Returns the area of the largest rectangle in . The area of a rectangle is defined by number of 1's that it contains.
Q: Suppose the following array were passed in to a mergesort algorithm: 42 39 87 21| 56 93 43 35 Draw…
A: I have applied the merge sort algorithm in the array and I have show how our array will be…
Q: Read the problem below. If ever there will be codes in the answer, write the code in Python. Even…
A: Insertion sort does not use an extra array to give the final sorted elements whereas in the case of…
Q: Your task is sorting the given list by dictionary order, sorting operation must be realized using…
A: The code is given below. It is well-commented.
Q: The algorithm SkipListSort is a sorting algorithm that begins by inserting a sequence of keys into a…
A: Given: The algorithm SkipListSort is a sorting algorithm that begins by inserting a sequence of…
Q: implement quicksort in SML with these requirements type (''a * ''a ->bool) -> ''alist -> ''a list…
A: Like Merge Sort, QuickSort is a Divide and Conquer set of rules. It selections an detail as pivot…
Q: Count overlapping disks def count_overlapping_disks(disks): Right on the heels of the previous…
A: Actually, python is a easiest programming language. It is a dynamically typed programming language.
Q: Q1: given N numbers in pl,p2,p3...pn, we would like to sort these number. The only process we can do…
A: C++ implementation is given below
Q: PYTHON Suppose that I have a list of lists: a = [ [25, 2, 5, 6, 8], [20,10,3,0,4], [2,5,9,2,2] ] I…
A: The program is implemented in Python using structured approach. In program we have a 2d list. The…
Q: EXPLAIN THIS PYTHON CODE STEP BY STEP WITH LOGICS Q.Create a doubly linked list that performs the…
A: This is the format of the linked list . It consist of nodes connected to each other .Each node…
Q: Python) Write a function that takes in a list (L) as input, and returns the number of inversions. An…
A: Ans : Code: def inversions(arr): n = len(arr) inv_count = 0 for i in range(n): for j…
Q: Some said the best initial gap size is the floor of the half of the length of the list. Explain why.…
A: Shell sorting algorithm is an extension of insertion sort. It has improved the average time…
Q: Write a python program to implement Breadth first search Traversal.
A: Introduction: Here we are required to create a program in python to implement Breadth first search…
Q: he requirement for methods are: def check_prime(p): #checks if the number p in the given list is…
A: A prime number is a positive number which has only 2 factors i.e. 1 and itself this means the only…
Q: The function below implements Quicksort. void Quicksort(int first, int last) { int i= first+1, j =…
A: It is based on divide-and-conquer technique. It chooses one element as pivot. The pivot element is…
Q: Consider the QuickSelect. This algorithm solves the problem of finding k-th smallest value in a…
A: Quickselect is a selection algorithm to find the k-th smallest element in an unordered list. It is…
Q: P follows: Every positive integer greater than 1 can be written uniquely as a prime or as the…
A: According to the information given:- We have to 1. First declare the variable - iterate = 2 and…
Q: A run is a sequence of adjacent repeated values. Write a Python script to generate a sequence of 20…
A: Python code :- import random def run():#create a list of 20 random number in range (1,6)List = []for…
Q: Use the divide-and-conquer approach to write a java code that finds the largest item in a list of n…
A: Code: import java.util.*; import java.lang.*; class LargeElement { public static int…
Q: For a relatively large n, you sort the numbers 1 to n with insertion sort. The numbers are given in…
A: Sorting is the process of arranging the elements in particular order or manner like ascending or…
Q: Consider a doubly-linked list with n elements which are sorted. Which of the following statements…
A: A doubly linked list is a data structure which consists of three parts which represents one data…
Q: (IN C++) Write a program to implement Quicksort. Also implement one of the slow sorts (Bubble,…
A: ANSWER:-
Q: What is the running time of finding a single number from an unsorted array of p elements. (a) O(n)…
A: Running time for searching an element in unsorted array of element n is O(p) Running time for…
Q: Suppose you want to find documents that contain at least k of a given set of n keywords. Suppose…
A: Let us consider the documents that contain at least k of a given set of nkeywords.The keyword index…
Q: Question 1 What are the running times of the following function in the best and worst cases? Assume…
A: In this question, we are given a function sum with size of list 'n'. We are asked the best case and…
