use Java. Given main(), define the Product class (in file Product.java) that will manage product inventory. Product class has three private member fields: a product code (String), the product's price (double), and the number count of product in inventory (int). Implement the following Constructor and member methods:
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
7.23 LAB: Product class
Given main(), define the Product class (in file Product.java) that will manage product inventory. Product class has three private member fields: a product code (String), the product's price (double), and the number count of product in inventory (int).
Implement the following Constructor and member methods:
- public Product(String code, double price, int count) - set the member fields using the three parameters
- public void setCode(String code) - set the product code (i.e. SKU234) to parameter code
- public String getCode() - return the product code
- public void setPrice(double p) - set the price to parameter p
- public double getPrice() - return the price
- public void setCount(int num) - set the number of items in inventory to parameter num
- public int getCount() - return the count
- public void addInventory(int amt) - increase inventory by parameter amt
- public void sellInventory(int amt) - decrease inventory by parameter amt
Ex. If a new Product object is created with code set to "Apple", price set to 0.40, and the count set to 3, the output is:
Name: AppleEx. If 10 apples are added to the Product object's inventory, but then 5 are sold, the output is:
Name: AppleEx. If the Product object's code is set to "Golden Delicious", price is set to 0.55, and count is set to 4, the output is:
Name: Golden Deliciouspublic class LabProgram {
public static void main(String args[]){
String name = "Apple";
double price = 0.40;
int num = 3;
Product p = new Product(name, price, num);
// Test 1 - Are instance variables set/returned properly?
System.out.println("Name: " + p.getCode()); // Should be 'Apple'
System.out.printf("Price: %.2f\n", p.getPrice()); // Should be '0.40'
System.out.println("Count: " + p.getCount()); // Should be 3
System.out.println();
// Test 2 - Are instance variables set/returned properly after adding and selling?
num = 10;
p.addInventory(num);
num = 5;
p.sellInventory(num);
System.out.println("Name: " + p.getCode()); // Should be 'Apple'
System.out.printf("Price: %.2f\n", p.getPrice()); // Should be '0.40'
System.out.println("Count: " + p.getCount()); // Should be 8
System.out.println();
// Test 3 - Do setters work properly?
name = "Golden Delicious";
p.setCode(name);
price = 0.55;
p.setPrice(price);
num = 4;
p.setCount(num);
System.out.println("Name: " + p.getCode()); // Should be 'Golden Delicious'
System.out.printf("Price: %.2f\n", p.getPrice()); // Should be '0.55'
System.out.println("Count: " + p.getCount()); // Should be 4
}
}

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

First time on this site. I don't see the anwser to this problem. Has it been anwsered?
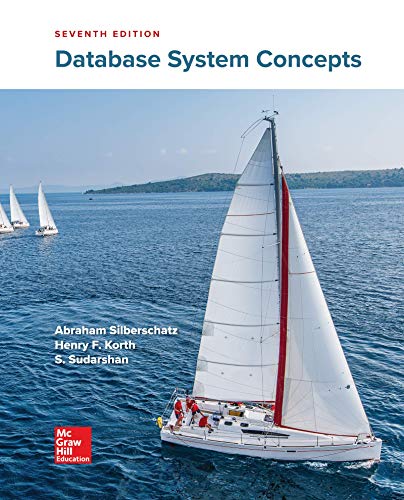
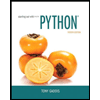
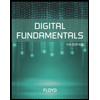
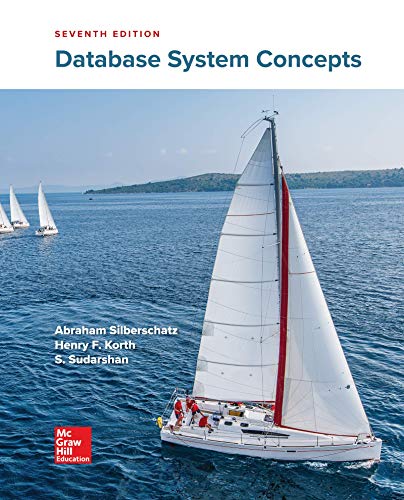
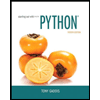
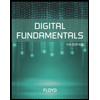
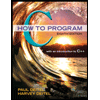
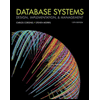
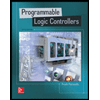