Read This Carefully!! (For your eyes-only) Your task, should you choose to accept it, is to design an application that will accept any phrase as input and allow the user to encrypt or decrypt it. The program should use one or two keys (two is highly recommended) to encrypt the message. These keys could be made up of an integer - a number between -32767 and +32768 or anything else you think might work. As an example, the key could be used to shift the letters of the alphabet to the right or left. For example, the letter "A" encoded with a key of "3" would produce the letter "D", three letters to its right in the alphabet. If the key is large enough that it goes past the end of the alphabet, the program should "wrap around" to a letter near the beginning of the alphabet. For example the letter "Z" encoded with a key of 3 would become "C". The decoding process should be done in reverse. You can use this scheme if you wish, but I challenge you to design one that I cannot crack! Program Restrictions: .Keep lower case letters as lower case and upper case letters as upper case. Make no changes to all non-letters (i.e. numbers, punctuation, special characters, etc.) AND these non-letter characters do NOT need to be encrypted. Suggested Program structure: Your program MAY include the following four methods. It may have different/additional ones. Each method must identify its author through commenting. public static boolean isALetter (String letter) Inputs a one character string and returns True if the character is a letter (i.e. a to z or A to Z). Returns false if it is not. A function to check that the password or encryption key is valid. For example if you decide to use the algorithm above: public static int putKeyInRange (int encryptKey) • Takes any key in the integer range (-32767 to +32768) as input and returns a number between 1 and 26 (the range of the alphabet). (hint: check if input is within the alphabet) public static char encode (char letter, int encryKey) • Inputs one character and an integer key and returns an encoded character based on the key. public static char decode (char letter, int encryKey) • Does the opposite of encode. (Hint: have it call the encode function with an opposite key.)
Read This Carefully!! (For your eyes-only) Your task, should you choose to accept it, is to design an application that will accept any phrase as input and allow the user to encrypt or decrypt it. The program should use one or two keys (two is highly recommended) to encrypt the message. These keys could be made up of an integer - a number between -32767 and +32768 or anything else you think might work. As an example, the key could be used to shift the letters of the alphabet to the right or left. For example, the letter "A" encoded with a key of "3" would produce the letter "D", three letters to its right in the alphabet. If the key is large enough that it goes past the end of the alphabet, the program should "wrap around" to a letter near the beginning of the alphabet. For example the letter "Z" encoded with a key of 3 would become "C". The decoding process should be done in reverse. You can use this scheme if you wish, but I challenge you to design one that I cannot crack! Program Restrictions: .Keep lower case letters as lower case and upper case letters as upper case. Make no changes to all non-letters (i.e. numbers, punctuation, special characters, etc.) AND these non-letter characters do NOT need to be encrypted. Suggested Program structure: Your program MAY include the following four methods. It may have different/additional ones. Each method must identify its author through commenting. public static boolean isALetter (String letter) Inputs a one character string and returns True if the character is a letter (i.e. a to z or A to Z). Returns false if it is not. A function to check that the password or encryption key is valid. For example if you decide to use the algorithm above: public static int putKeyInRange (int encryptKey) • Takes any key in the integer range (-32767 to +32768) as input and returns a number between 1 and 26 (the range of the alphabet). (hint: check if input is within the alphabet) public static char encode (char letter, int encryKey) • Inputs one character and an integer key and returns an encoded character based on the key. public static char decode (char letter, int encryKey) • Does the opposite of encode. (Hint: have it call the encode function with an opposite key.)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Topic Video
