Read the following program named myArray.cpp carefully. Complete all missing code as indicated by the comments. Use the following command lines to compile and test your program before your submission: c++ -o myArray myArray.cpp ./myArray In the main function, you need to Declare constant integers n1=32, n2=16, and m=8 Declare a character array a1[n1] and an integer array a2[n2] and setup array elements randomly, where a1 contains uppercase letters A~Z only, and the values of a2 satisfies 0≤v<512 Display a1 and a2, m elements per line // Student Name // Student ID //myArray.cpp #include #include #include using namespace std; template class myArray { T *ptr; // body of array int size; // size of array public: myArray(int s); // constructor ~myArray(){free(ptr);} // destructor void setValue(int i, T v){ptr[i]=v;} // set value T getValue(int i){return ptr[i];} // get value int getSize(){return size;} // get size void display(int); // display elements }; // constructor, to be implemented template myArray::myArray(int s){} // display elements, m elements per line, to be implemented template void myArray::display(int m){} int main(){ // declare three constant integers n1=32, n2=16, and m=8 // declare a character array a1[n1] and an integer array a2[n2] // setup a1 randomly, where a1 contains uppercase letters A~Z only // setup a2 randomly, where the value of an element satisfies 0≤v<512 // display a1 and a2 return 0; }
Read the following program named myArray.cpp carefully. Complete all missing code as indicated by the comments. Use the following command lines to compile and test your program before your submission:
c++ -o myArray myArray.cpp <enter>
./myArray <enter>
In the main function, you need to
- Declare constant integers n1=32, n2=16, and m=8
- Declare a character array a1[n1] and an integer array a2[n2] and setup array elements randomly, where a1 contains uppercase letters A~Z only, and the values of a2 satisfies 0≤v<512
- Display a1 and a2, m elements per line
// Student Name
// Student ID
//myArray.cpp
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
template <class T> class myArray {
T *ptr; // body of array
int size; // size of array
public:
myArray(int s); // constructor
~myArray(){free(ptr);} // destructor
void setValue(int i, T v){ptr[i]=v;} // set value
T getValue(int i){return ptr[i];} // get value
int getSize(){return size;} // get size
void display(int); // display elements
};
// constructor, to be implemented
template <class T> myArray<T>::myArray(int s){}
// display elements, m elements per line, to be implemented
template <class T> void myArray<T>::display(int m){}
int main(){
// declare three constant integers n1=32, n2=16, and m=8
// declare a character array a1[n1] and an integer array a2[n2]
// setup a1 randomly, where a1 contains uppercase letters A~Z only
// setup a2 randomly, where the value of an element satisfies 0≤v<512
// display a1 and a2
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

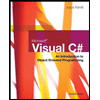
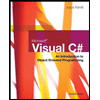