STEP 1 – Create Superclass and Subclasses Create an Eclipse project named JohnDoeHw7, and use the default package, i.e., no package name should be provided. Inside the project, create the parent class (superclass) Player as specified in the table below. Save it in a file Player.java. Create the two child classes (subclasses) shown below. Save each class in a separate file – BaseballPlayer.java, and BasketballPlayer.java. Class names Player (superclass) id player name BaseballPlayer (subclass of Player) number of hits number of times at bat BasketballPlayer (subclass of Player) number of shots made number of shots attempted team name position
STEP 1 – Create Superclass and Subclasses Create an Eclipse project named JohnDoeHw7, and use the default package, i.e., no package name should be provided. Inside the project, create the parent class (superclass) Player as specified in the table below. Save it in a file Player.java. Create the two child classes (subclasses) shown below. Save each class in a separate file – BaseballPlayer.java, and BasketballPlayer.java. Class names Player (superclass) id player name BaseballPlayer (subclass of Player) number of hits number of times at bat BasketballPlayer (subclass of Player) number of shots made number of shots attempted team name position
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
I am in Java class now. Can you guys help me to do this assignment please ?
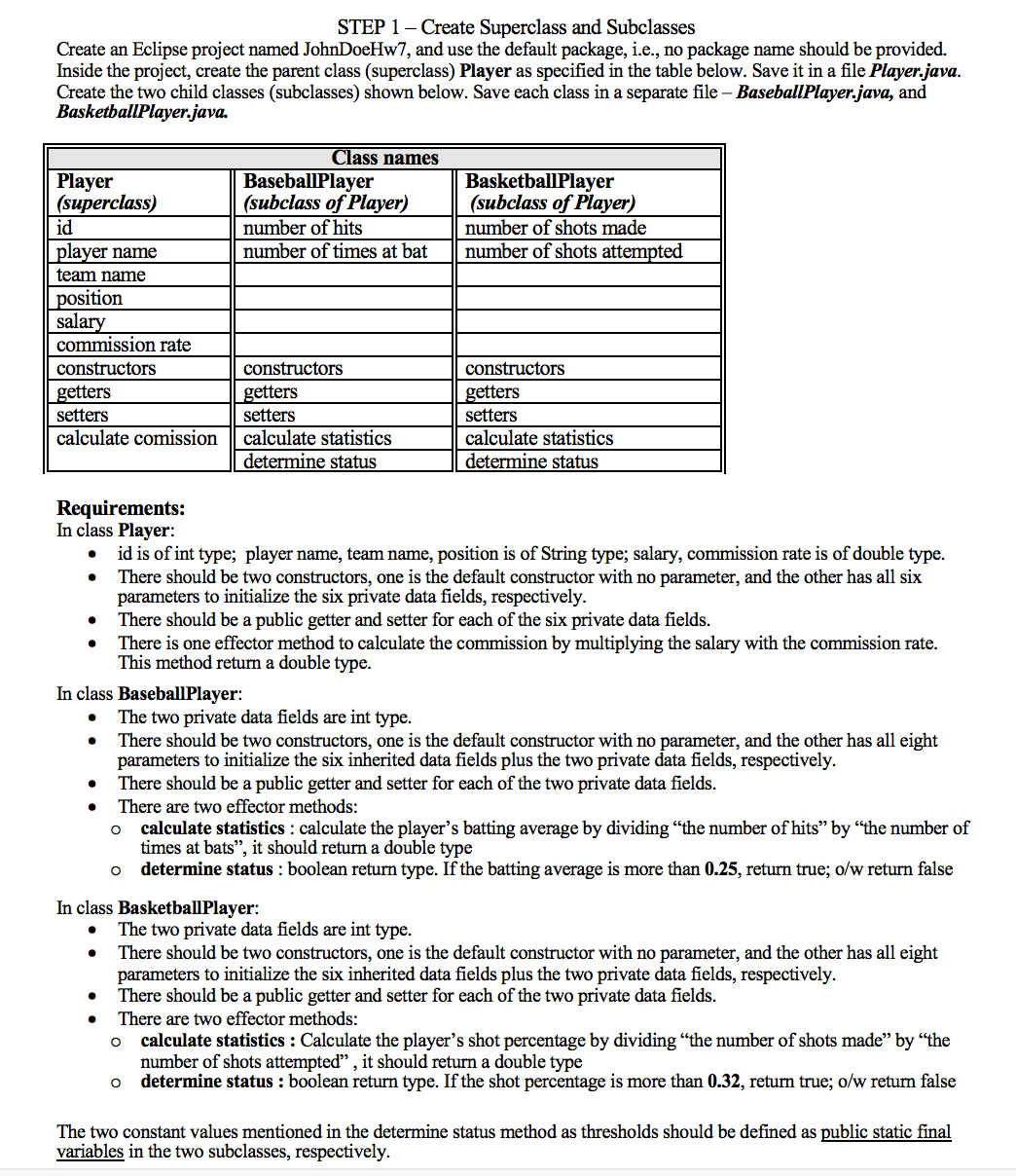
Transcribed Image Text:STEP 1 – Create Superclass and Subclasses
Create an Eclipse project named JohnDoeHw7, and use the default package, i.e., no package name should be provided.
