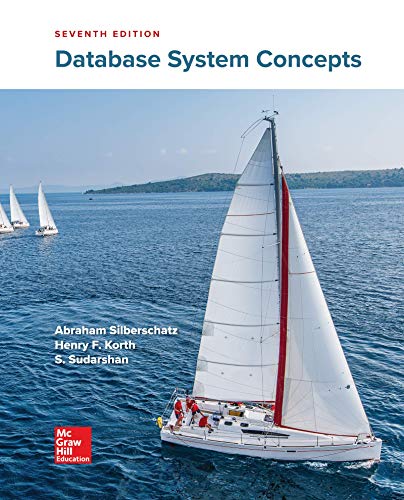
Concept explainers
C
Instructions: Use the code template below to implement the length() and reverse() functions into the main()
/* This program will create a string which is the reverse of an original string using pointers.
* Performs string handling, manipulates pointers, passes pointers to functions.
*/
#include <stdio.h>
#include <stdlib.h>
int length( )
{
while(str1[len++] != '\0'); // Calculate length of string
len--; // Remove the null character
len--; // Array index start from 0 to (length -1)
return ;
}
void reverse( )
{
while (counter < len)
{
i = str1[counter];
str1[counter] = str1[len];
str1[len] = i;
counter++;
len--;
}
return ;
}
int main ()
{
char str1[41]; // String to be Reversed
printf("Enter some text (No spaces and 40 characters max): ");
scanf("%40s", str2);
printf("Reversed string: %s\n", str1);
return 0;
}

Step by stepSolved in 2 steps with 2 images

- C++ Code: You will implement the following functions: /** * Reads from standard input a list of Short Tandem Repeat (STRs) * and their known counts for several individuals * * @param nameSTRs the STRs (eg. AGAT, AATG, TATC) * @param nameIndividuals the names of individuals (eg. Alice, Bob, Charlie) * @param STRcounts the count of the longest consecutive occurrences of each STR in the DNA sequence for each individual * @pre nameSTRs, nameIndividuals, and nameSTRs are empty * @post nameSTRs, nameIndividuals and STRcounts are populated with data read from stdin **/ void readData(vector<string>& nameSTRs, vector<string>& nameIndividuals, vector<vector<int>>& STRcounts) For example, consider the input: 3 AGAT AATG TATC Alice 5 2 8 Bob 3 7 4 Charlie 6 1 5 It shows, in the first line, the number of STRs followed by the names of those STRs, which will be populated into the vector nameSTRs. The remaining lines contain data for a number of individuals. Their…arrow_forwardI need to convert this code from C language to C++arrow_forwardC++ Given code is #pragma once#include <iostream>#include "ourvector.h"using namespace std; ourvector<int> intersect(ourvector<int> &v1, ourvector<int> &v2) { // TO DO: write this function return {};}arrow_forward
- IN C PROGRAMMING LANGUAGE: Please write a pointer version of squeeze() named psqueeze(char *s, char c) which removes c from the string s.arrow_forwardPYTHON Problem Statement Given a list of numbers (nums), for each element in nums, calculate how many numbers in the list are smaller than it. Write a function that does the calculation and returns the result (as a list). For example, if you are given [6,5,4,8], your function should return [2, 1, 0, 3] because there are two numbers less than 6, one number less than 5, zero numbers less than 4, and three numbers less than 8. Sample Input smaller_than_current([6,5,4,8]) Sample Output [2, 1, 0, 3]arrow_forwardC++ The binary search algorithm given in this chapter is nonrecursive. Write and implement a recursive version of the binary search algorithm. The program should prompt Y / y to continue searching and N / n to discontinue. If an item is found in the list display the following message: x found at position y else: x is not in the list Use the following list of integers when testing your program: 2, 6, 8, 13, 25, 33, 39, 42, 53, 58, 61, 68, 71, 84, 97arrow_forward
- Write a function strlen that takes one char str[] as an argument, and return the number of characters in the str[] array. (c++)arrow_forwardIn C++ Assume that a 5 element array of type string named boroughs has been declared and initialized. Write the code necessary to switch (exchange) the values of the first and last elements of the array.arrow_forwardhelp me solve this in c++ please Write a program that asks the user to enter a list of numbers from 1 to 9 in random order, creates and displays the corresponding 3 by 3 square, and determines whether the resulting square is a Lo Shu Magic Square. Notes Create the square by filling the numbers entered from left to right, top to bottom. Input validation - Do not accept numbers outside the range. Do not accept repeats. Must use two-dimensional arrays in the implementation. Functional decomposition — Program should rely on functions that are consistent with the algorithm.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
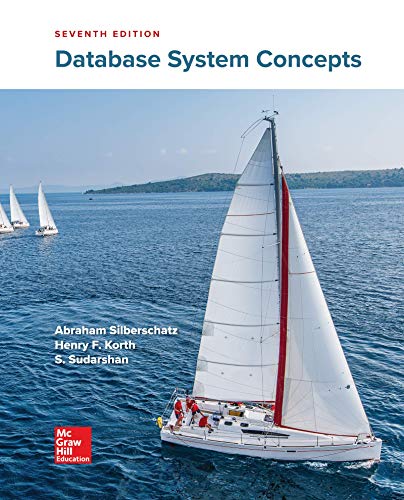
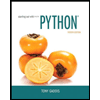
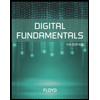
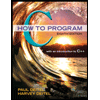
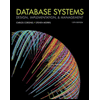
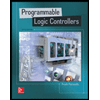