servationlist1) { this.reservationlist1 = reservatio
Chapter9: Using Classes And Objects
Section: Chapter Questions
Problem 9E
Related questions
Question
Please allow the buttons to sort properly the sortByname, sortbywaittime and capacity to all sort please
// Constructor that takes a Driver object as input
public EntryScreen(Driver reservationlist1) {
this.reservationlist1 = reservationlist1;
// Initialize the necessary components
txttableNumber = new JTextField(20);
setTitle("Make a Reservation");
pnlCommand = new JPanel();
pnlDisplay = new JPanel();
String[] columnNames = {"ID", "Name", "Capacity", "Date", "Time"};
tableModel = new DefaultTableModel(columnNames, 0);
table = new JTable(tableModel);
JScrollPane scrollPane = new JScrollPane(table);
add(scrollPane, BorderLayout.NORTH);
// create the table and set the model
// add the table to a scroll pane and add it to the GUI
// add labels and text fields to the display panel
pnlDisplay.add(new JLabel("Name:"));
txtName = new JTextField(20);
pnlDisplay.add(txtName);
pnlDisplay.add(new JLabel("Date:"));
txtDate = new JTextField(5);
pnlDisplay.add(txtDate);
pnlDisplay.add(new JLabel("Time:"));
txtTime = new JTextField(5);
pnlDisplay.add(txtTime);
pnlDisplay.add(new JLabel("Capacity:"));
txtcapacity = new JTextField(20);
pnlDisplay.add(txtcapacity);
// add the save and close buttons to the command panel
pnlCommand.setLayout(new GridLayout(1, 4));
SaveCommand = new JButton("Save");
commandCloser = new JButton("Close");
ClearAll = new JButton("Clear All");
cmdSortFirstName = new JButton("Sort by Name");
cmdSortWaittime= new JButton("Sort by Time");
Capacity= new JButton("Capacity");
pnlCommand.add(SaveCommand);
pnlCommand.add(commandCloser);
pnlCommand.add(ClearAll);
pnlCommand.add(cmdSortWaittime);
pnlCommand.add(cmdSortFirstName);
pnlCommand.add(Capacity);
// add the display and command panels to the GUI
add(pnlDisplay, BorderLayout.CENTER);
add(pnlCommand, BorderLayout.SOUTH);
// finalize the GUI and make it visible
pack();
setVisible(true);
// add action listeners to the buttons
commandCloser.addActionListener(new commandCloser());
SaveCommand.addActionListener(new SaveCommand());
// set this object to be the current instance of EntryScreen
ReservationEntry1 = this;
}
// ActionListener class for the close button
private class commandCloser implements ActionListener {
public void actionPerformed(ActionEvent d) {
ReservationEntry1.setVisible(false);
System.out.println("Close button clicked");
}
}
// ActionListener class for the save button
private class SaveCommand implements ActionListener {
public void actionPerformed(ActionEvent e) {
// generate a random ID and table number
Random random = new Random();
int id = random.nextInt(10000) + 1;
// extract information from the text fields
String name = txtName.getText();
String[] Name = name.split(", ");
String Capacity = txtcapacity.getText();
int capacity = 0;
if (!Capacity.isEmpty()) {
try {
capacity = Integer.parseInt(Capacity);
} catch (NumberFormatException ex) {
System.out.println(" Error");
}
}
String date = txtDate.getText();
String time = txtTime.getText();
// create a reservation object
Reservation reservation = new Reservation(id, name, tableNumber, capacity, date, time);
// add the reservation data to the table model
Object[] rowData = {id, name, capacity, date, time};
tableModel.addRow(rowData);
// add the reservation to the driver's list
reservationlist1.addReservation(reservation);
// hide the reservation entry screen
ReservationEntry1.setVisible(true);
// display ID in a dialog box
JOptionPane.showMessageDialog(null, "Reservation ID: " + id);
}
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
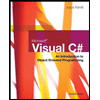
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
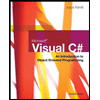
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,