QUESTION Write a complete C++ program that helps the Ministry of Health (MOH) to determine the status of a zone by calculating the number of active cases for COVID 19. The program should perform the following tasks: Task 1: Write a function named dispstatus. a) This is a non-retuming function. b) It takes the number of active cases as an input parameter. e) The function should display the status of a zone based on the conditions in Table 1. Table 1 Number of active cases Status of zone Red Above 40 21 until 40 Orange 1 until 20 Yellow No case Green Task 2: Write a function named getInput. a) This is a non-returning function. b) It takes the number of total cases, new cases, total death, and total recovered as input parameters. c) The function should ask the user to enter the number of total cases, new cases, total death, and total recovered. d) It sends all the values entered by the user in (c) back to the calling module through the use of reference parameters. Task 3: Write a function named dispoutput. a) This is a non-returning function. b) It takes the number of active cases as an input parameter. c) The function should display the number of active cases and zone status by calling the dispstatus function. Task 4: Write a function named calcAverage. a) It takes the number of states and total active cases as input parameters. The function should calculate the average number of active cases per state. b) c) It should return the average value calculated in (b). Task 5: Write a main function to perform the following tasks: a) You need to use an appropriate LOOP to perform the process in this function. The loop will be repeated when the user press ENTER. b) You are NOT ALLOWED to use arrays except an array of characters. c) The function should ask the user to enter a state name. d) The function may need to call the functions that are defined in Task 2, Task 3, and Task 4. e) The function should calculate the number of active cases for Covid 19 using the following formula: Number of active cases = Total cases + New cases- Total Death- Total Recovered ) The function should determine the state with the highest number of active cases and calculate the number of states, and the total number of active cases for all states. Note: You are NOT ALLOWED to use any predefined function(s) to determine the highest number of active cases. g) The program should produce the output as in the sample execution given below. Note: The values in bold are input by the user. Task 6: List all function prototypes. Task 7: You must ensure your program fulfill the following criteria: a) The program is able to run. b) The program uses an appropriate structure for the program (e.g. all required header files are included, the program is properly written, proper indentation, etc.)
QUESTION Write a complete C++ program that helps the Ministry of Health (MOH) to determine the status of a zone by calculating the number of active cases for COVID 19. The program should perform the following tasks: Task 1: Write a function named dispstatus. a) This is a non-retuming function. b) It takes the number of active cases as an input parameter. e) The function should display the status of a zone based on the conditions in Table 1. Table 1 Number of active cases Status of zone Red Above 40 21 until 40 Orange 1 until 20 Yellow No case Green Task 2: Write a function named getInput. a) This is a non-returning function. b) It takes the number of total cases, new cases, total death, and total recovered as input parameters. c) The function should ask the user to enter the number of total cases, new cases, total death, and total recovered. d) It sends all the values entered by the user in (c) back to the calling module through the use of reference parameters. Task 3: Write a function named dispoutput. a) This is a non-returning function. b) It takes the number of active cases as an input parameter. c) The function should display the number of active cases and zone status by calling the dispstatus function. Task 4: Write a function named calcAverage. a) It takes the number of states and total active cases as input parameters. The function should calculate the average number of active cases per state. b) c) It should return the average value calculated in (b). Task 5: Write a main function to perform the following tasks: a) You need to use an appropriate LOOP to perform the process in this function. The loop will be repeated when the user press ENTER. b) You are NOT ALLOWED to use arrays except an array of characters. c) The function should ask the user to enter a state name. d) The function may need to call the functions that are defined in Task 2, Task 3, and Task 4. e) The function should calculate the number of active cases for Covid 19 using the following formula: Number of active cases = Total cases + New cases- Total Death- Total Recovered ) The function should determine the state with the highest number of active cases and calculate the number of states, and the total number of active cases for all states. Note: You are NOT ALLOWED to use any predefined function(s) to determine the highest number of active cases. g) The program should produce the output as in the sample execution given below. Note: The values in bold are input by the user. Task 6: List all function prototypes. Task 7: You must ensure your program fulfill the following criteria: a) The program is able to run. b) The program uses an appropriate structure for the program (e.g. all required header files are included, the program is properly written, proper indentation, etc.)
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter6: User-defined Functions
Section: Chapter Questions
Problem 1TF: Mark the following statements as true or false:
a. To use a predefined function in a program, you...
