Recall your simple calculator program, add another function to it called result. This function called result should accept four parameters (operation, num1, num2, output) and should print out the output of each arithmetic operation. The output could look like this: "The result of ADDING 10 and 20 is 30" Hint 1: call the result function right after calling the respective operation. Example: x = ADD(n, m) result ("ADDING", n, m, x) Hint 2: Placeholders and string.format() function could be very useful here.
Recall your simple calculator program, add another function to it called result. This function called result should accept four parameters (operation, num1, num2, output) and should print out the output of each arithmetic operation. The output could look like this: "The result of ADDING 10 and 20 is 30" Hint 1: call the result function right after calling the respective operation. Example: x = ADD(n, m) result ("ADDING", n, m, x) Hint 2: Placeholders and string.format() function could be very useful here.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter5: Repetition Statements
Section5.5: A Closer Look: Loop Programming Techniques
Problem 12E: (Program) Write a program that tests the effectiveness of the rand() library function. Start by...
Related questions
Question
THIS IS THE SIMPLE CALCULATOR PROGRAM
def ADD (num1,num2):
sum=num1+num2
print(sum)
def SUBT (num1,num2):
res=num1-num2
print(res)
def MULT (num1,num2):
product=num1*num2
print(product)
def DIV (num1,num2):
results=num1/num2
print(results)
def POW (num1,num2):
power=num1**num2
print(power)
def MOD (num1,num2):
modulo=num1%num2
print(modulo)
def INT (num1,num2):
integer=int(num1/num2)
print(integer)
def PERCENT (num1,num2):
percentage=(num1/num2)*100
print(percentage)
A=int(input("Enter the first number: "))
B=int(input("Enter the second number: "))
ADD(A,B)
SUBT(A,B)
MULT(A,B)
DIV(A,B)
POW(A,B)
MOD(A,B)
INT(A,B)
PERCENT(A,B)
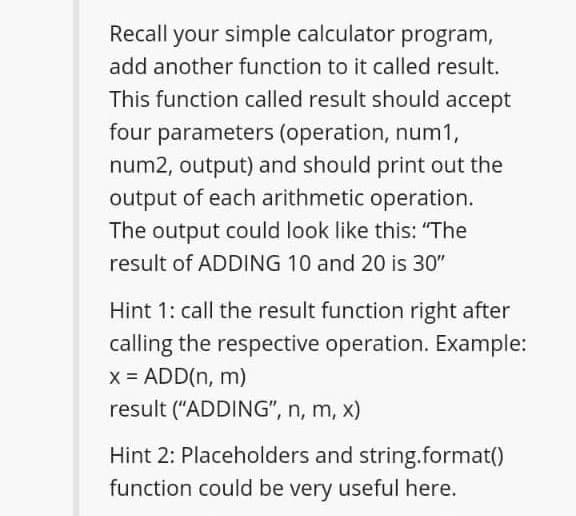
Transcribed Image Text:Recall your simple calculator program,
add another function to it called result.
This function called result should accept
four parameters (operation, num1,
num2, output) and should print out the
output of each arithmetic operation.
The output could look like this: "The
result of ADDING 10 and 20 is 30"
Hint 1: call the result function right after
calling the respective operation. Example:
x = ADD(n, m)
result ("ADDING", n, m, x)
Hint 2: Placeholders and string.format()
function could be very useful here.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
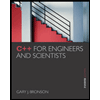
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
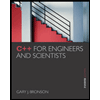
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr