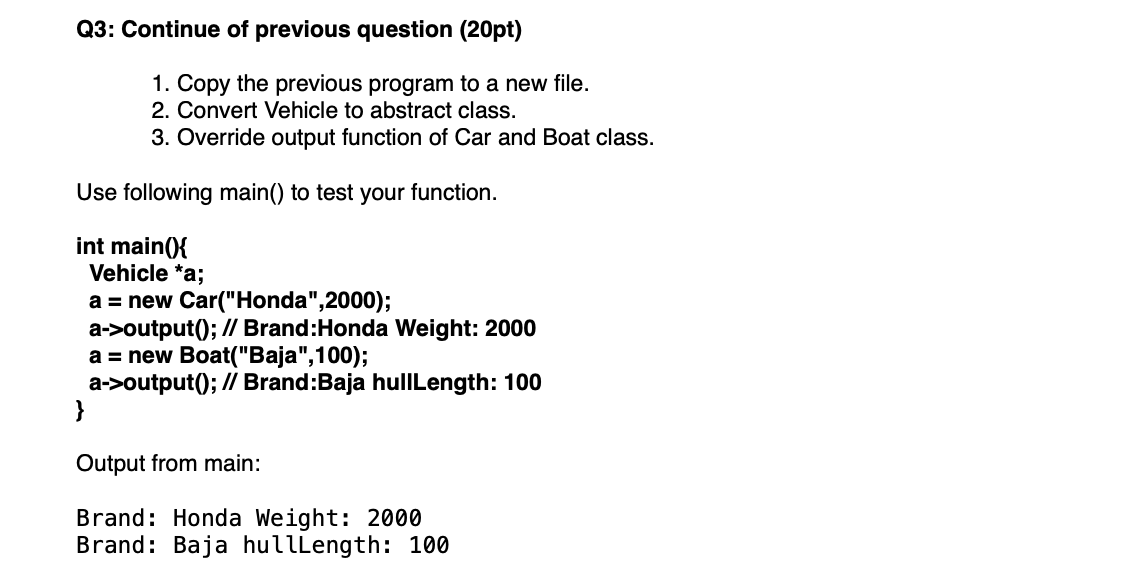

Step by step
Solved in 3 steps

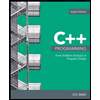
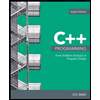