Related questions
Question
help(:
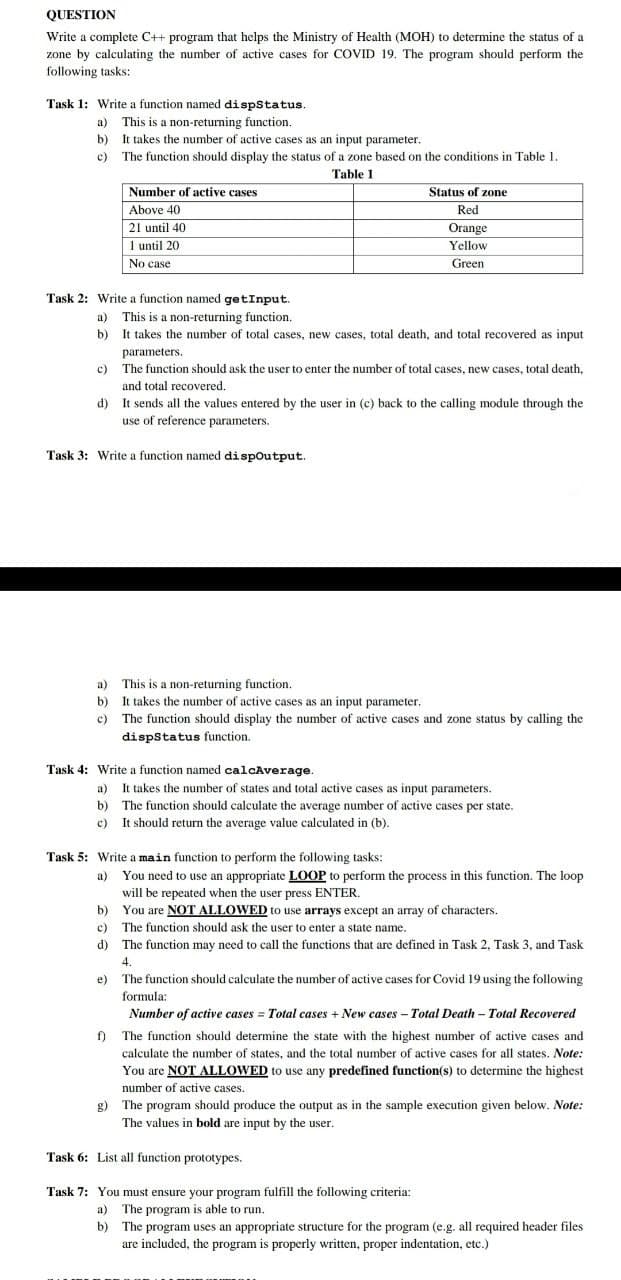
Transcribed Image Text:QUESTION
Write a complete C++ program that helps the Ministry of Health (MOH) to determine the status of a
zone by calculating the number of active cases for COVID 19. The program should perform the
following tasks:
Task 1: Write a function named dispstatus.
a) This is a non-returning function.
b) It takes the number of active cases as an input parameter.
c) The function should display the status of a zone based on the conditions in Table 1.
Table 1
Number of active cases
Above 40
Status of zone
Red
21 until 40
Orange
1 until 20
Yellow
No case
Green
Task 2: Write a function named getInput.
a) This is a non-returning function.
b) It takes the number of total cases, new cases, total death, and total recovered as input
parameters.
c) The function should ask the user to enter the number of total cases, new cases, total death,
and total recovered.
d) It sends all the values entered by the user in (c) back to the calling module through the
use of reference parameters.
Task 3: Write a function named dispoutput.
This is a non-returning function.
b) It takes the number of active cases as an input parameter.
c) The function should display the number of active cases and zone status by calling the
dispstatus function.
a)
Task 4: Write a function named calcAverage.
a) It takes the number of states and total active cases as input parameters.
b) The function should calculate the average number of active cases per state.
c) It should return the average value calculated in (b).
Task 5: Write a main function to perform the following tasks:
a) You need to use an appropriate LOOP to perform the process in this function. The loop
will be repeated when the user press ENTER.
b) You are NOT ALLOWED to use arrays except an array of characters.
c) The function should ask the user to enter a state name.
d) The function may need to call the functions that are defined in Task 2, Task 3, and Task
4.
The function should calculate the number of active cases for Covid 19 using the following
formula:
Number of active cases = Total cases + New cases - Total Death - Total Recovered
e)
f) The function should determine the state with the highest number of active cases and
calculate the number of states, and the total number of active cases for all states. Note:
You are NOT ALLOWED to use any predefined function(s) to determine the highest
number of active cases.
g) The program should produce the output as in the sample execution given below. Note:
The values in bold are input by the user.
Task 6: List all function prototypes.
Task 7: You must ensure your program fulfill the following criteria:
a) The program is able to run.
b) The program uses an appropriate structure for the program (e.g. all required header files
are included, the program is properly written, proper indentation, etc.)
