someone help me modify the code please. Instructions: In the code editor, you are provided with the main() function that asks the user for 10 elements that represents the options for the ring as well as a single integer value that represents the ring that she wants. Then, a call to the findRing() function is made and the array of rings and the ring she wants are passed into it. Your task is to implement the findRing() function. This has the following details: Return type - int Name - findRing Parameters int* - for the array of rings int - size of the array of rings int - the ring that she wants Return value - the index of the ring in the array of rings. It is guaranteed that there is only one such ring that matches the ring that she wants. Input 1. Ten integer values representing the rings 2. Integer value representing the ring she wants #include int findRing(int rings[],int n,int wantedRing) {int index=0; for(int i=0;i
someone help me modify the code please.
Instructions:
- In the code editor, you are provided with the main() function that asks the user for 10 elements that represents the options for the ring as well as a single integer value that represents the ring that she wants. Then, a call to the findRing() function is made and the array of rings and the ring she wants are passed into it.
-
Your task is to implement the findRing() function. This has the following details:
- Return type - int
- Name - findRing
-
Parameters
- int* - for the array of rings
- int - size of the array of rings
- int - the ring that she wants
- Return value - the index of the ring in the array of rings. It is guaranteed that there is only one such ring that matches the ring that she wants.
Input
1. Ten integer values representing the rings
2. Integer value representing the ring she wants
#include <stdio.h>
int findRing(int rings[],int n,int wantedRing)
{int index=0;
for(int i=0;i<n;i++)
{
if(rings[i]==wantedRing)//Performing a linear search to find the wanted ring
{
index=i;
}
}
return index;
}
int main() {
int rings[10];
for(int i = 0; i <10; i++) {
printf("Enter option #%d: ", i + 1);
scanf("%d", &rings[i]);
}
int wantedRing;
printf("Enter the ring she wants: ");
scanf("%d", &wantedRing);
printf("\nRing %d found at option %d!", wantedRing, findRing(rings, 10, wantedRing) + 1);
return 0;
}
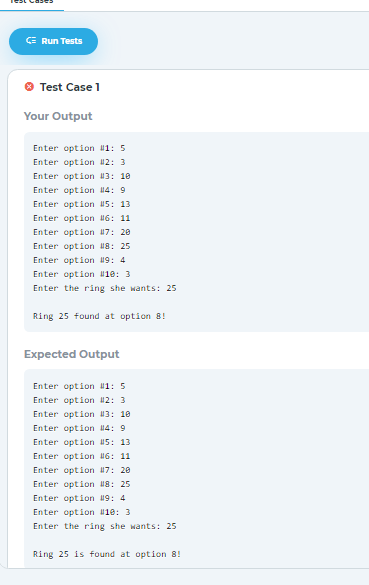

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

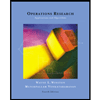
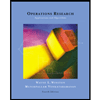