Input Format The first line of the input is an integer T denoting the number of lines that will follow. Each line then consists of a variable number of elements separated by a space. The line starts with a word op denoting what operation to do. The operations will only be any of the following: add, sub, neg, mul_s, mul.The next elements will be space-separated numbers. For add, sub, and mul, op will be followed by six numbers az ay az bz by bz corresponding to the coordinates of vectors a and b respectively., For mul_s, op will be followed by four numbers az ay a, s corresponding to the coordinates of the vector a and a scalar s. respectively. For neg, op will be followed by three numbers a, a, az corresponding to the coordinates of the vector a. Constraints Input Constraints T< 100 A = (az, ay, az) € z³, {a; € Z: [a;|<10°} op € {add, sub, neg, mul, mul_s} Max running time of code should be s5 seconds for each test input. You can assume that all of the inputs are well-formed and are always provided within these constraints. You are not required to handle any errors. Functional Constraints You are required to create a class named Vector3D with an -init_(self, x, y, z) function. It should also have the following special functions: -_add__, -_sub__, __neg, -_mul_. Failure to do so will mark your code with a score of zero. Output Format The output consists of T lines, with each line i corresponding to the 3D vector aa aiy aiz that is the result of performing the operation requested on that line. Sample Input 0 5 add 1 2 3 4 sub 1 2 3 4 5 6 mul_s 1 2 3 -2 mul 1 2 3 45 6 neg 3 3 3 Sample Output 0 579 -3 -3 -3 -2 -4 -6 4 10 18 -3 -3 -3
class Vector3D:
def __init__(self, x, y, z):
# TODO: Create a routine that saves the vector
# <x, y, z> into this Vector3D object.
pass
def __add__(self, other):
# TODO: Create a routine that adds this vector with Vector3D other
# and returns the result as a Vector3D object
return None
def __neg__(self):
# TODO: Create a routine that returns the negative (opposite) of
# this vector as a Vector3D object
return None
def __sub__(self, other):
# TODO: Create a routine that subtracts this vector with Vector3D other
# and returns the result as a Vector3D object
return None
def __mul__(self, other):
# TODO: Create a routine that multiplies this vector with other
# depending on its data type, and returns the result as a
# Vector3D object. other can either be an integer
# scalar or a Vector3D object.
return None
def main():
testcases = int(input())
for t in range(testcases):
line_in = input().split()
op = line_in[0].strip()
vec_vals = [int(x) for x in line_in[1:]]
# TODO: a Write routine that processes a line in the input
# op - string
# - operation to do with the provided
# - can only be one from the set: {add, sub, neg, mul_s, mul}
# vec_vals - list of integers
# - numbers that follow the op in the input line
# - can only have a length of 3, 4, or 6
if __name__ == '__main__':
main()
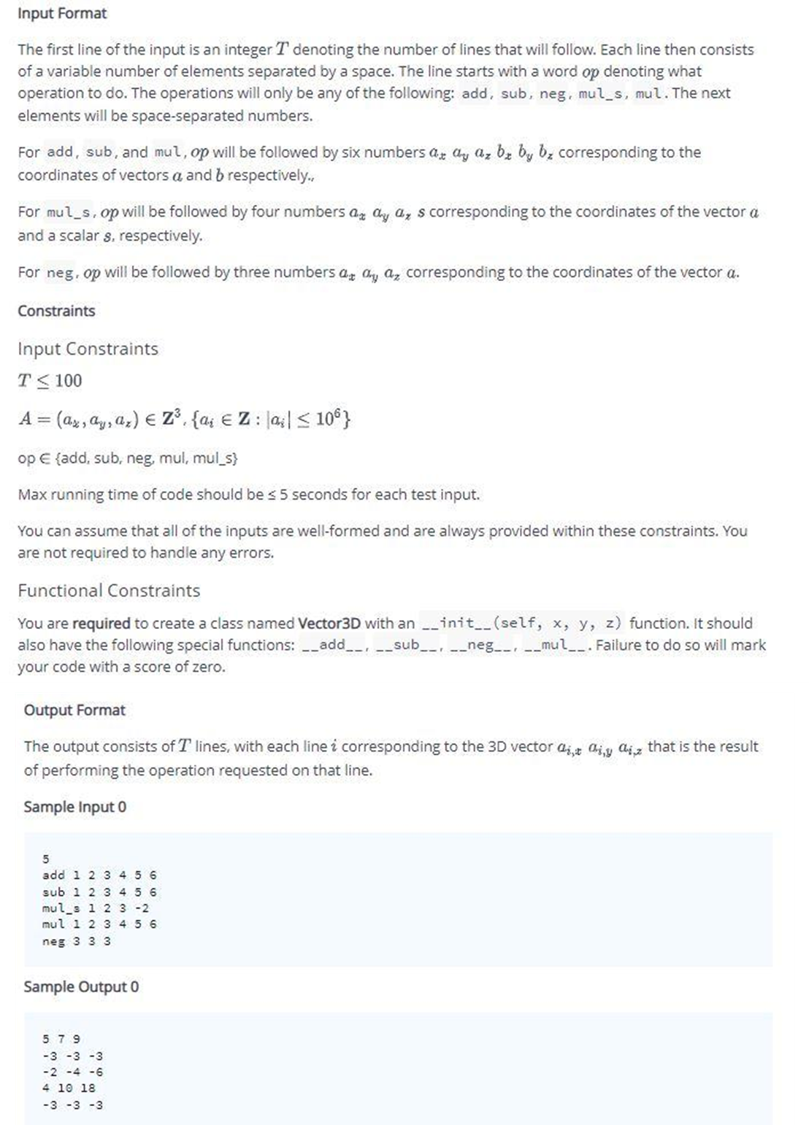
![1
3 class Vector3D:
def init _(self, x, y, z):
# TODO: Create a routine that saves the vector
4
6.
<x, y, z> into this Vector3D object.
pass
8
def add_(self, other):
# TODO: Create a routine that adds this vector with Vector3D other
and returns the result as a Vector3D object
10
11
%23
12
13
return None
14
15
def -neg__(self):
16
= TODO: Create a routine that returns the negative (opposite) of
17
%23
this vector as a Vector3D object
18
19
return None
20
def -_sub__(self, other):
# TODO: Create a routine that subtracts this vector with Vector3D other
%23
21
22
23
and returns the result as a Vector3D object
24
25
return None
26
27 Y
def mul_(self, other):
# TODO: Create a routine that multiplies this vector with other
28
29
%23
depending on its data type, and returns the result as a
Vector3D object. other can either be an integer
scalar or a Vector3D object.
30
%23
31
32
33
return None
34
35 vdef main():
36
testcases = int(input ())
37
for t in range (testcases) :
line in = input().split()
op = line_in [0].strip()
vec vals [int (x) for x in line in[1:])
38
39
40
41
42
43
# TODO: a Write routine that processes a line in the input
- string
- operation to do with the provided vectors
- can only be one from the set: {add, sub, neg, mul_s, mul}
44
# op
45
%23
46
47
# vec vals - list of integers
- numbers that follow the op in the input line
- can only have a length of 3, 4, or 6
48
%3D
49
%23
50
51 vif -_name_ == '-_main__':
main()
52](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa3cf655d-3bdc-4013-8c1f-5d6d56ea7228%2F219f4d51-9c6a-46f2-a124-034978de950c%2F3iuj9rc_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

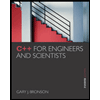
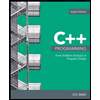
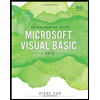
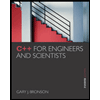
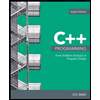
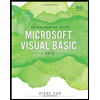
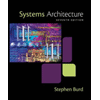
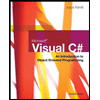