Testing Standard techniques for testing also apply here. You will want to check expected vs. actual values produced. Another thing to check is logging, but ensure that you make a comment about this if you are looking at the logs. That said, in a multi-threaded environment we are leading ourselves into non- deterministic runtime environments so it will be impossible to check expected vs actual values for all of your metrics (some will still work though). There is only one way around this: build unit tests for all of your code. These tests only run a single portion of your code (e.g. one test for a single method in a class). Given the time constraint, building unit tests is unfeasible as it can take as much, or more time to write than the source code. In this case, you can test your program by running a handful of known successful tests, along with a collection of tests that probe edge cases in your program (e.g. can it handle 0 actions/second?). Design Decisions Report Write out your reasons for doing one thing over another, as well as listing all the design patterns you used and where they are located. This will include reasoning for things like "why I used the Factory method in location XYZ". Include a visual design of your program. The visualization does not need to follow UML conventions, but it needs to be clear, and simple. This design should/could be partially completed prior to programming as well. I expect to see all of the following in your document: ― Overview of your program. o Idea you selected. Overview of how it's implemented. ― Design/implementation decisions - • Patterns used • Choice of logic and/or data structures • Usage of DRY, SOLID • Synchronization choices • Etc. ― Visual design of the program • Try using an extension in your IDE to produce a UML diagram • Alternatively, you can build a simplified one that doesn't need to follow UML Skeleton Program Along with this document, you'll find a skeleton program that you can build from. It has the following components: Matrix: This is where your simulation is run from, and where you will be creating your objects and threads. -Unit: Create concrete classes which inherit from this abstract class. See the Robot example in Main.java. SimulationInput: This is a helpful container for the input to the simulation. It will hold things like the amount of time to run for, and the number of actions that should be performed per second. ― Statistics: This is a singleton that will hold the statistics for the simulation. You will need to modify it to add new statistics and provide a way to output them. Main: This is where the program starts from. It should be used for testing. You will find helper methods for running tests with different forms of input. Be sure to read through all the code in those files, there are hints to help you scattered throughout. You are free to modify them but the final product needs to do the same thing as the original (I suggest not changing the basic functionality). The skeleton program is straightforward: It creates an input object called SimulationInput