The program should have one class per .java file all calling to a main.java file using getters and setters. Here are the classes: Employee - this should handle user imput to gather Id (hard code to 1), first name, last name, middle initial, address, city, state, zip, phone, email, and hourly rate. PayPeriod - this should handle user input to gather payid (hard code to 123456), start date (hard code to 1), end date (hard code to 30), and hours worked. TaxPayment - this should have fields for federal tax, state tax, and fica tax with an instance of this class to pay period so we have a place to store it. TaxManager - this computea the taxes from the gross total and adds them to the overall output. Be sure to use a method such as TaxPayment ComputeTaxPayment(double grossPay, TaxRates taxRates) to be stored in PayPeriod. Array - self explanatory, do the array stuff here. PayrollManager - this class calculates the regular pay, overtime pay (if applicable), gross total and calls for PrintPaystub to print the paystub into main. Main - the main class that calls for all user input and PrintPaystub to print the necessary information
In this java assignment, we will need to use arraylists so we can store data for an employee.
If the user wants to enter data for more than one employee, it should print like this:
Employee List
Id Name
-----------------------
1. First Name
2. Bob Smith
If the user only enters one employee, it should print out a paystub for that employee that would look like this (with overtime coming into play as well, paying an extra 50% beyond 40 hours):
-------------------------------
Id - 1
Name - First M. Last
Address - 1234 Main St, City, St, Zip
Phone - 1234567890
Email - sample@gmail.com
Hours worked - 45
Hourly Rate - $10
Regular Pay, 40 hours at $10/hr - $400
Overtime Pay, 5 hours at $15/hr - $75
Gross Total - $475
------------------------------
Federal tax (20%) - $95
State Tax (5%) - $23.75
Fica Tax (3%) - $14.25
------------------------------
Net Check - $342
The program should have one class per .java file all calling to a main.java file using getters and setters. Here are the classes:
Employee - this should handle user imput to gather Id (hard code to 1), first name, last name, middle initial, address, city, state, zip, phone, email, and hourly rate.
PayPeriod - this should handle user input to gather payid (hard code to 123456), start date (hard code to 1), end date (hard code to 30), and hours worked.
TaxPayment - this should have fields for federal tax, state tax, and fica tax with an instance of this class to pay period so we have a place to store it.
TaxManager - this computea the taxes from the gross total and adds them to the overall output. Be sure to use a method such as TaxPayment ComputeTaxPayment(double grossPay, TaxRates taxRates) to be stored in PayPeriod.
Array - self explanatory, do the array stuff here.
PayrollManager - this class calculates the regular pay, overtime pay (if applicable), gross total and calls for PrintPaystub to print the paystub into main.
Main - the main class that calls for all user input and PrintPaystub to print the necessary information.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

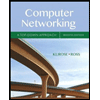
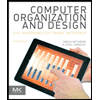
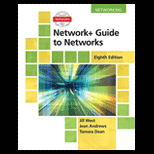
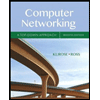
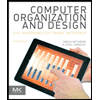
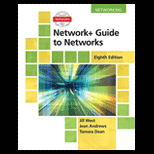
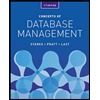
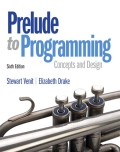
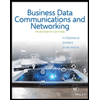