Rewrite the two print statements in the code using format printing to show the result in two decimal places . def resistance(i,e): return e/i #Compute the total resistance as per the given formula def parallel_resistance(r1,r2,r3): return 1.0/((1.0/r1)+(1.0/r2)+(1.0/r3)) #Compute the series resistance def series_resistance(r1,r2,r3): return r1+r2+r3 #Read the voltage e1=float(input('Voltage 1 : ')) e2=float(input('Voltage 2 : ')) e3=float(input('Voltage 3 : ')) #Read the current i1=float(input('Current 1 : ')) i2=float(input('Current 2 : ')) i3=float(input('Current 3 : ')) #Compute the resistance r1=resistance(i1,e1) r2=resistance(i2,e2) r3=resistance(i3,e3) #Compute the resistance in parallel Circuit rp=parallel_resistance(r1,r2,r3) #Compute the resistance in series Circuit rs=series_resistance(r1,r2,r3) #Display the result print('Resistance in Parallel Circuit : {0:.2f}'.format(rp)) print('Resistance in Series Circuit : {0:.2f}'.format(rs))
Rewrite the two print statements in the code using format printing to show the result in two decimal places .
def resistance(i,e):
return e/i
#Compute the total resistance as per the given formula
def parallel_resistance(r1,r2,r3):
return 1.0/((1.0/r1)+(1.0/r2)+(1.0/r3))
#Compute the series resistance
def series_resistance(r1,r2,r3):
return r1+r2+r3
#Read the voltage
e1=float(input('Voltage 1 : '))
e2=float(input('Voltage 2 : '))
e3=float(input('Voltage 3 : '))
#Read the current
i1=float(input('Current 1 : '))
i2=float(input('Current 2 : '))
i3=float(input('Current 3 : '))
#Compute the resistance
r1=resistance(i1,e1)
r2=resistance(i2,e2)
r3=resistance(i3,e3)
#Compute the resistance in parallel Circuit
rp=parallel_resistance(r1,r2,r3)
#Compute the resistance in series Circuit
rs=series_resistance(r1,r2,r3)
#Display the result
print('Resistance in Parallel Circuit : {0:.2f}'.format(rp))
print('Resistance in Series Circuit : {0:.2f}'.format(rs))
- Adding main in code.
def resistance(i,e):
return e/i
#Compute the total resistance as per the given formula
def parallel_resistance(r1,r2,r3):
return 1.0/((1.0/r1)+(1.0/r2)+(1.0/r3))
#Compute the series resistance
def series_resistance(r1,r2,r3):
return r1+r2+r3
def main():
#Read the voltage
e1=float(input('Voltage 1 : '))
e2=float(input('Voltage 2 : '))
e3=float(input('Voltage 3 : '))
#Read the current
i1=float(input('\nCurrent 1 : '))
i2=float(input('Current 2 : '))
i3=float(input('Current 3 : '))
#Compute the resistance
r1=resistance(i1,e1)
r2=resistance(i2,e2)
r3=resistance(i3,e3)
#Compute the resistance in parallel Circuit
rp=parallel_resistance(r1,r2,r3)
#Compute the resistance in series Circuit
rs=series_resistance(r1,r2,r3)
#Display the result
print('\nResistance in Parallel Circuit : {0:.2f}'.format(rp))
print('Resistance in Series Circuit : {0:.2f}'.format(rs))
main()

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

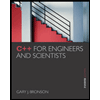
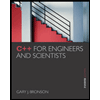