and adds the input to it. wwwww Then it starts a test with the Robot example class (in Main.java): o Here, we initialize the Statistics singleton with the given input. • Then, we start the Matrix simulation. ♣ The test initializes the Robot/Unit example and runs it with the given input. • Finally, we return the statistics object to the main method. ― After the test, we output the final statistics from the test run. Your units are all Runnable so they absolutely must be run as a Thread. They need to output information as they perform their actions as well, they can't stay silent in the background (unless that is purposeful). The simulation must be entirely multi-threaded. You must use 1 semaphore for some form of interactivity.
Testing Standard techniques for testing also apply here. You will want to check expected vs. actual values produced. Another thing to check is logging, but ensure that you make a comment about this if you are looking at the logs. That said, in a multi-threaded environment we are leading ourselves into non- deterministic runtime environments so it will be impossible to check expected vs actual values for all of your metrics (some will still work though). There is only one way around this: build unit tests for all of your code. These tests only run a single portion of your code (e.g. one test for a single method in a class). Given the time constraint, building unit tests is unfeasible as it can take as much, or more time to write than the source code. In this case, you can test your program by running a handful of known successful tests, along with a collection of tests that probe edge cases in your program (e.g. can it handle 0 actions/second?). Design Decisions Report Write out your reasons for doing one thing over another, as well as listing all the design patterns you used and where they are located. This will include reasoning for things like "why I used the Factory method in location XYZ". Include a visual design of your program. The visualization does not need to follow UML conventions, but it needs to be clear, and simple. This design should/could be partially completed prior to programming as well. I expect to see all of the following in your document: ― Overview of your program. o Idea you selected. Overview of how it's implemented. ― Design/implementation decisions - • Patterns used • Choice of logic and/or data structures • Usage of DRY, SOLID • Synchronization choices • Etc. ― Visual design of the program • Try using an extension in your IDE to produce a UML diagram • Alternatively, you can build a simplified one that doesn't need to follow UML Skeleton Program Along with this document, you'll find a skeleton program that you can build from. It has the following components: Matrix: This is where your simulation is run from, and where you will be creating your objects and threads. -Unit: Create concrete classes which inherit from this abstract class. See the Robot example in Main.java. SimulationInput: This is a helpful container for the input to the simulation. It will hold things like the amount of time to run for, and the number of actions that should be performed per second. ― Statistics: This is a singleton that will hold the statistics for the simulation. You will need to modify it to add new statistics and provide a way to output them. Main: This is where the program starts from. It should be used for testing. You will find helper methods for running tests with different forms of input. Be sure to read through all the code in those files, there are hints to help you scattered throughout. You are free to modify them but the final product needs to do the same thing as the original (I suggest not changing the basic functionality). The skeleton program is straightforward: It creates an input object called SimulationInput and adds the input to it. wwwww Then it starts a test with the Robot example class (in Main.java): o Here, we initialize the Statistics singleton with the given input. • Then, we start the Matrix simulation. ♣ The test initializes the Robot/Unit example and runs it with the given input. • Finally, we return the statistics object to the main method. ― After the test, we output the final statistics from the test run. Your units are all Runnable so they absolutely must be run as a Thread. They need to output information as they perform their actions as well, they can't stay silent in the background (unless that is purposeful). The simulation must be entirely multi-threaded. You must use 1 semaphore for some form of interactivity.
Chapter11: Advanced Inheritance Concepts
Section: Chapter Questions
Problem 3PE
Related questions
Question
I really need help with this one please, its really hard for me. In Java, could you use all the new tools and techniques to build a simple simulation of
Social Media Simulation
it absolutely must use the skeleton provided. you can also implement a Java Swing visualization for your simulation. Before starting, carefully read everything below, you need to implement at least 5 design patterns of your choice.
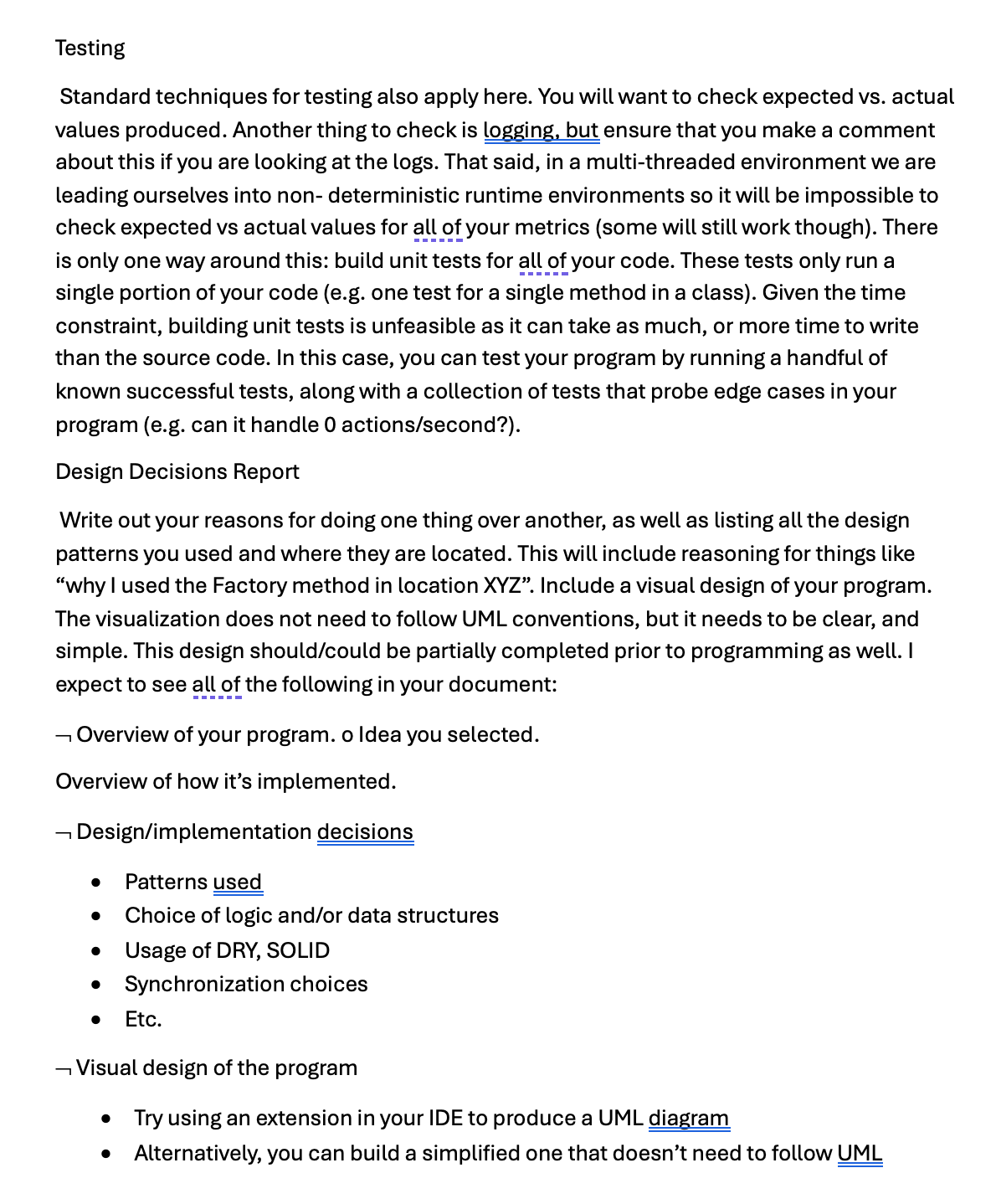
Transcribed Image Text:Testing
Standard techniques for testing also apply here. You will want to check expected vs. actual
values produced. Another thing to check is logging, but ensure that you make a comment
about this if you are looking at the logs. That said, in a multi-threaded environment we are
leading ourselves into non- deterministic runtime environments so it will be impossible to
check expected vs actual values for all of your metrics (some will still work though). There
is only one way around this: build unit tests for all of your code. These tests only run a
single portion of your code (e.g. one test for a single method in a class). Given the time
constraint, building unit tests is unfeasible as it can take as much, or more time to write
than the source code. In this case, you can test your program by running a handful of
known successful tests, along with a collection of tests that probe edge cases in your
program (e.g. can it handle 0 actions/second?).
Design Decisions Report
Write out your reasons for doing one thing over another, as well as listing all the design