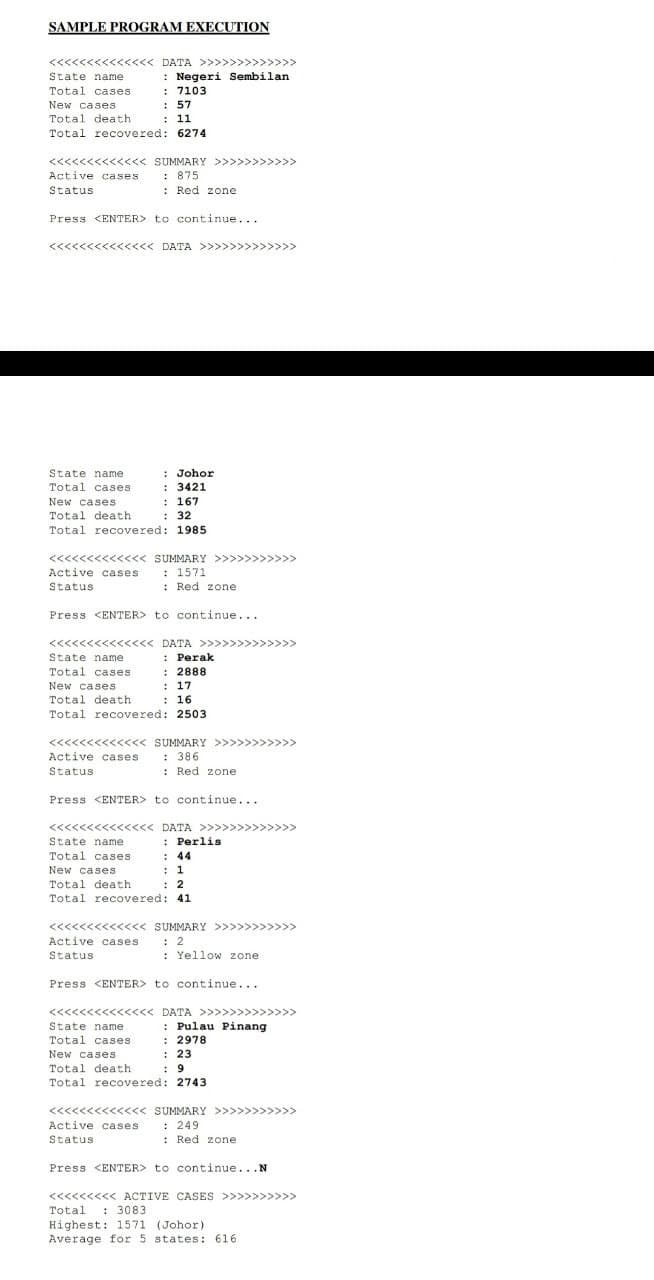
Transcribed Image Text:SAMPLE PROGRAM EXECUTION
<<<<<<<<<<<<<< DATA >>>>>>>>>>>>>
: Negeri Sembilan
: 7103
: 57
: 11
State name
Total cases
New cases
Total death
Total recovered: 6274
くくくくくくくくくくくくく SUMMARY >>>>>>>>>>>
: 875
: Red zone
Active cases
Status
Press <ENTER> to continue...
<<<<<<<<<<<<<< DATA >>>>>>>>>>>>>
: Johor
: 3421
: 167
: 32
State name
Total cases
New cases
Total death
Total recovered: 1985
<<<<<<<<<<<<< SUMMARY >>>>>>>>>>>
: 1571
: Red zone
Active cases
Status
Press <ENTER> to continue...
<<<<<<<<<<<<<< DATA >>>>>>>>>>>>>
: Perak
: 2888
: 17
: 16
State name
Total cases
New cases
Total death
Total recovered: 2503
くくくくくくくくくくくくく SUMMARY >>>>>>>>>>>
: 386
: Red zone
Active cases
Status
Press <ENTER> to continue...
くくくくくくくくくくくくくく DATA >>>>>>>>>>>>>
: Perlis
: 44
: 1
: 2
State name
Total cases
New cases
Total death
Total recovered: 41
<<<<<<<<<<<<< SUMMARY >>>>>>>>>>>
: 2
: Yellow zone
Active cases
Status
Press <ENTER> to continue...
<<<<<<<<<<<<<< DATA >>>>>>>>>>>>>
: Pulau Pinang
: 2978
: 23
: 9
State namme
Total cases
New cases
Total death
Total recovered: 2743
<<<<<<<<<<<<< SUMMARY >>>>>>>>>>>
: 249
: Red zone
Active cases
Status
Press <ENTER> to continue...N
<<<<<<<<< ACTIVE CASES >>>>>>>>>>
Total : 3083
Highest: 1571 (Johor)
Average for 5 states: 616
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
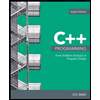
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
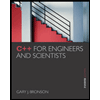
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
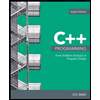
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
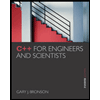
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr