Design and implement a Java class named Book with two data members: title and price. The class should have suitable constructors, get/set methods, and the toString method. Design and implement another Java class named BookShelf which has an ArrayList data member named bookList to store books. The class should have suitable constructors, get/set methods, and the toString method, as well as methods for people to add a book (prototype: addBook(Book book)), remove a book (prototype: removeBook(Book book)), and search for a book (prototype: findBook(Book book)). Test the two classes by creating a Bookshelf object and five Book objects. Add the books to the bookshelf. Display the contents of the bookshelf. Test the removeBook and findBook methods as well. Take screenshots of your tests and submit them with your Java code (not the whole project)
Design and implement a Java class named Book with two data members: title and price. The class should have suitable constructors, get/set methods, and the toString method. Design and implement another Java class named BookShelf which has an ArrayList data member named bookList to store books. The class should have suitable constructors, get/set methods, and the toString method, as well as methods for people to add a book (prototype: addBook(Book book)), remove a book (prototype: removeBook(Book book)), and search for a book (prototype: findBook(Book book)). Test the two classes by creating a Bookshelf object and five Book objects. Add the books to the bookshelf. Display the contents of the bookshelf. Test the removeBook and findBook methods as well. Take screenshots of your tests and submit them with your Java code (not the whole project)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

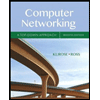
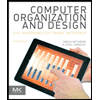
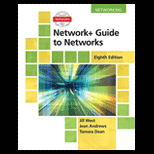
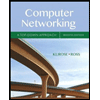
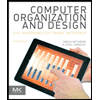
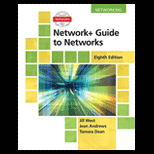
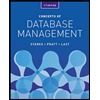
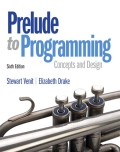
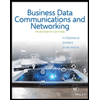