Right now we have a CourseListType that will hold a static 50 courses. Now we need to make that a little more flexible. To do that we want to change the static array to a dynamic array. So, what do we need to do? Modify CourseListType so that it contains a pointer to a CourseType rather than an array of CourseType. Modify the CourseListType constructor to create a dynamic array allocating space for a specific number of CourseType elements rather than always using 50. (Note: This is still not totally flexible, but is much better than a static limit.) Finally, we need to create a destructor to for CourseListType to deallocate space whenever a declared object goes out of scope.)
Right now we have a CourseListType that will hold a static 50 courses. Now we need to make that a little more flexible. To do that we want to change the static array to a dynamic array. So, what do we need to do?
- Modify CourseListType so that it contains a pointer to a CourseType rather than an array of CourseType.
- Modify the CourseListType constructor to create a dynamic array allocating space for a specific number of CourseType elements rather than always using 50. (Note: This is still not totally flexible, but is much better than a static limit.)
- Finally, we need to create a destructor to for CourseListType to deallocate space whenever a declared object goes out of scope.)
//courseListType.h file
#ifndef COURSELISTTYPE_H_INCLUDED
#define COURSELISTTYPE_H_INCLUDED
#include <string>
#include "courseType.h"
class courseTypeList {
public:
void print() const;
void addCourse(std::string);
courseTypeList();
private:
static const int NUM_COURSES = 50;
int courseCount;
courseType courses[NUM_COURSES];
};
#endif // COURSELISTTYPE_H_INCLUDED
//courseListType Implementation File
#include <iostream>
#include <string>
#include "courseTypeList.h"
void courseTypeList::print() const {
for (int i = 0; i < courseCount; i++) {
courses[i].print();
}
}
void courseTypeList::addCourse(std::string name) {
courses[courseCount++].setCourseName(name);
}
courseTypeList::courseTypeList() {
courseCount = 0;
}
===========================
This is just a reference to the courseType class that was mentioned in the above instructions:
//courseType.h file
#ifndef COURSETYPE_H_INCLUDED
#define COURSETYPE_H_INCLUDED
#include <iostream>
#include <string>
using namespace std;
class courseType {
public:
courseType(int, string);
int getCourseID();
string getCourseName();
void setCourseID(int);
void setCourseName(string);
void printCourse();
private:
int courseID;
string courseName;
};
//courseType Implementation FIile
#include "courseType.h"
#include <string>
using namespace std;
courseType::courseType(int id, string name) {
this-> courseID = id;
this-> courseName = name;
}
int courseType::getCourseID() {
return courseID;
}
string courseType::getCourseName() {
return courseName;
}
void courseType::setCourseID(int id) {
courseID = id;
}
void courseType::setCourseName (string name) {
courseName = name;
}
void courseType::printCourse() {
cout << "Course ID: " << getCourseID() << endl;
cout << "Course Name: " << getCourseName() << endl;
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

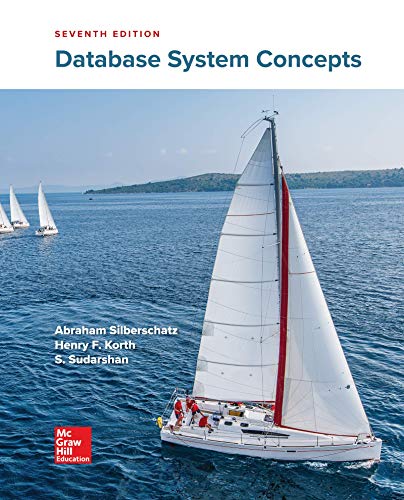
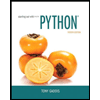
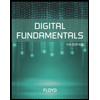
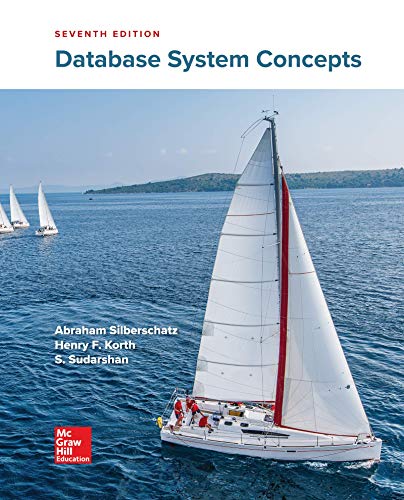
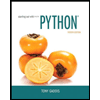
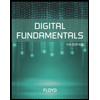
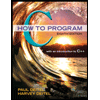
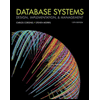
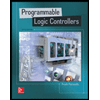