Repeat the example below but this time you should do the following : - Maintain a database in a file: - Add any new vehicle to the fleet. Vehicle information should include -Model year -Make Model name -License plate number -Rent a vehicle to the registered customer -Receive rented vehicle back from customer #include #include #include typedef struct { int id; char name[50]; char phone[15]; /* data */ } Customer; void addCustomer(Customer *arr, int size); void searchCustomer(Customer *arr, int size); int main() { Customer arr[10]; int size = 0; addCustomer(arr, size++); addCustomer(arr, size++); searchCustomer(arr, size); } void addCustomer(Customer *arr, int size) { int id; char name[50]; char phone[15]; printf("Enter Customer ID\n"); scanf("%d", &arr[size].id); printf("Enter name\n"); scanf("%s", arr[size].name); printf("Enter phone\n"); scanf("%s", arr[size].phone); } void searchCustomer(Customer *arr, int size) { char key[50]; int keyID; printf("Enter 1 for Search by ID\nEnter 2 for Search by Name/PhoneNumber\n"); int ch; scanf("%d", &ch); if (ch == 1) { printf("Enter Customer ID to Search\n"); scanf("%d", &keyID); } else { printf("Enter Customer Name or Customer Number to Search\n"); scanf("%s", key); } for (int i = 0; i < size; i++) { if (ch == 2 && strcmp(arr[i].name, key) == 0 || strcmp(arr[i].phone, key) == 0) { printf("ID is %d \nName is %s\nPhone NUmber is %s", arr[i].id, arr[i].name, arr[i].phone); return; } else if (ch == 1) { if (keyID == arr[i].id) { printf("ID is %d \nName is %s\nPhone NUmber is %s", arr[i].id, arr[i].name, arr[i].phone); return; } } } }
Repeat the example below but this time you should do the following :
- Maintain a
- Add any new vehicle to the fleet. Vehicle information should include
-Model year
-Make Model name
-License plate number
-Rent a vehicle to the registered customer
-Receive rented vehicle back from customer
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
typedef struct
{
int id;
char name[50];
char phone[15];
/* data */
} Customer;
void addCustomer(Customer *arr, int size);
void searchCustomer(Customer *arr, int size);
int main()
{
Customer arr[10];
int size = 0;
addCustomer(arr, size++);
addCustomer(arr, size++);
searchCustomer(arr, size);
}
void addCustomer(Customer *arr, int size)
{
int id;
char name[50];
char phone[15];
printf("Enter Customer ID\n");
scanf("%d", &arr[size].id);
printf("Enter name\n");
scanf("%s", arr[size].name);
printf("Enter phone\n");
scanf("%s", arr[size].phone);
}
void searchCustomer(Customer *arr, int size)
{
char key[50];
int keyID;
printf("Enter 1 for Search by ID\nEnter 2 for Search by Name/PhoneNumber\n");
int ch;
scanf("%d", &ch);
if (ch == 1)
{
printf("Enter Customer ID to Search\n");
scanf("%d", &keyID);
}
else
{
printf("Enter Customer Name or Customer Number to Search\n");
scanf("%s", key);
}
for (int i = 0; i < size; i++)
{
if (ch == 2 && strcmp(arr[i].name, key) == 0 || strcmp(arr[i].phone, key) == 0)
{
printf("ID is %d \nName is %s\nPhone NUmber is %s", arr[i].id, arr[i].name, arr[i].phone);
return;
}
else if (ch == 1)
{
if (keyID == arr[i].id)
{
printf("ID is %d \nName is %s\nPhone NUmber is %s", arr[i].id, arr[i].name, arr[i].phone);
return;
}
}
}
}
Note: code with comments and output screenshot is must

Step by step
Solved in 3 steps with 1 images

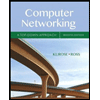
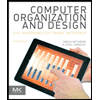
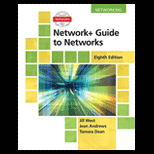
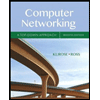
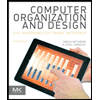
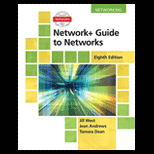
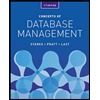
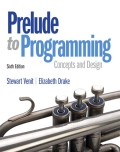
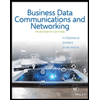