mplement in C Programming 7.6.1: LAB: Simple car Given two integers that represent the miles to drive forward and the miles to drive in reverse as user inputs, create a SimpleCar variable that performs the following operations: Drives input number of miles forward Drives input number of miles in reverse Honks the horn Reports car status SimpleCar.h contains the struct definition and related function declarations. SimpleCar.c contains related function definitions. Ex: If the input is: 100 4 the output is: beep beep Car has driven: 96 miles main.c #include #include "SimpleCar.h" int main() { /* Type your code here. */ return 0; } SimpleCar.h #ifndef SIMPLE_CAR_H #define SIMPLE_CAR_H typedef struct SimpleCar_struct { int miles; } SimpleCar; SimpleCar InitCar(); SimpleCar Drive(int dist, SimpleCar car); SimpleCar Reverse(int dist, SimpleCar car); int GetOdometer(SimpleCar car); void HonkHorn(SimpleCar car); void Report(SimpleCar car); #endif SimpleCar.c #include #include #include "SimpleCar.h" SimpleCar InitCar(){ SimpleCar newCar; newCar.miles = 0; return newCar; } SimpleCar Drive(int dist, SimpleCar car){ car.miles = car.miles + dist; return car; } SimpleCar Reverse(int dist, SimpleCar car){ car.miles = car.miles - dist; return car; } int GetOdometer(SimpleCar car){ return car.miles; } void HonkHorn(SimpleCar car){ printf("beep beep\n"); } void Report(SimpleCar car){ printf("Car has driven: %d miles\n", car.miles); }
Implement in C
7.6.1: LAB: Simple car
Given two integers that represent the miles to drive forward and the miles to drive in reverse as user inputs, create a SimpleCar variable that performs the following operations:
- Drives input number of miles forward
- Drives input number of miles in reverse
- Honks the horn
- Reports car status
SimpleCar.h contains the struct definition and related function declarations. SimpleCar.c contains related function definitions.
Ex: If the input is:
100 4
the output is:
beep beep Car has driven: 96 miles
main.c
#include <stdio.h>
#include "SimpleCar.h"
int main() {
/* Type your code here. */
return 0;
}
SimpleCar.h
#ifndef SIMPLE_CAR_H
#define SIMPLE_CAR_H
typedef struct SimpleCar_struct {
int miles;
} SimpleCar;
SimpleCar InitCar();
SimpleCar Drive(int dist, SimpleCar car);
SimpleCar Reverse(int dist, SimpleCar car);
int GetOdometer(SimpleCar car);
void HonkHorn(SimpleCar car);
void Report(SimpleCar car);
#endif
SimpleCar.c
#include <stdio.h>
#include <string.h>
#include "SimpleCar.h"
SimpleCar InitCar(){
SimpleCar newCar;
newCar.miles = 0;
return newCar;
}
SimpleCar Drive(int dist, SimpleCar car){
car.miles = car.miles + dist;
return car;
}
SimpleCar Reverse(int dist, SimpleCar car){
car.miles = car.miles - dist;
return car;
}
int GetOdometer(SimpleCar car){
return car.miles;
}
void HonkHorn(SimpleCar car){
printf("beep beep\n");
}
void Report(SimpleCar car){
printf("Car has driven: %d miles\n", car.miles);
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

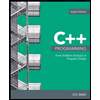
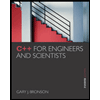
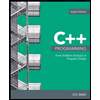
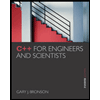