Inside the project, create the parent class (superclass) Player as specified in the table below. Save it in a file Player.java.
Create the two child classes (subclasses) shown below. Save each class in a separate file – BaseballPlayer.java, and
BasketballPlayer.java.
Class names
Player
(superclass)
id
BaseballPlayer
(subclass of Player)
BasketballPlayer
(subclass of Player)
number of shots made
number of hits
number of times at bat
player name
number of shots attempted
team name
position
salary
commission rate
constructors
constructors
constructors
getters
getters
| getters
setters
setters
setters
calculate comission
calculate statistics
determine status
calculate statistics
| determine status
Requirements:
In class Player:
id is of int type; player name, team name, position is of String type; salary, commission rate is of double type.
There should be two constructors, one is the default constructor with no parameter, and the other has all six
parameters to initialize the six private data fields, respectively.
There should be a public getter and setter for each of the six private data fields.
There is one effector method to calculate the commission by multiplying the salary with the commission rate.
This method return a double type.
In class BaseballPlayer:
The two private data fields are int type.
There should be two constructors, one is the default constructor with no parameter, and the other has all eight
parameters to initialize the six inherited data fields plus the two private data fields, respectively.
There should be a public getter and setter for each of the two private data fields.
There are two effector methods:
calculate statistics : calculate the player's batting average by dividing "the number of hits" by "the number of
times at bats", it should return a double type
determine status : boolean return type. If the batting average is more than 0.25, return true; o/w return false
In class BasketballPlayer:
The two private data fields are int type.
There should be two constructors, one is the default constructor with no parameter, and the other has all eight
parameters to initialize the six inherited data fields plus the two private data fields, respectively.
There should be a public getter and setter for each of the two private data fields.
There are two effector methods:
calculate statistics : Calculate the player's shot percentage by dividing "the number of shots made" by “the
number of shots attempted" , it should return a double type
determine status : boolean return type. If the shot percentage is more than 0.32, return true; o/w return false
The two constant values mentioned in the determine status method as thresholds should be defined as public static final
variables in the two subclasses, respectively.
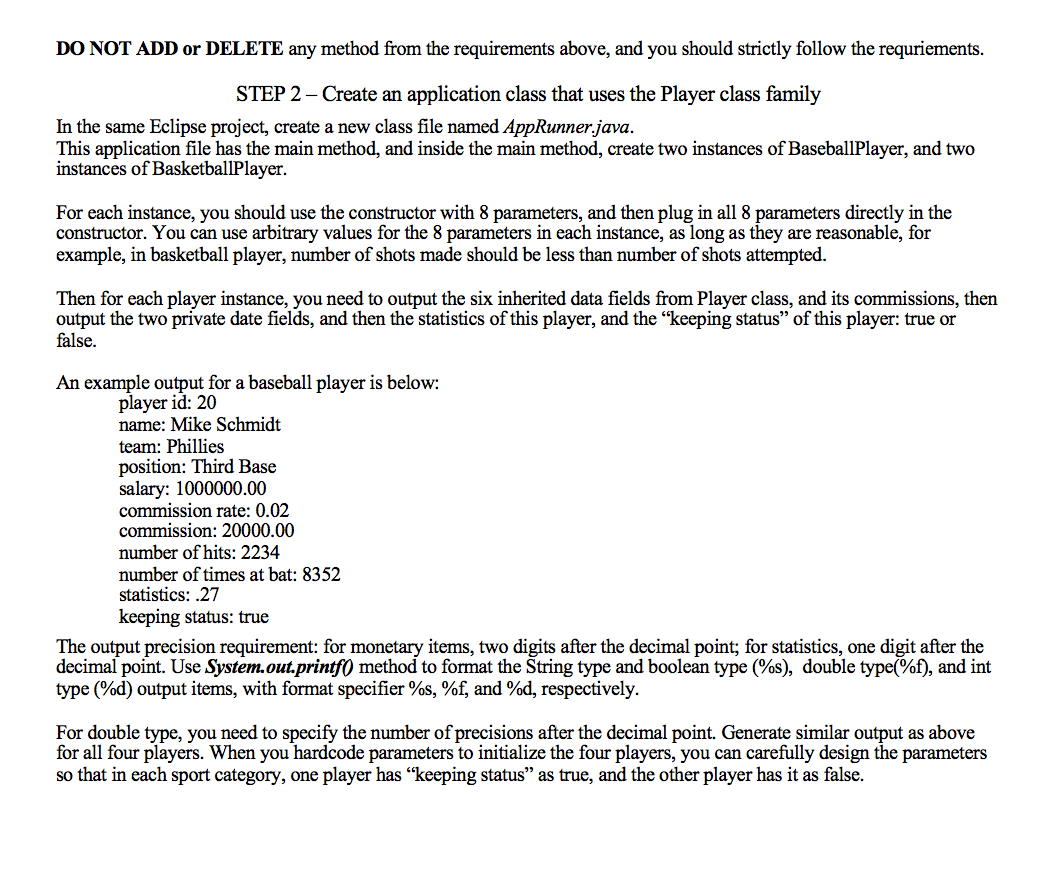
Transcribed Image Text:DO NOT ADD or DELETE any method from the requirements above, and you should strictly follow the requriements.
STEP 2 – Create an application class that uses the Player class family
In the same Eclipse project, create a new class file named AppRunner.java.
This application file has the main method, and inside the main method, create two instances of BaseballPlayer, and two
instances of BasketballPlayer.
For each instance, you should use the constructor with 8 parameters, and then plug in all 8 parameters directly in the
constructor. You can use arbitrary values for the 8 parameters in each instance, as long as they are reasonable, for
example, in basketball player, number of shots made should be less than number of shots attempted.
Then for each player instance, you need to output the six inherited data fields from Player class, and its commissions, then
output the two private date fields, and then the statistics of this player, and the "keeping status" of this player: true or
false.
An example output for a baseball player is below:
player id: 20
name: Mike Schmidt
team: Phillies
position: Third Base
salary: 1000000.00
commission rate: 0.02
commission: 20000.00
number of hits: 2234
number of times at bat: 8352
statistics: .27
keeping status: true
The output precision requirement: for monetary items, two digits after the decimal point; for statistics, one digit after the
decimal point. Use System.out.printf() method to format the String type and boolean type (%s), double type(%f), and int
type (%d) output items, with format specifier %s, %f, and %d, respectively.
For double type, you need to specify the number of precisions after the decimal point. Generate similar output as above
for all four players. When you hardcode parameters to initialize the four players, you can carefully design the parameters
so that in each sport category, one player has "keeping status" as true, and the other player has it as false.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
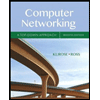
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
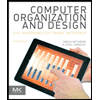
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
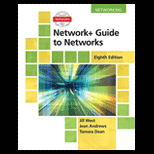
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
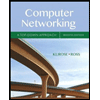
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
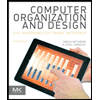
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
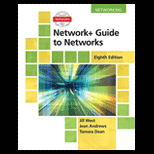
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
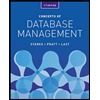
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
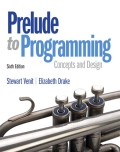
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
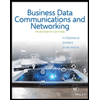
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY