Part-1: Implementing the Course class without constructors: Course name: String number: int instructor: String students: int[] getNumberOfStudents(): int printStudentsIDs(): void addStudent(id: int): void removeStudent(id:int): boolean 1. Inside the body of the Course class: a. Define the data fields name, number, faculty, and students according to their data types in the UML diagram. The students array includes IDs of students registered in the course. For example, the following statement declares the data field name: String name; b. Define the method getNumberOfStudents that returns the number of students registered in the course, which is the size of the students array. c. Define the void method printStudentsIDs which prints IDs of students (elements of the students array) on one line space separated and prints a new line afterwards. d. Define the method addStudent which takes an integer that represents a student ID and add it to the array students. e. Define the method removeStudent which takes an integer that represents a student ID, and searches the students array for the requested ID. If it is found, it must remove it from the array and return true. If it is not found, it must return false. Note: you may assume that each student ID appears only once in the array students. 2. In your main method: a. Declare and create an object of type Course named cl as in the following statement: Course c1= new Course(); b. Print the values of the object cl data fields (name, number, instructor, and students) and observe the output. Print the values using full statements as follows: Course name: .... Course number: .... Course instructor: .... Course students reference variable: .... Note: In order to access data fields of an object we use the dot operator. For example, in order to print the value of name of the object cl, we use the following statement: System.out.println("Course name:" + c1.name); c. Invoke the method printStudentsIDs to print value of the students array elements. Observe and justify the output. Note: in order to invoke the printStudentsIDs method for the object c1, use the dot operator as in the following example: cl.printStudentsIDs(); Remember that since printStudentsIDs is a void method, you must invoke it as a separate statement.
Part-1: Implementing the Course class without constructors: Course name: String number: int instructor: String students: int[] getNumberOfStudents(): int printStudentsIDs(): void addStudent(id: int): void removeStudent(id:int): boolean 1. Inside the body of the Course class: a. Define the data fields name, number, faculty, and students according to their data types in the UML diagram. The students array includes IDs of students registered in the course. For example, the following statement declares the data field name: String name; b. Define the method getNumberOfStudents that returns the number of students registered in the course, which is the size of the students array. c. Define the void method printStudentsIDs which prints IDs of students (elements of the students array) on one line space separated and prints a new line afterwards. d. Define the method addStudent which takes an integer that represents a student ID and add it to the array students. e. Define the method removeStudent which takes an integer that represents a student ID, and searches the students array for the requested ID. If it is found, it must remove it from the array and return true. If it is not found, it must return false. Note: you may assume that each student ID appears only once in the array students. 2. In your main method: a. Declare and create an object of type Course named cl as in the following statement: Course c1= new Course(); b. Print the values of the object cl data fields (name, number, instructor, and students) and observe the output. Print the values using full statements as follows: Course name: .... Course number: .... Course instructor: .... Course students reference variable: .... Note: In order to access data fields of an object we use the dot operator. For example, in order to print the value of name of the object cl, we use the following statement: System.out.println("Course name:" + c1.name); c. Invoke the method printStudentsIDs to print value of the students array elements. Observe and justify the output. Note: in order to invoke the printStudentsIDs method for the object c1, use the dot operator as in the following example: cl.printStudentsIDs(); Remember that since printStudentsIDs is a void method, you must invoke it as a separate statement.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please help me solve this with java
![Part-1: Implementing the Course class without constructors:
Course
name: String
number: int
instructor: String
students: int[]
getNumberOfStudents(): int
printStudentsIDs(): void
addStudent(id: int): void
removeStudent(id:int): boolean
1. Inside the body of the Course class:
a.
Define the data fields name, number, faculty, and students according to their data types in the UML
diagram. The students array includes IDs of students registered in the course.
For example, the following statement declares the data field name:
String name;
b. Define the method getNumberOfStudents that returns the number of students registered in the course,
which is the size of the students array.
c. Define the void method printStudentsIDs which prints IDs of students (elements of the students array)
on one line space separated and prints a new line afterwards.
d. Define the method addStudent which takes an integer that represents a student ID and add it to the
array students.
e. Define the method removeStudent which takes an integer that represents a student ID, and searches
the students array for the requested ID. If it is found, it must remove it from the array and return true.
If it is not found, it must return false.
Note: you may assume that each student ID appears only once in the array students.
2. In your main method:
a. Declare and create an object of type Course named cl as in the following statement:
Course c1= new Course();
b. Print the values of the object cl data fields (name, number, instructor, and students) and observe the
output. Print the values using full statements as follows:
Course name: ....
Course number: ....
Course instructor: ....
Course students reference variable: ....
Note: In order to access data fields of an object we use the dot operator. For example, in order to print
the value of name of the object c1, we use the following statement:
System.out.println("Course name:" + c1.name);
c. Invoke the method printStudentsIDs to print value of the students array elements. Observe and justify
the output.
Note: in order to invoke the printStudentsIDs method for the object c1, use the dot operator as in the
following example:
c1.printStudentsIDs();
Remember that since printStudentsIDs is a void method, you must invoke it as a separate
statement.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F062092f6-cd84-46b5-8a9a-916af0ff0401%2F6c1ed035-ecb7-4ed8-aa23-0dc1ada79747%2Fofkxqgm_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Part-1: Implementing the Course class without constructors:
Course
name: String
number: int
instructor: String
students: int[]
getNumberOfStudents(): int
printStudentsIDs(): void
addStudent(id: int): void
removeStudent(id:int): boolean
1. Inside the body of the Course class:
a.
Define the data fields name, number, faculty, and students according to their data types in the UML
diagram. The students array includes IDs of students registered in the course.
For example, the following statement declares the data field name:
String name;
b. Define the method getNumberOfStudents that returns the number of students registered in the course,
which is the size of the students array.
c. Define the void method printStudentsIDs which prints IDs of students (elements of the students array)
on one line space separated and prints a new line afterwards.
d. Define the method addStudent which takes an integer that represents a student ID and add it to the
array students.
e. Define the method removeStudent which takes an integer that represents a student ID, and searches
the students array for the requested ID. If it is found, it must remove it from the array and return true.
If it is not found, it must return false.
Note: you may assume that each student ID appears only once in the array students.
2. In your main method:
a. Declare and create an object of type Course named cl as in the following statement:
Course c1= new Course();
b. Print the values of the object cl data fields (name, number, instructor, and students) and observe the
output. Print the values using full statements as follows:
Course name: ....
Course number: ....
Course instructor: ....
Course students reference variable: ....
Note: In order to access data fields of an object we use the dot operator. For example, in order to print
the value of name of the object c1, we use the following statement:
System.out.println("Course name:" + c1.name);
c. Invoke the method printStudentsIDs to print value of the students array elements. Observe and justify
the output.
Note: in order to invoke the printStudentsIDs method for the object c1, use the dot operator as in the
following example:
c1.printStudentsIDs();
Remember that since printStudentsIDs is a void method, you must invoke it as a separate
statement.
![Part-1: Implementing the Course class without constructors:
Course
name: String
number: int
instructor: String
students: int[]
getNumberOfStudents(): int
printStudentsIDs(): void
addStudent(id: int): void
removeStudent(id:int): boolean
1. Inside the body of the Course class:
a.
Define the data fields name, number, faculty, and students according to their data types in the UML
diagram. The students array includes IDs of students registered in the course.
For example, the following statement declares the data field name:
String name;
b. Define the method getNumberOfStudents that returns the number of students registered in the course,
which is the size of the students array.
c. Define the void method printStudentsIDs which prints IDs of students (elements of the students array)
on one line space separated and prints a new line afterwards.
d. Define the method addStudent which takes an integer that represents a student ID and add it to the
array students.
e. Define the method removeStudent which takes an integer that represents a student ID, and searches
the students array for the requested ID. If it is found, it must remove it from the array and return true.
If it is not found, it must return false.
Note: you may assume that each student ID appears only once in the array students.
2. In your main method:
a. Declare and create an object of type Course named cl as in the following statement:
Course c1= new Course();
b. Print the values of the object cl data fields (name, number, instructor, and students) and observe the
output. Print the values using full statements as follows:
Course name: ....
Course number: ....
Course instructor: ....
Course students reference variable: ....
Note: In order to access data fields of an object we use the dot operator. For example, in order to print
the value of name of the object c1, we use the following statement:
System.out.println("Course name:" + c1.name);
c. Invoke the method printStudentsIDs to print value of the students array elements. Observe and justify
the output.
Note: in order to invoke the printStudentsIDs method for the object c1, use the dot operator as in the
following example:
c1.printStudentsIDs();
Remember that since printStudentsIDs is a void method, you must invoke it as a separate
statement.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F062092f6-cd84-46b5-8a9a-916af0ff0401%2F6c1ed035-ecb7-4ed8-aa23-0dc1ada79747%2Ftocw72_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Part-1: Implementing the Course class without constructors:
Course
name: String
number: int
instructor: String
students: int[]
getNumberOfStudents(): int
printStudentsIDs(): void
addStudent(id: int): void
removeStudent(id:int): boolean
1. Inside the body of the Course class:
a.
Define the data fields name, number, faculty, and students according to their data types in the UML
diagram. The students array includes IDs of students registered in the course.
For example, the following statement declares the data field name:
String name;
b. Define the method getNumberOfStudents that returns the number of students registered in the course,
which is the size of the students array.
c. Define the void method printStudentsIDs which prints IDs of students (elements of the students array)
on one line space separated and prints a new line afterwards.
d. Define the method addStudent which takes an integer that represents a student ID and add it to the
array students.
e. Define the method removeStudent which takes an integer that represents a student ID, and searches
the students array for the requested ID. If it is found, it must remove it from the array and return true.
If it is not found, it must return false.
Note: you may assume that each student ID appears only once in the array students.
