QUESTIONS = 1. Why are the parameters x and y used in the function definitions rather than first_num/second_num? = 2. What is meant by local and global scope of variables? = 3. What is the purpose of the return statenent? = TASKS = 1. Write a new function to multiply two numbers together and return the result = 2. Write a neu function to divide two nunbers and return the result. Run the progran and try entening '0' as the second number what happens? How COuld you fix this? = 3. Write a nen function to display a menu whích should allou the user to change the numbers or choose which operation they would like to perform, e.g. choose from addition, multiplication, etc = 4. Modify your menu function to include an option to exit the program and add a loop to allom the user to restart the program until they choose the exit option E 5. Write a neu function which takes two argunents, a number and a power and returns the numbertpower. You should research and use the math.pow() function to do this. What is the difference between nath.pow() and **? E6. Write a new function which takes two arguments, a number and the nth root (e.g. 2 would be square root, 3 cubic root, etc) and returns the nth root of the number. You should research an appropriate way to do this
QUESTIONS = 1. Why are the parameters x and y used in the function definitions rather than first_num/second_num? = 2. What is meant by local and global scope of variables? = 3. What is the purpose of the return statenent? = TASKS = 1. Write a new function to multiply two numbers together and return the result = 2. Write a neu function to divide two nunbers and return the result. Run the progran and try entening '0' as the second number what happens? How COuld you fix this? = 3. Write a nen function to display a menu whích should allou the user to change the numbers or choose which operation they would like to perform, e.g. choose from addition, multiplication, etc = 4. Modify your menu function to include an option to exit the program and add a loop to allom the user to restart the program until they choose the exit option E 5. Write a neu function which takes two argunents, a number and a power and returns the numbertpower. You should research and use the math.pow() function to do this. What is the difference between nath.pow() and **? E6. Write a new function which takes two arguments, a number and the nth root (e.g. 2 would be square root, 3 cubic root, etc) and returns the nth root of the number. You should research an appropriate way to do this
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter6: User-defined Functions
Section: Chapter Questions
Problem 9SA
Related questions
Question
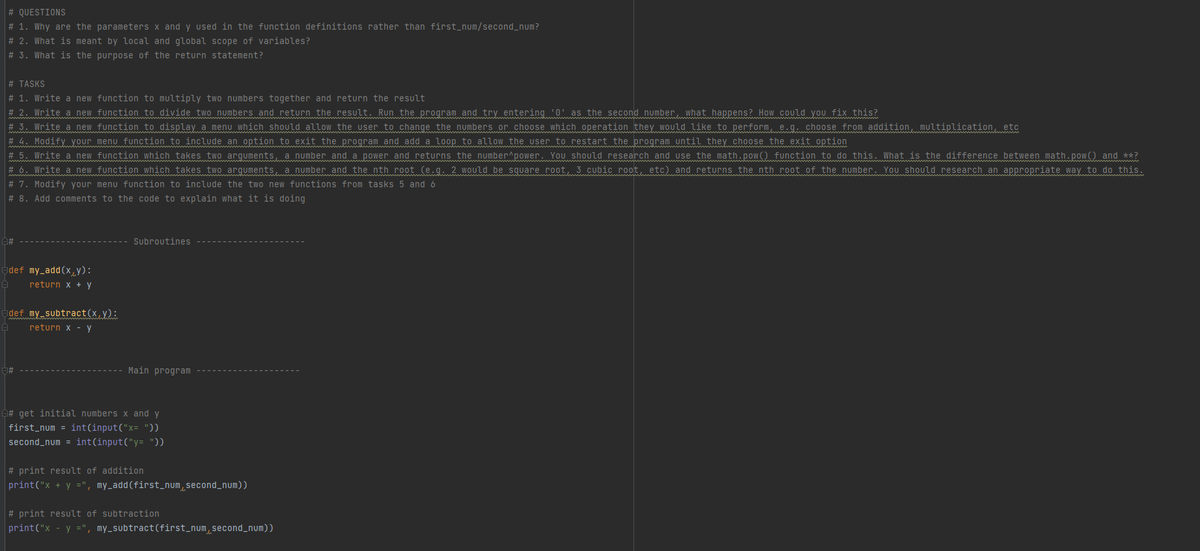
Transcribed Image Text:# QUESTIONS
# 1. Why are the parameters x and y used in the function definitions rather than first_num/second_num?
# 2. What is meant by local and global scope of variables?
# 3. What is the purpose of the return statement?
# TASKS
# 1. Write a new function to multiply two numbers together and return the result
# 2. Write a new function to divide two numbers and return the result. Run the program and try entering '0' as the second number, what happens? How could you fix this?
# 3. Write a new function to display a menu which should allow the user to change the numbers or choose which operation they would like to perform, e.g. choose from addition, multiplication, etc
# 4. Modify your menu function to include an option to exit the program and add a loop to allow the user to restart the program until they choose the exit option
# 5. Write a new function which takes two arguments, a number and a power and returns the number^power. You should research and use the math.pow() function to do this. What is the difference between math.pow() and **?
# 6. Write a new function which takes two arguments, a number and the nth root (e.g. 2 would be square root, 3 cubic root
etc) and returns the nth root of the number. You should research an appropriate way to do this.
# 7. Modify your menu function to include the two new functions from tasks 5 and 6
# 8. Add comments to the code to explain what it is doing
白#
Subroutines
Edef my_add(x,y):
return x + y
def my subtract(x,y):
return x - y
%23
Main program
A# get initial numbers x and y
first_num = int(input("x= "))
second_num = int(input("y= "))
# print result of addition
print("x + y =", my_add(first_num second_num))
# print result of subtraction
print("x - y =", my_subtract(first_num, second_num))
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
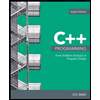
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
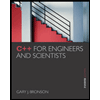
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
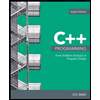
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
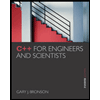
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr