The purpose of this assignment is to practice OOP with Decisions, Loops, Arrays and ArrayList, Input/Output Files, and Constructing Objects and Classes. Create a NetBeans project named HW2_YourId. Develop classes for the required solutions. Important: Apply good programming practices: Provide API documentation comments for your class(s), class constructor(s) and method(s) using the Java standard form for documentation comments discussed in this course. Use meaningful variable and constant names. Show vour name university id and section number as a comment at the start of each class
The purpose of this assignment is to practice OOP with Decisions, Loops, Arrays and ArrayList, Input/Output Files, and Constructing Objects and Classes. Create a NetBeans project named HW2_YourId. Develop classes for the required solutions. Important: Apply good programming practices: Provide API documentation comments for your class(s), class constructor(s) and method(s) using the Java standard form for documentation comments discussed in this course. Use meaningful variable and constant names. Show vour name university id and section number as a comment at the start of each class
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter12: Event-driven Gui Programming, Multithreading, And Animation
Section: Chapter Questions
Problem 8PE
Related questions
Topic Video
Question
write java
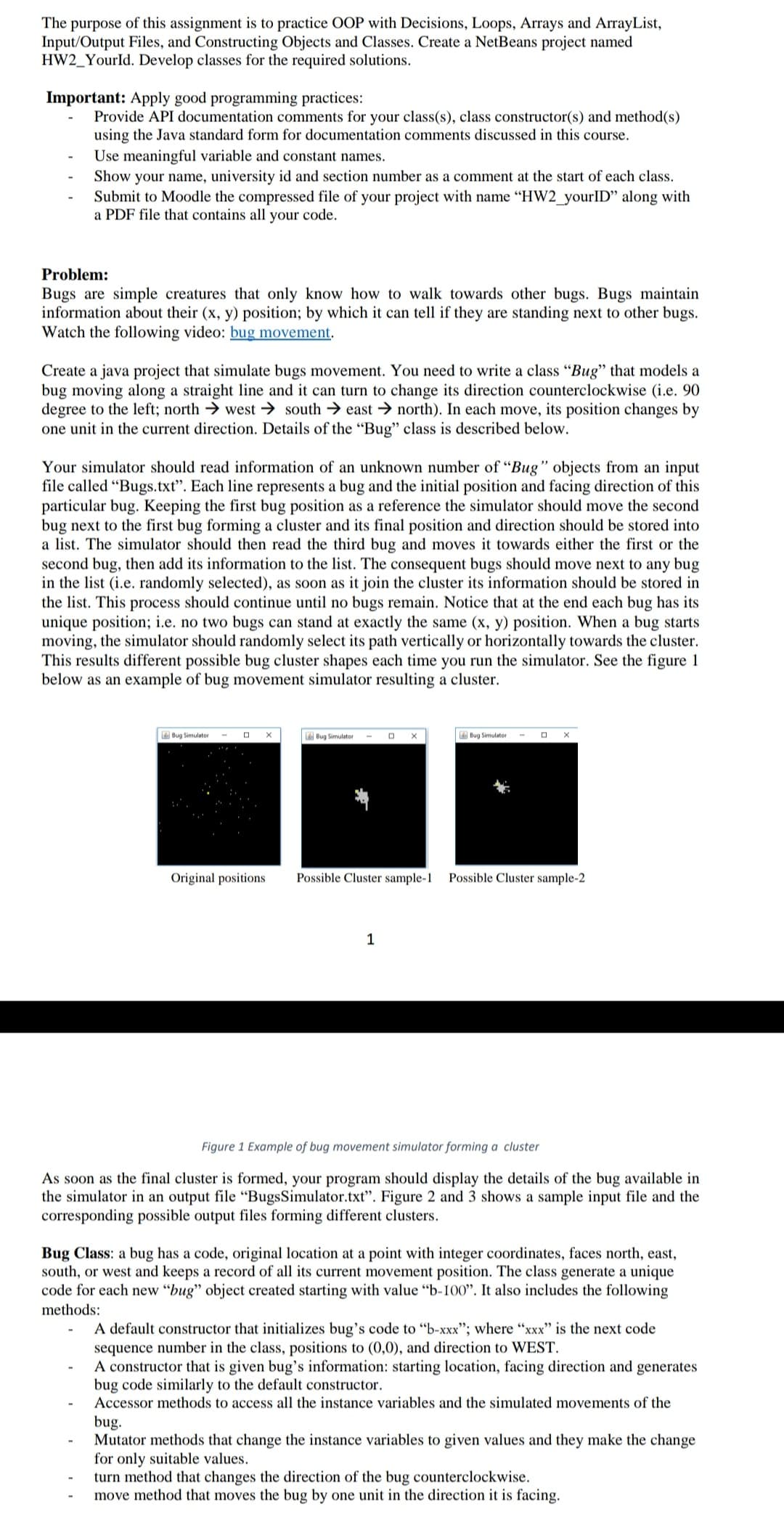
Transcribed Image Text:The purpose of this assignment is to practice OOP with Decisions, Loops, Arrays and ArrayList,
Input/Output Files, and Constructing Objects and Classes. Create a NetBeans project named
HW2_YourId. Develop classes for the required solutions.
Important: Apply good programming practices:
Provide API documentation comments for your class(s), class constructor(s) and method(s)
using the Java standard form for documentation comments discussed in this course.
Use meaningful variable and constant names.
Show your name, university id and section number as a comment at the start of each class.
Submit to Moodle the compressed file of your project with name "HW2_yourlID" along with
a PDF file that contains all your code.
Problem:
Bugs are simple creatures that only know how to walk towards other bugs. Bugs maintain
information about their (x, y) position; by which it can tell if they are standing next to other bugs.
Watch the following video: bug movement.
Create a java project that simulate bugs movement. You need to write a class "Bug" that models a
bug moving along a straight line and it can turn to change its direction counterclockwise (i.e. 90
degree to the left; north → west → south → east → north). In each move, its position changes by
