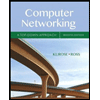
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Please write a python code with 7 different functions in this template
def main():
"""Driver function: calls other functions to perform all tasks."""
lines = get_user_input()
num_words = get_num_words(lines)
num_sentences = get_num_sentences(lines)
avg_words = get_avg_words(num_words, num_sentences)
most_freq_word = get_most_used_word(lines)
print_stats(num_words, num_sentences, avg_words, most_freq_word)
if __name__ == '__main__':
main()
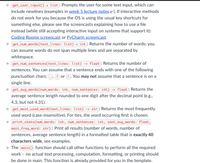
Transcribed Image Text:o get_user_input() → list : Prompts the user for some text input, which can
include newlines (examples in week 5 lecture notes e). If interactive methods
do not work for you because the OS is using the usual key shortcuts for
something else, please see the screencasts explaining how to use a file
instead (while still accepting interactive input on systems that support it):
Coding Rooms screencast or PyCharm screencast.
o get_num_words(text_lines: list) → int : Returns the number of words; you
can assume words do not span multiple lines and are separated by
whitespace.
o get_num_sentences(text_lines: list) -> float : Returns the number of
sentences. You can assume that a sentence ends with one of the following
punctuation chars: ., ? or 9. You may not assume that a sentence is on a
single line.
o get_avg_words(num_words: int, num_sentences: int) -> float : Returns the
average sentence length rounded to one digit after the decimal point (e.g.,
4.3, but not 4.31).
o get_most_used_word(text_lines: list) -> str : Returns the most frequently
used word (case-insensitive). For ties, the word occurring first is chosen.
o print_stats(num_words: int, num_sentences: int, sent_avg_words: float,
most_freq_word: str): Print all results (number of words, number of
sentences, average sentence length) in a formatted table that is exactly 40
characters wide, see examples.
o The main() function should call other functions to perform all the required
work - no actual text processing, computation, formatting, or printing should
be done in main. This function is already provided for you in the template.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Similar questions
- See attached images. Please help - C++arrow_forwardpython function!: a function called popular_color with one parameter, of type dict[str, str] of names and favorite colors. The return type is a str and it is the most commonly occuring color. If two colors are tied for popularity, the first color shown will be picked.arrow_forwardWhich of the following statements is NOT correct? Group of answer choices C++ language provides a set of C++ class templates to implement common data structures, which is known as Standard Template Library (STL); In STL, a map is used to store a collection of entries that consists of keys and their values. Keys must be unique, and values need not be unique; In STL, a set is used to store a collection of elements and it does not allow duplicates; Both set and map class templates in STL are implemented with arrays.arrow_forward
- Alert: Don't submit AI generated answer and please submit a step by step solution and detail explanation for each steps. Write a python program using functionsarrow_forwardPrompt: In Python language, write a function that applies the logistic sigmoid function to all elements of a NumPy array. Code: import numpy as np import math import matplotlib.pyplot as plt import pandas as pd def sigmoid(inputArray): modifiedArray = np.zeros(len(inputArray)) #YOUR CODE HERE: return(modifiedArray) def test(): inputs = np.arange(-100, 100, 0.5) outputs = sigmoid(inputs) plt.figure(1) plt.plot(inputs) plt.title('Input') plt.xlabel('Index') plt.ylabel('Value') plt.show() plt.figure(2) plt.plot(outputs,'Black') plt.title('Output') plt.xlabel('Index') plt.ylabel('Value') plt.show() test()arrow_forwardPlease complete the following guidelines and hints. Using C language. Please use this template: #include <stdio.h>#define MAX 100struct cg { // structure to hold x and y coordinates and massfloat x, y, mass;}masses[MAX];int readin(void){/* Write this function to read in the datainto the array massesnote that this function should return the number ofmasses read in from the file */}void computecg(int n_masses){/* Write this function to compute the C of Gand print the result */}int main(void){int number;if((number = readin()) > 0)computecg(number);return 0;}Testing your workTypical Input from keyboard:40 0 10 1 11 0 11 1 1Typical Output to screen:CoG coordinates are: x = 0.50 y = 0.50arrow_forward
- In C++ Assume that a 5 element array of type string named boroughs has been declared and initialized. Write the code necessary to switch (exchange) the values of the first and last elements of the array.arrow_forwardwrite in html and scriptarrow_forwardQ/Complete the following code in Python language (biometrics) for voice recognition and apply the code, mentioning the approved source if it exists import osimport numpy as npfrom pyAudioAnalysis import audioBasicIO, audioFeatureExtraction, audioTrainTestfrom pydub import AudioSegment# Function to capture and save voice samplesdef capture_voice_samples(num_samples, speaker_name):os.makedirs("speakers", exist_ok=True)os.makedirs(f"speakers/{speaker_name}", exist_ok=True)for i in range(num_samples):input(f"Press Enter and start speaking for sample {i + 1}...")# Recording audio using pyAudioAnalysisaudio = audioBasicIO.record_audio(4, 44100)filepath = f"speakers/{speaker_name}/sample_{i + 1}.wav"audioBasicIO.write_audio_file(filepath, audio, 44100)print(f"Sample {i + 1} saved for {speaker_name}")# Function to extract features from voice samplesdef extract_features():speakers = [d for d in os.listdir("speakers") if os.path.isdir(os.path.join("speakers", d))]all_features = []all_labels =…arrow_forward
- C++ Language Please add an execution chart for this code like the example below. I have provided the code and the example execution chart. : JUST NEED EXECUTION CHARTTT. Thanks Sample Execution Chart Template//your header files// constant size of the array// declare an array of BankAccount objects called accountsArray of size =SIZE// comments for other declarations with variable names etc and their functionality// function prototypes// method to fill array from file, details of input and output values and purpose of functionvoid fillArray (ifstream &input,BankAccount accountsArray[]);// method to find the largest account using balance as keyint largest(BankAccount accountsArray[]);// method to find the smallest account using balance as keyint smallest(BankAccount accountsArray[]);// method to display all elements of the accounts arrayvoid printArray(BankAccount accountsArray[]);int main() {// function calls come here:// give the function call and a comment about the purpose,//…arrow_forwardQ_8. Decription:- Given an array of integers, write a PHP function to find the maximum element in the array. PHP Function Signature: phpCopy code function findMaxElement($arr) { // Your code here } Example: Input: [10, 4, 56, 32, 7] Output: 56 You can now implement the findMaxElement function to solve this problems. .arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
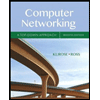
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
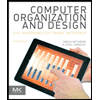
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
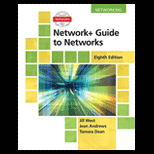
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
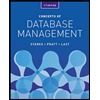
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
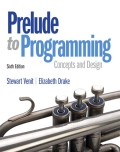
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
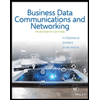
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY