import java.util.StringJoiner; public class preLabC { public static MathVector myMethod(int[] testValues) { // Create an object of type "MathVector". I // return the Math Vector Object back to the testing script. return VectorObject; } } Comments ▾ Description The MathVector.java file contains a class (MathVector). This class allows you to create objects in your program that can contain vectors (a 1D array) and perform actions on those vectors. This is useful for doing math operations like you would in your linear algebra class. Your method should evaluate against two tests. What do you need to do in this exercise? You need to create an "instance" of the MathVector object, VectorObject, in your method. If you do this correctly, your method will accept a 1D array of integers, testValues, feed it into the VectorObject and then return the VectorObject. If you look in the file MathVector.java you'll see that this is one way in which MathVector objects can be created (constructed): 24- 25 26 27 28- 29 30 31- * Creates a MathVector instance backed by the given array. * * @param source the array to use */ public MathVector(int[] source) { 2 this.array source;
import java.util.StringJoiner; public class preLabC { public static MathVector myMethod(int[] testValues) { // Create an object of type "MathVector". I // return the Math Vector Object back to the testing script. return VectorObject; } } Comments ▾ Description The MathVector.java file contains a class (MathVector). This class allows you to create objects in your program that can contain vectors (a 1D array) and perform actions on those vectors. This is useful for doing math operations like you would in your linear algebra class. Your method should evaluate against two tests. What do you need to do in this exercise? You need to create an "instance" of the MathVector object, VectorObject, in your method. If you do this correctly, your method will accept a 1D array of integers, testValues, feed it into the VectorObject and then return the VectorObject. If you look in the file MathVector.java you'll see that this is one way in which MathVector objects can be created (constructed): 24- 25 26 27 28- 29 30 31- * Creates a MathVector instance backed by the given array. * * @param source the array to use */ public MathVector(int[] source) { 2 this.array source;
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![1 import java.util.Random;
2
3
4
5
6-
7
8
9
10
11
12
▼
import java.util.StringJoiner;
public class preLabC {
public static MathVector myMethod(int[] testValues) {
// Create an object of type "MathVector".
// return the Math Vector Object back to the testing script.
return VectorObject;
13
14
15 }
}
▸ Comments
Description
The MathVector.java file contains a class (Math Vector). This class allows you to create objects in your program that
can contain vectors (a 1D array) and perform actions on those vectors. This is useful for doing math operations like
you would in your linear algebra class.
Your method should evaluate against two tests.
What do you need to do in this exercise? You need to create an "instance" of the MathVector object, VectorObject,
in your method. If you do this correctly, your method will accept a 1D array of integers, testValues, feed it into the
VectorObject and then return the VectorObject.
If you look in the file MathVector.java you'll see that this is one way in which MathVector objects can be created
(constructed):
24
25
26
27
28
29 ▼
30
31 -
32
/**
* Creates a MathVector instance backed by the given array.
*
* @param source the array to use
*/
public MathVector(int[] source) {
this.array = source;
}](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fcfd79b32-4de5-49e8-861a-3fd719d02c63%2F8885cd42-2469-4ad1-a2f5-7f6a28c3d998%2Fz56n3nt_processed.png&w=3840&q=75)
Transcribed Image Text:1 import java.util.Random;
2
3
4
5
6-
7
8
9
10
11
12
▼
import java.util.StringJoiner;
public class preLabC {
public static MathVector myMethod(int[] testValues) {
// Create an object of type "MathVector".
// return the Math Vector Object back to the testing script.
return VectorObject;
13
14
15 }
}
▸ Comments
Description
The MathVector.java file contains a class (Math Vector). This class allows you to create objects in your program that
can contain vectors (a 1D array) and perform actions on those vectors. This is useful for doing math operations like
you would in your linear algebra class.
Your method should evaluate against two tests.
What do you need to do in this exercise? You need to create an "instance" of the MathVector object, VectorObject,
in your method. If you do this correctly, your method will accept a 1D array of integers, testValues, feed it into the
VectorObject and then return the VectorObject.
If you look in the file MathVector.java you'll see that this is one way in which MathVector objects can be created
(constructed):
24
25
26
27
28
29 ▼
30
31 -
32
/**
* Creates a MathVector instance backed by the given array.
*
* @param source the array to use
*/
public MathVector(int[] source) {
this.array = source;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
I got another question. What would you do for this?
![preLabC.java
1 import java.util.Random;
2 import java.util.StringJoiner;
3
4- public class preLabC {
5
6-
7
8
9
10
11
12
13
14
15
16
17
18
19
20 }
public static String myMethod(MathVector inputVector) {
String vectorStringValue = new String(); // empty String object
System.out.println("here is the contents object, inputVector: + inputVector);
// get a String out of that MathVector object. Do it here. On the next line.
// return the double value here.
return vectorStringValue;
"1
▸ Compilation
Description
A MathVector object will be passed to your method. Return its contents as a String.