2. In your main method:
a. Declare and create an object of type Course named cl as in the following statement:
Course c1= new Course();
b. Print the values of the object cl data fields (name, number, instructor, and students) and observe the
output. Print the values using full statements as follows:
Course name: ....
Course number: ....
Course instructor: ....
Course students reference variable: ....
Note: In order to access data fields of an object we use the dot operator. For example, in order to print
the value of name of the object c1, we use the following statement:
System.out.println("Course name:" + c1.name);
c. Invoke the method printStudentsIDs to print value of the students array elements. Observe and justify
the output.
Note: in order to invoke the printStudentsIDs method for the object c1, use the dot operator as in the
following example:
c1.printStudentsIDs();
Remember that since printStudentsIDs is a void method, you must invoke it as a separate
statement.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 4 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
Please help me complete the solution ?
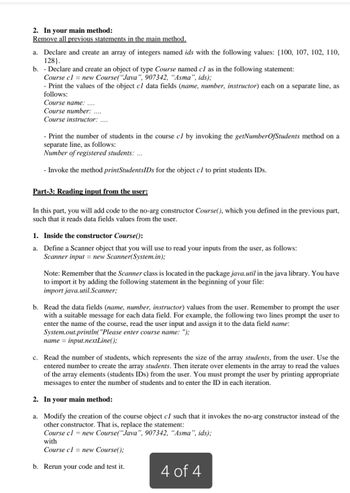
Transcribed Image Text:2. In your main method:
Remove all previous statements in the main method.
a. Declare and create an array of integers named ids with the following values: {100, 107, 102, 110,
128}.
b.
- Declare and create an object of type Course named cl as in the following statement:
Course c1= new Course("Java", 907342, "Asma", ids);
- Print the values of the object cl data fields (name, number, instructor) each on a separate line, as
follows:
Course name: ....
Course number: ....
Course instructor:
- Print the number of students in the course c1 by invoking the getNumberOfStudents method on a
separate line, as follows:
Number of registered students:...
Invoke the method printStudentsIDs for the object cl to print students IDs.
Part-3: Reading input from the user:
In this part, you will add code to the no-arg constructor Course(), which you defined in the previous part,
such that it reads data fields values from the user.
1. Inside the constructor Course():
a. Define a Scanner object that you will use to read your inputs from the user, as follows:
Scanner input = new Scanner(System.in);
Note: Remember that the Scanner class is located in the package java.util in the java library. You have
to import it by adding the following statement in the beginning of your file:
import java.util.Scanner;
b. Read the data fields (name, number, instructor) values from the user. Remember to prompt the user
with a suitable message for each data field. For example, the following two lines prompt the user to
enter the name of the course, read the user input and assign it to the data field name:
System.out.println("Please enter course name: ");
name = input.nextLine();
c. Read the number of students, which represents the size of the array students, from the user. Use the
entered number to create the array students. Then iterate over elements in the array to read the values
of the array elements (students IDs) from the user. You must prompt the user by printing appropriate
messages to enter the number of students and to enter the ID in each iteration.
2. In your main method:
a. Modify the creation of the course object cl such that it invokes the no-arg constructor instead of the
other constructor. That is, replace the statement:
Course c1= new Course("Java", 907342, "Asma", ids);
with
Course c1= new Course();
b. Rerun your code and test it.
4 of 4
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
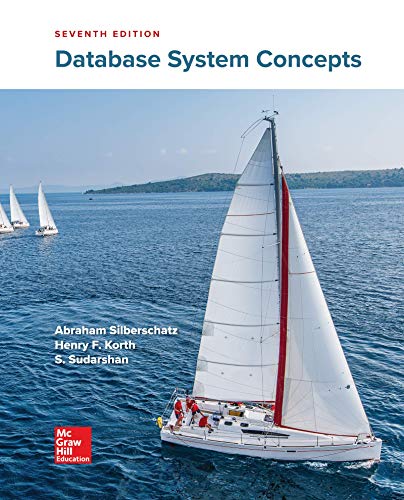
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
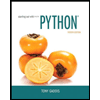
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
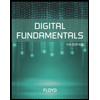
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
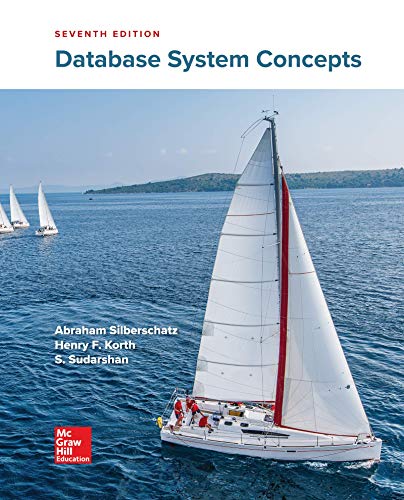
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
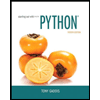
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
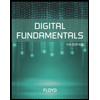
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
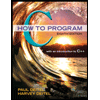
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
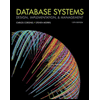
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
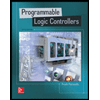
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education