Q: Write a divide and conquer algorithm that reverses the order of the elements of a list. def…
A: Below is the code in python and sample output:
Q: Consider the situation when Quicksort is used to sort an array of n elements into ascending order,…
A: The best option to do the comparison of the algorithms would be to express or indicate the running…
Q: solution should have O(l1.length + l2.length) time complexity, since this is what you will be asked…
A: let us see the answer:- Introduction:- The concept is comparable to array merging. However, the new…
Q: What is the (worst case) time complexity of the following find function, which searches for a key in…
A: The recursive relation of given code T(n) = T(n-1) + K = T(n-2) + K +K = T(n-3) + K…
Q: A list/array of size N has integer data. There may be duplicate values in the list. You have at your…
A: - The question is to examine and find the time complexity of the algorithms showed. - We have to…
Q: Write a function that returns of multiples of n in given list. Ex: [12,41,63,21,52,17,18] n=3…
A: def multpiles_of_n(l,n): res=[] # taking empty list for i in l: #iterating over the list…
Q: 1a) write python code for creating a 10 x 20 table (2D list of lists) named t, initialized to all…
A: 1. a. Program: a=[[0]*10]*20for i in a: print(i) Screenshot: Output:
Q: Rewrite the following code in your way so you will have lower complexity. 36 def example5(A, B): #…
A: In this program, I understand that we need to count the number of elements in B which are equal to…
Q: We learnt this week that lists can be multi-dimensional. For e.g., the following is another example…
A: Please find the answer below :
Q: The bubble sort algorithm discussed in class is used to sort the following sequence of integers: 2…
A: Part(a) Number of passes: Given total number of elements in the sequence are 6. Maximum number of…
Q: In Python, A list may contain {0,1,2}, find the number of strings of size n that do not contain two…
A: Algorithm: 1. Create a list of lists 2. Create a list of numbers 3. Call rec(list_of_lists,…
Q: For this exercise, a list of non-negative numbers will be given, for which the following operation…
A: 1. create a list.2. ask user to enter elements.3. iterate till the range of the list.4. append the…
Q: in C Implement the QuickSort algorithm. - For n=3 the algorithm uses the rearrange function with…
A: Quick sort algorithm is a sorting algorithm which uses partition procedure to sort. It is best…
Q: Consider the following C++ code fragment for Selection Sort (the numbers on the left-hand are line…
A: Answer: We have discussed about selection sort in brief explanation
Q: a. A list/array of size N has integer data. There may be duplicate values in the list. You have at…
A: - The question is to determine the time complexities of the parts a and b of the provided problem.…
Q: write a Python function that takes in a list of integers (elements), checks whether the list is…
A: Coded using Python 3.
Q: Write a python program that asks the user to give multiple numbers separated by commas. Your task is…
A: numbers = input("\t input values: ") #input numbers from user seperated by commanum =…
Q: 20. Consider an algorithm intending to store all prime numbers between 2 and 20 (inclusive) in a…
A: (a) if is_prime(2*i): prime_list.append(2*i) i=i+1increment of i is being done inside the if…
Q: Create a python code that takes a list and find the sequence of consecutive numbers where the…
A: Program Explanation: Python 3 program to print the absolute difference of the consecutive even and…
Q: Performing sequential search for an item that is not present in an unsorted list has Best-Case…
A: Since, we know that the item is not present. And also list is unsorted, the key value( the item…
Q: Suppose you compute min & max of the following list of numbers using the divide and conquer…
A: In divide and conquer algorithm, it contains mainly 3 steps, that are: Divide Conquer Combine In…
Q: Write an algorithm for the following to swap two adjacent elements by adjusting only the links (and…
A: Singly-linked lists. Algorithm void SwapTwoAdjacentNodes_Single(Position BeforeP, List L){…
using this code(PYTHON):
def longest_chain(lst: list[int]) -> int:
i = 0
answer = []
while i < len(lst) and lst[i] == 1:
answer.append(lst[i])
i += 1
return sum(answer)
How do I solve these two questions
![def largest_at_position(matrix: list[list[int]], row: int, col: int) -> int:
Returns the area of the largest rectangle whose top left corner is at
position <row>, <col> in <matrix>,
You MUST make use of the helper <longest_chain> here as you loop through
each row of the matrix. Do not modify (i.e., mutate) the input matrix.
>>> case1 = [[1, 0, 1, 0, 0],
[1, 0, 1, 1, 1],
[1, 1, 1, 1, 1],
[1, 0, 0, 1, 0]]
..
>>> largest_at_position(case1, 0, 0)
4
>>> largest_at_position(case1, 2, 0)
5
>>> largest_at_position(case1, 1, 2)
6](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd90ccff3-c80e-49fc-8493-7c939907e572%2F4e4e8f86-6bde-4e3f-9225-6c4ddde8a33e%2F1w4pbjo_processed.png&w=3840&q=75)
![def largest in_matrix(matrix: list[list[int]]) -> int:
II II ||
Returns the area of the largest rectangle in <matrix>.
The area of a rectangle is defined by number of 1's that it contains.
Again, you MUST make use of the helper <largest_at_position> here. If you
managed to code <largest_at_position> correctly, this function should be
simple to implement.
Similarly, do not modify (i.e., mutate) the input matrix.
Precondition:
<matrix> will only contain the integers 1 and 0.
>>> case1 = [[1, 0, 1, 0, 0],
[1, 0, 1, 1, 1],
[1, 1, 1, 1, 1],
[1, 0, 0, 1, 0]]
>>> largest_in_matrix(case1)
6](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd90ccff3-c80e-49fc-8493-7c939907e572%2F4e4e8f86-6bde-4e3f-9225-6c4ddde8a33e%2F56kf3ko_processed.png&w=3840&q=75)

Step by step
Solved in 2 steps

- A4. Code to remove duplicate elements from ArrayList in java? Using Java 8 Stream.distinct() Approach Get the ArrayList with duplicate values. Create a new List from this ArrayList. Using Stream().distinct() method which return distinct object stream. convert this object stream into List /**.Write a Python code using the given function and conditions. Do not use Numpy. Use LinkedList Manipulation. Given function: def insert(self, newElement, index) Pre-condition: The list is not empty. Post-condition: This method inserts newElement at the given index of the list. If an element with the same key as newElement value already exists in the list, then it concludes the key already exists and does not insert the key. [You must also check the validity of the index].Need help only part 2. Thank you! In this assignment, you will create a Linked List data structure variant called a “Circular Linked List”. The Node structure is the same as discussed in the slides and defined as follows (we will use integers for data elements): public class Node { public int data; public Node next; } For the Circular Linked List, its class definition is as follows: public class CircularLinkedList { public int currentSize; public Node current; } In this Circular Linked List (CLL), each node has a reference to an existing next node. When Node elements are added to the CLL, the structure looks like a standard linked list with the last node’s next pointer always pointing to the first. In this way, there is no Node with a “next” pointer in the CLL that is ever pointing to null. For example, if a CLL has elements “5”, “3” and “4” and “current” is pointing to “3”, the CLL should look like: Key observations with this structure:…
- 1-Let the list have a head and a tail. That is, a pointer (have a marker) to both the beginning (first Node) of the list and the last Node. What process does Tail facilitate? 2-insert(int index, int element): adds this element to the index position. For example, if index is 4, it adds this element between index 3 and 4 in the list. The size of the list has increased by one. 3-append(int elem): Adds the element to the end of the list. The size of the list has increased by one. 4-get(int index): Returns the element at the index position of the list, no change in the list. 5-remove(int index): Returns the element at the index position of the list. This element is removed from the list and the list size is reduced by one. 6-findMin(): returns the index of the smallest number in the list. 7-findMax(): returns the index of the largest number in the list. 8-search(int elem): searches elem in the list. It returns -1 when you can't find elem's index when you find it. 9-ToArray(): Return an…1-Let the list have a head and a tail. That is, a pointer (have a marker) to both the beginning (first Node) of the list and the last Node. What process does Tail facilitate? 2-insert(int index, int element): adds this element to the index position. For example, if index is 4, it adds this element between index 3 and 4 in the list. The size of the list has increased by one. 3-append(int elem): Adds the element to the end of the list. The size of the list has increased by one. 4-get(int index): Returns the element at the index position of the list, no change in the list. 5-remove(int index): Returns the element at the index position of the list. This element is removed from the list and the list size is reduced by one. 6-findMin(): returns the index of the smallest number in the list. 7-findMax(): returns the index of the largest number in the list. 8-search(int elem): searches elem in the list. It returns -1 when you can't find elem's index when you find it. 9-ToArray(): Return an…1-Let the list have a head and a tail. That is, a pointer (have a marker) to both the beginning (first Node) of the list and the last Node. What process does Tail facilitate? 2-insert(int index, int element): adds this element to the index position. For example, if index is 4, it adds this element between index 3 and 4 in the list. The size of the list has increased by one. 3-append(int elem): Adds the element to the end of the list. The size of the list has increased by one. 4-get(int index): Returns the element at the index position of the list, no change in the list. 5-remove(int index): Returns the element at the index position of the list. This element is removed from the list and the list size is reduced by one. 6-findMin(): returns the index of the smallest number in the list. 7-findMax(): returns the index of the largest number in the list. 8-search(int elem): searches elem in the list. It returns -1 when you can't find elem's index when you find it. 9-ToArray(): Return an…
- What is the biggest advantage of linked list over array? Group of answer choices Unlike array, linked list can dynamically grow and shrink With linked list, it is faster to access a specific element than with array Linked list is easier to implement than array Unlike array, linked list can only hold a fixed number of elementsWhich of the following statements are true? A. Appending of elements to a dynamic list takes constant time. B. Row-wise traversal of 2-dimensional arrays is commonly faster than column-wise traversal. C. Tranversing an array is faster than traversing a linked list with the same number of entries. D. Node lookup time in a balanced binary search tres is O(1).Create a class Student with the fields: name (a String) and gpa (a double). Create a linked list of Student objects, called students using the LinkedList class from java.util.Sort the linked list students “by length” of name (see Sect. 11.3 in textbook) using the sort method from class Collections.Sort the initial linked list students by gpa using the sort method from class Collections. Apply the toArray() method from class LinkedList to the initial linked list students and sort the obtained array “by length” of name using the sort method from class Arrays. After each sort, print the result.Do NOT use the sort method from the List interface (also available from a LinkedList).
- Question 11 If N represents the number of elements in the collection, then the contains method of the ArrayCollection class is O(1). True False Question 12 With the text's array-based list implementation, the set of stored elements is scattered about the array. True False Question 13 The add method of the LinkedCollection class places the new element at the beginning of the underlying linked list. True False Question 14 If N represents the number of elements in the list, then the index-based add method of the ABList class is O(N). True False Question 15 The attributes of a class that are used to determine equality of objects of the class are called the key attributes. True False Question 16 According to the text's specifications, a collection is a list. True False Question 17 O(1) is the order of growth execution time of the add operation when using the ArrayCollection class, assuming a collection size of N. True False Question 18 When an object of class LBList…Create a Numpy List named as marks with six float numbers, showing the final marks of a student in six subjects. Round the marks from marks array from Q-3 a) up to two decimal numbers What if you need to create the same list as Q-3 a), but for five students. Can you still use 1-D array? How many dimensions should be there? Find the highest marks a student has obtained using marks array from Q-3 a) Use seaborn to show marks array from Q-3 a)simulate Cantor’s diagonalization argument. Using a pool of 5-letter words, build a 5 by 5 matrix in which each row is part of the list you are to compare. You are comparing the word that is extracted from the diagonal and each letter is replaced with the shifted letter.
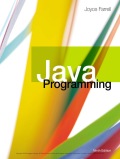
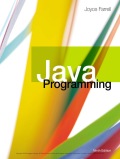