Question
Please help me. Description of what to do is below. A user must be able to enter any phrase or sentence that they would like to encrypt or decrypt
Then if you can in a txt file create a gui for that program. An example is below in the photos
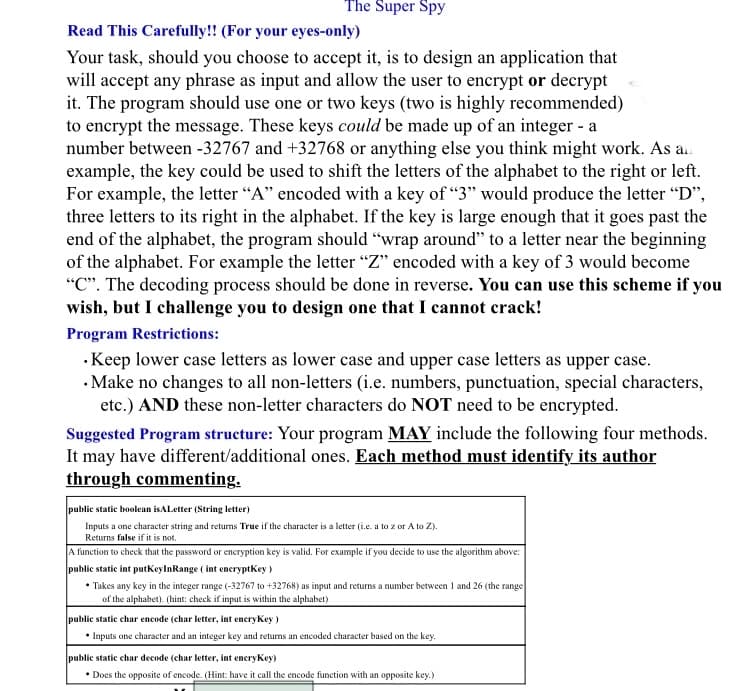
Transcribed Image Text:The Super Spy
Read This Carefully!! (For your eyes-only)
Your task, should you choose to accept it, is to design an application that
will accept any phrase as input and allow the user to encrypt or decrypt
it. The program should use one or two keys (two is highly recommended)
to encrypt the message. These keys could be made up of an integer - a
number between -32767 and +32768 or anything else you think might work. As an
example, the key could be used to shift the letters of the alphabet to the right or left.
For example, the letter "A" encoded with a key of "3" would produce the letter "D",
three letters to its right in the alphabet. If the key is large enough that it goes past the
end of the alphabet, the program should "wrap around" to a letter near the beginning
of the alphabet. For example the letter "Z" encoded with a key of 3 would become
"C". The decoding process should be done in reverse. You can use this scheme if you
wish, but I challenge you to design one that I cannot crack!
Program Restrictions:
• Keep lower case letters as lower case and upper case letters as upper case.
• Make no changes to all non-letters (i.e. numbers, punctuation, special characters,
etc.) AND these non-letter characters do NOT need to be encrypted.
Suggested Program structure: Your program MAY include the following four methods.
It may have different/additional ones. Each method must identify its author
through commenting.
public static boolean is ALetter (String letter)
Inputs a one character string and returns True if the character is a letter (i.e. a to z or A to Z).
Returns false if it is not.
A function to check that the password or encryption key is valid. For example if you decide to use the algorithm above:
public static int putKeyInRange (int encryptKey)
• Takes any key in the integer range (-32767 to +32768) as input and returns a number between 1 and 26 (the range
of the alphabet). (hint: check if input is within the alphabet)
public static char encode (char letter, int encryKey)
• Inputs one character and an integer key and returns an encoded character based on the key.
public static char decode (char letter, int encryKey)
• Does the opposite of encode. (Hint: have it call the encode function with an opposite key.)
![import java.awt.*;
import java.awt.event.*; // for listening to buttons
import javax.swing.*;
public class GUIExampleApp extends JFrame implements ActionListener
H
// Declare class variables for labels and buttons
// and variables to be used throughout the class
JLabel lblInput, lbloutput;
JTextField txtInput, txtOutput;
JButton btnReverse, btnClear;
Container frame;
// declare the container frame
public GUIExampleApp () // constructing the window
(
super ("GUIExampleApp");
// Set the frame's name
frame getContent Pane (); // get the container frame
// Create labels, text boxes and buttons
lblInput new JLabel ("Enter a phrase");
1b10utput= new JLabel ("Output String");
txtInput= new JTextField (50);
txtOutput= new JTextField (50);
btnReverse new JButton ("Reverse String");
btnClear new JButton ("Clear");
// set the size and layout of the applet.
frame.setLayout (new GridLayout (6, 1));
// add the labels, textboxes and buttons
// The order matters!!
frame.add (lblInput);
frame.add (txtInput);
frame.add (lbloutput);
frame.add (txtOutput);
frame.add (btnReverse);
frame.add (btnClear);
// make the buttons listen to clicks on this application
btnReverse.addActionListener
(this);
btnClear.addActionListener (this);
setSize (300, 200);
setVisible (true);
// Set the frame's size
// Show it.
} // Constructor
public void actionPerformed (ActionEvent e)
(
// check which button was clicked
if (e.getSource ()-btnReverse)
(
// declare strings for input and output
String phraseIn, phraseOut;
phraseIn txtInput.getText (); // get the input string
phraseOut
String Processor.strReverse (phrase In); //
reverse the string
txtOutput.setText (phraseOut); //output on the textfield
}
else if (e.getSource () == btnClear)
(
txt Input.setText (""); // clear both strings
txtOutput.setText ("");
}
// public void paint (Graphics g).
// pu
public
// Not used in our case
// ) // paint method
public static void main (String[] args)
(
new GUIExampleApp (); // Create a GUIExampleApp frame
) // main method
) // GUIExampleApp class](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F815f7d1e-9a0f-4d9f-a027-2424a4e2482a%2F00f6ffae-7fe0-4f9b-ad0d-5f2e8db29fee%2Fpewrg48_processed.jpeg&w=3840&q=75)
Transcribed Image Text:import java.awt.*;
import java.awt.event.*; // for listening to buttons
import javax.swing.*;
public class GUIExampleApp extends JFrame implements ActionListener
H
// Declare class variables for labels and buttons
// and variables to be used throughout the class
JLabel lblInput, lbloutput;
JTextField txtInput, txtOutput;
JButton btnReverse, btnClear;
Container frame;
// declare the container frame
public GUIExampleApp () // constructing the window
(
super ("GUIExampleApp");
// Set the frame's name
frame getContent Pane (); // get the container frame
// Create labels, text boxes and buttons
lblInput new JLabel ("Enter a phrase");
1b10utput= new JLabel ("Output String");
txtInput= new JTextField (50);
txtOutput= new JTextField (50);
btnReverse new JButton ("Reverse String");
btnClear new JButton ("Clear");
// set the size and layout of the applet.
frame.setLayout (new GridLayout (6, 1));
// add the labels, textboxes and buttons
// The order matters!!
frame.add (lblInput);
frame.add (txtInput);
frame.add (lbloutput);
frame.add (txtOutput);
frame.add (btnReverse);
frame.add (btnClear);
// make the buttons listen to clicks on this application
btnReverse.addActionListener
(this);
btnClear.addActionListener (this);
setSize (300, 200);
setVisible (true);
// Set the frame's size
// Show it.
} // Constructor
public void actionPerformed (ActionEvent e)
(
// check which button was clicked
if (e.getSource ()-btnReverse)
(
// declare strings for input and output
String phraseIn, phraseOut;
phraseIn txtInput.getText (); // get the input string
phraseOut
String Processor.strReverse (phrase In); //
reverse the string
txtOutput.setText (phraseOut); //output on the textfield
}
else if (e.getSource () == btnClear)
(
txt Input.setText (""); // clear both strings
txtOutput.setText ("");
}
// public void paint (Graphics g).
// pu
public
// Not used in our case
// ) // paint method
public static void main (String[] args)
(
new GUIExampleApp (); // Create a GUIExampleApp frame
) // main method
) // GUIExampleApp class
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
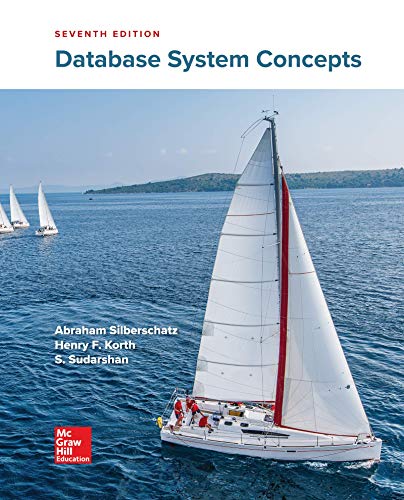
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
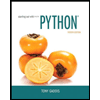
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
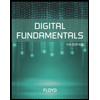
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
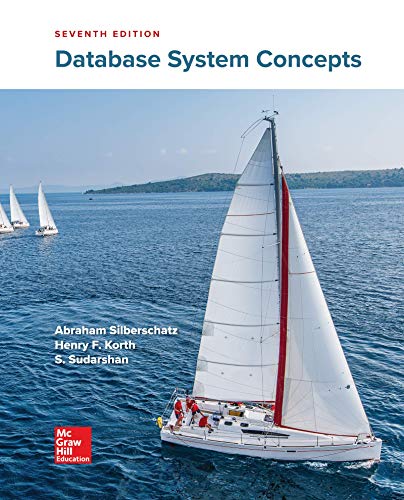
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
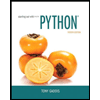
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
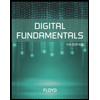
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
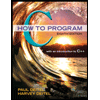
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
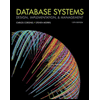
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
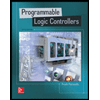
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education