patterns you used and where they are located. This will include reasoning for things like
"why I used the Factory method in location XYZ". Include a visual design of your program.
The visualization does not need to follow UML conventions, but it needs to be clear, and
simple. This design should/could be partially completed prior to programming as well. I
expect to see all of the following in your document:
― Overview of your program. o Idea you selected.
Overview of how it's implemented.
― Design/implementation decisions
-
• Patterns used
• Choice of logic and/or data structures
• Usage of DRY, SOLID
•
Synchronization choices
•
Etc.
― Visual design of the program
• Try using an extension in your IDE to produce a UML diagram
•
Alternatively, you can build a simplified one that doesn't need to follow UML
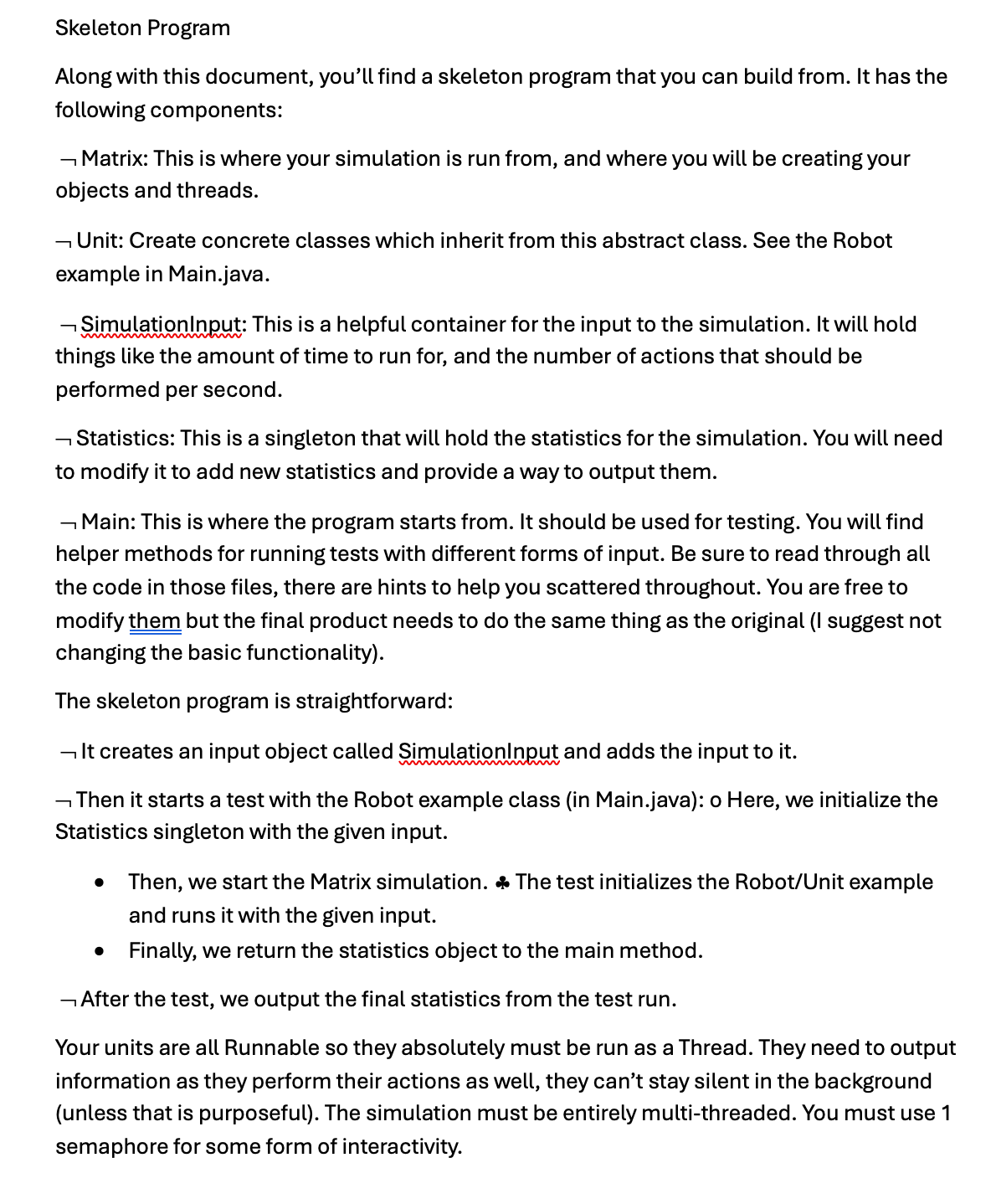
Transcribed Image Text:Skeleton Program
Along with this document, you'll find a skeleton program that you can build from. It has the
following components:
Matrix: This is where your simulation is run from, and where you will be creating your
objects and threads.
-Unit: Create concrete classes which inherit from this abstract class. See the Robot
example in Main.java.
SimulationInput: This is a helpful container for the input to the simulation. It will hold
things like the amount of time to run for, and the number of actions that should be
performed per second.
― Statistics: This is a singleton that will hold the statistics for the simulation. You will need
to modify it to add new statistics and provide a way to output them.
Main: This is where the program starts from. It should be used for testing. You will find
helper methods for running tests with different forms of input. Be sure to read through all
the code in those files, there are hints to help you scattered throughout. You are free to
modify them but the final product needs to do the same thing as the original (I suggest not
changing the basic functionality).
The skeleton program is straightforward:
It creates an input object called SimulationInput and adds the input to it.
wwwww
Then it starts a test with the Robot example class (in Main.java): o Here, we initialize the
Statistics singleton with the given input.
• Then, we start the Matrix simulation. ♣ The test initializes the Robot/Unit example
and runs it with the given input.
• Finally, we return the statistics object to the main method.
― After the test, we output the final statistics from the test run.
Your units are all Runnable so they absolutely must be run as a Thread. They need to output
information as they perform their actions as well, they can't stay silent in the background
(unless that is purposeful). The simulation must be entirely multi-threaded. You must use 1
semaphore for some form of interactivity.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
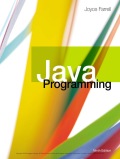
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
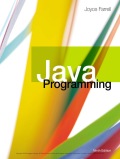
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT