Requirements: Use Java Programming only. Show screenshots of your work. 5. Write a for (or while) statement to calculate the factorial of n, which is defined as n! = n*(n1)*...*2*1. For instance, 5! = 5*4*3*2*1 = 120. Aassign the result to an int type variable named factorial. Assume that factorial and int type variable n have been declared and n has been initialized. (Hint: You may need to initialize the factorial to be one first) 6. Write method public static int linearSearch(int [] arr, ine key) which returns the largest index such that arr[index] matches the key. If there is match, returns -1. 7. Write method public static double average(int [] arr) which returns the average of all elements in given array arr. Assume that arr has length at least 1. 8. Write method public void crazyPrint(String sentence, int n) which print out the given sentence n times. Assume n is positive integer. 9. Write method public static int sum(int n) that take a positive integer n as input and return the sum of 1+2+...+n 10. Write method public static int binarySearch(int[] arr, int target) that return an index of one match of target in arr. If there is no match, return -1. 11. Write a nested for loop statement to print out the multiplication table for numbers 1, 2, .., 9. The output should looks like: 1 x 1 = 1, 1 x 2 = 2, 1 x 3 = 3, 1 x 4 = 4, 1 x 5 = 5, 1 x 6 = 6, 1 x 7 = 7, 1 x 8 = 8, 1 x 9 = 9 2 x 2 = 4, 2 x 3 = 6, .................................................................................., 2 x 9 = 18 3 x 3 = 9, .................................................................................., 3 x 9 = 27 .................................................................................................... 9 x 9 = 81 (Hint: Use System.out.printf to get columns alignment nice and neat) 12. Write a method public static initializeArray(int [] arr) to initialize a given int array named arr with random numbers between 101 and 200, inclusive. 13. Suppose that int [][] triangle = new int[3][]; Write several statements to initialize triangle[0] as {1, 2}; triangle[1] as {3, 4, 5}; and triangle[2] = {6, 7, 8, 9}; 14. Write public static int[] sums(int [][] arr) that returns an integer array, in which ith element is the sum of ith row of table arr. 15. Write public static boolean zeroDiagonal(int [][] arr) which returns true if the diagonal elements in table arr are all zeros.
Addition of Two Numbers
Adding two numbers in programming is essentially the same as adding two numbers in general arithmetic. A significant difference is that in programming, you need to pay attention to the data type of the variable that will hold the sum of two numbers.
C++
C++ is a general-purpose hybrid language, which supports both OOPs and procedural language designed and developed by Bjarne Stroustrup. It began in 1979 as “C with Classes” at Bell Labs and first appeared in the year 1985 as C++. It is the superset of C programming language, because it uses most of the C code syntax. Due to its hybrid functionality, it used to develop embedded systems, operating systems, web browser, GUI and video games.
Requirements:
- Use Java Programming only.
- Show screenshots of your work.
5. Write a for (or while) statement to calculate the factorial of n, which is defined as n! = n*(n1)*...*2*1. For instance, 5! = 5*4*3*2*1 = 120. Aassign the result to an int type variable named factorial. Assume that factorial and int type variable n have been declared and n has been initialized. (Hint: You may need to initialize the factorial to be one first)
6. Write method public static int linearSearch(int [] arr, ine key) which returns the largest index such that arr[index] matches the key. If there is match, returns -1.
7. Write method public static double average(int [] arr) which returns the average of all elements in given array arr. Assume that arr has length at least 1.
8. Write method public void crazyPrint(String sentence, int n) which print out the given sentence n times. Assume n is positive integer.
9. Write method public static int sum(int n) that take a positive integer n as input and return the sum of 1+2+...+n
10. Write method public static int binarySearch(int[] arr, int target) that return an index of one match of target in arr. If there is no match, return -1.
11. Write a nested for loop statement to print out the multiplication table for numbers 1, 2, .., 9. The output should looks like: 1 x 1 = 1, 1 x 2 = 2, 1 x 3 = 3, 1 x 4 = 4, 1 x 5 = 5, 1 x 6 = 6, 1 x 7 = 7, 1 x 8 = 8, 1 x 9 = 9 2 x 2 = 4, 2 x 3 = 6, .................................................................................., 2 x 9 = 18 3 x 3 = 9, .................................................................................., 3 x 9 = 27 .................................................................................................... 9 x 9 = 81 (Hint: Use System.out.printf to get columns alignment nice and neat)
12. Write a method public static initializeArray(int [] arr) to initialize a given int array named arr with random numbers between 101 and 200, inclusive.
13. Suppose that int [][] triangle = new int[3][]; Write several statements to initialize triangle[0] as {1, 2}; triangle[1] as {3, 4, 5}; and triangle[2] = {6, 7, 8, 9};
14. Write public static int[] sums(int [][] arr) that returns an integer array, in which ith element is the sum of ith row of table arr.
15. Write public static boolean zeroDiagonal(int [][] arr) which returns true if the diagonal elements in table arr are all zeros.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

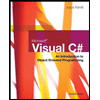
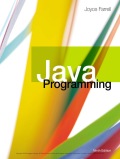
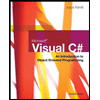
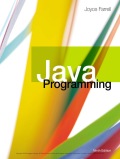