If you look in the file MathVector.java you'll see there is a way to output the contents of a MathVector
object as a String. This makes it useful for displaying to the user.
/**
* Returns a String representation of this vector. The String should be in the format "[1, 2, 3]"
*
* @return a String representation of this vector
* @apiNote **DO NOT** use the built-in (@code Arrays.toString()} method.
*/
@Override
public String toString() {
var sj = new StringJoiner(",", "[", "]");
for (var e: array) {
sj.add(String.value0f(e));
}
return sj.toString();
You might have noticed that there's an @override term there. That's because many objects already
have a "toString()" method associated with them... because Java was designed to include them by
default. Here, the override tells Java "I know, I know. You already have a toString() that you'd assign
here. But it's not good enough. Here's a better one for this particular kind of object." It's a little bit
like saying "Most humans have two legs. So, by default, I'll give everyone two legs. But sometimes we
override that and give no legs, or just one leg to a person. And sometimes we give them four so that
they can be a centaur!"
To use this in a println() method, just name your object. The toString() method will
be called implicitly. Or you can just write System.out.println("look! " +
myObject.toString());](https://content.bartleby.com/qna-images/question/cfd79b32-4de5-49e8-861a-3fd719d02c63/a6e99a85-ecb1-4bcb-97cb-991ddd746f2d/0jv0ihe_thumbnail.png)
Transcribed Image Text:preLabC.java
1 import java.util.Random;
2 import java.util.StringJoiner;
3
4- public class preLabC {
5
6-
7
8
9
10
11
12
13
14
15
16
17
18
19
20 }
public static String myMethod(MathVector inputVector) {
String vectorStringValue = new String(); // empty String object
System.out.println("here is the contents object, inputVector: + inputVector);
// get a String out of that MathVector object. Do it here. On the next line.
// return the double value here.
return vectorStringValue;
"1
▸ Compilation
Description
A MathVector object will be passed to your method. Return its contents as a String.
If you look in the file MathVector.java you'll see there is a way to output the contents of a MathVector
object as a String. This makes it useful for displaying to the user.
/**
* Returns a String representation of this vector. The String should be in the format "[1, 2, 3]"
*
* @return a String representation of this vector
* @apiNote **DO NOT** use the built-in (@code Arrays.toString()} method.
*/
@Override
public String toString() {
var sj = new StringJoiner(",", "[", "]");
for (var e: array) {
sj.add(String.value0f(e));
}
return sj.toString();
You might have noticed that there's an @override term there. That's because many objects already
have a "toString()" method associated with them... because Java was designed to include them by
default. Here, the override tells Java "I know, I know. You already have a toString() that you'd assign
here. But it's not good enough. Here's a better one for this particular kind of object." It's a little bit
like saying "Most humans have two legs. So, by default, I'll give everyone two legs. But sometimes we
override that and give no legs, or just one leg to a person. And sometimes we give them four so that
they can be a centaur!"
To use this in a println() method, just name your object. The toString() method will
be called implicitly. Or you can just write System.out.println("look! " +
myObject.toString());
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
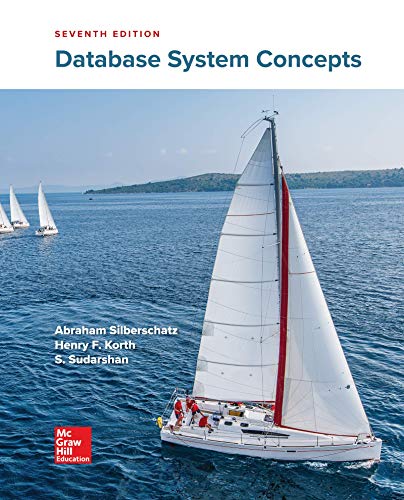
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
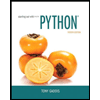
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
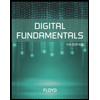
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
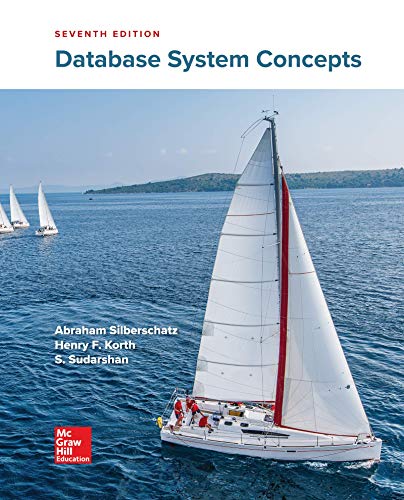
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
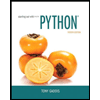
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
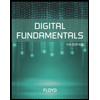
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
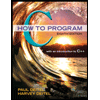
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
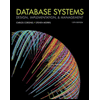
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
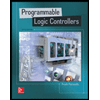
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education