Create a function that will determine whether one integer is a multiple of another. For example, if 20 is divisible by 4 then 20 is a multiple of 4. Requirements: 1. Call the function isMultiple. 2. Have the function receive two integer arguments through its parameter list. Name the two variables firstlnt and secondInt. 3. The function will return a Boolean result of whether the first integer is a multiple of the second integer. 4. Write a driver for this function to test it. Remember, a driver function is simply the main function that is specifically written to test a function. a. Name the first number entered: intFirst b. Name the second number entered: intSecond 5. Test isMultiple using the following data: a. Test 1: intFirst = 35 and intSecond = 7 b. Test 2: intFirst = 7 and intSecond = 35 c. Test 3: intFirst = 25 and intSecond = 3 d. Test 4: intFirst = 8 and intSecond = 3 e. Test 5: intFirst = 16 and intSecond = 2 f. Test 6: intFirst = 2 and intSecond = 16 6. Be sure to print the results of each test. a. The message should indicate whether or not the two numbers are multiples. Do not print true or false. b. Do NOT print the message in the function. The message should be printed within the main method. 7. Create an outer loop with a prompt asking the user if they want to enter another set of numbers.
Create a function that will determine whether one integer is a multiple of another. For example, if 20 is divisible by 4 then 20 is a multiple of 4. Requirements: 1. Call the function isMultiple. 2. Have the function receive two integer arguments through its parameter list. Name the two variables firstlnt and secondInt. 3. The function will return a Boolean result of whether the first integer is a multiple of the second integer. 4. Write a driver for this function to test it. Remember, a driver function is simply the main function that is specifically written to test a function. a. Name the first number entered: intFirst b. Name the second number entered: intSecond 5. Test isMultiple using the following data: a. Test 1: intFirst = 35 and intSecond = 7 b. Test 2: intFirst = 7 and intSecond = 35 c. Test 3: intFirst = 25 and intSecond = 3 d. Test 4: intFirst = 8 and intSecond = 3 e. Test 5: intFirst = 16 and intSecond = 2 f. Test 6: intFirst = 2 and intSecond = 16 6. Be sure to print the results of each test. a. The message should indicate whether or not the two numbers are multiples. Do not print true or false. b. Do NOT print the message in the function. The message should be printed within the main method. 7. Create an outer loop with a prompt asking the user if they want to enter another set of numbers.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter6: User-defined Functions
Section: Chapter Questions
Problem 9SA
Related questions
Question
In C++ please
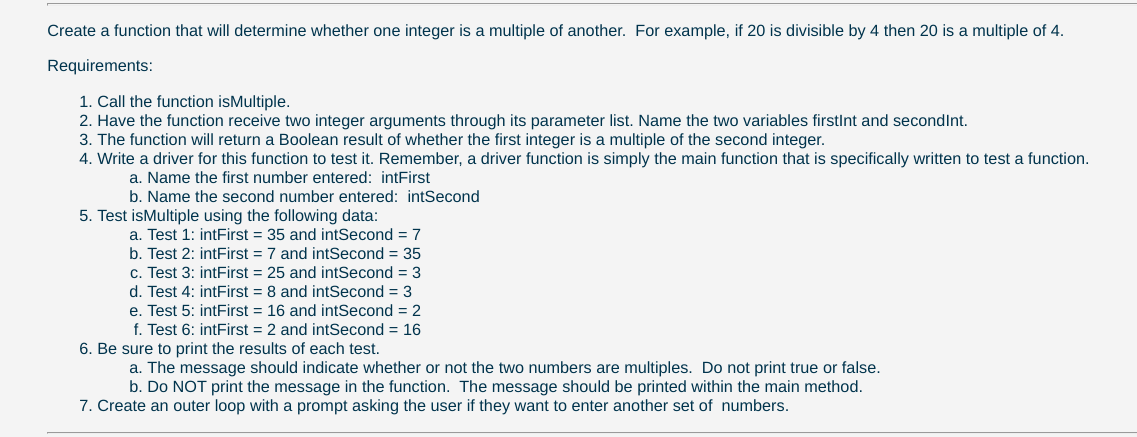
Transcribed Image Text:Create a function that will determine whether one integer is a multiple of another. For example, if 20 is divisible by 4 then 20 is a multiple of 4.
Requirements:
1. Call the function isMultiple.
2. Have the function receive two integer arguments through its parameter list. Name the two variables firstlnt and secondInt.
3. The function will return a Boolean result of whether the first integer is a multiple of the second integer.
4. Write a driver for this function to test it. Remember, a driver function is simply the main function that is specifically written to test a function.
a. Name the first number entered: intFirst
b. Name the second number entered: intSecond
5. Test isMultiple using the following data:
a. Test 1: intFirst = 35 and intSecond = 7
b. Test 2: intFirst = 7 and intSecond = 35
c. Test 3: intFirst = 25 and intSecond = 3
d. Test 4: intFirst = 8 and intSecond = 3
e. Test 5: intFirst = 16 and intSecond = 2
f. Test 6: intFirst = 2 and intSecond = 16
6. Be sure to print the results of each test.
a. The message should indicate whether or not the two numbers are multiples. Do not print true or false.
b. Do NOT print the message in the function. The message should be printed within the main method.
7. Create an outer loop with a prompt asking the user if they want to enter another set of numbers.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
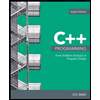
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
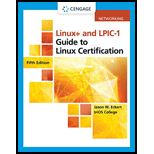
LINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.
Computer Science
ISBN:
9781337569798
Author:
ECKERT
Publisher:
CENGAGE L
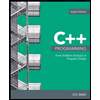
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
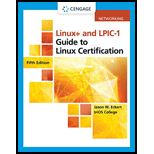
LINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.
Computer Science
ISBN:
9781337569798
Author:
ECKERT
Publisher:
CENGAGE L