one unit in the current direction. Details of the "Bug" class is described below.
Your simulator should read information of an unknown number of “Bug" objects from an input
file called "Bugs.txt". Each line represents a bug and the initial position and facing direction of this
particular bug. Keeping the first bug position as a reference the simulator should move the second
bug next to the first bug forming a cluster and its final position and direction should be stored into
a list. The simulator should then read the third bug and moves it towards either the first or the
second bug, then add its information to the list. The consequent bugs should move next to any bug
in the list (i.e. randomly selected), as soon as it join the cluster its information should be stored in
the list. This process should continue until no bugs remain. Notice that at the end each bug has its
unique position; i.e. no two bugs can stand at exactly the same (x, y) position. When a bug starts
moving, the simulator should randomly select its path vertically or horizontally towards the cluster.
This results different possible bug cluster shapes each time you run the simulator. See the figure 1
below as an example of bug movement simulator resulting a cluster.
Bug Simulator
Bug Simulator
Bug Simulator
Original positions
Possible Cluster sample-1
Possible Cluster sample-2
1
Figure 1 Example of bug movement simulator forming a cluster
As soon as the final cluster is formed, your program should display the details of the bug available in
the simulator in an output file “BugsSimulator.txt". Figure 2 and 3 shows a sample input file and the
corresponding possible output files forming different clusters.
Bug Class: a bug has a code, original location at a point with integer coordinates, faces north, east,
south, or west and keeps a record of all its current movement position. The class generate a unique
code for each new "bug" object created starting with value "b-I00". It also includes the following
methods:
A default constructor that initializes bug's code to "b-xxx"; where "xxx" is the next code
sequence number in the class, positions to (0,0), and direction to WEST.
A constructor that is given bug's information: starting location, facing direction and generates
bug code similarly to the default constructor.
Accessor methods to access all the instance variables and the simulated movements of the
bug.
Mutator methods that change the instance variables to given values and they make the change
for only suitable values.
turn method that changes the direction of the bug counterclockwise.
move method that moves the bug by one unit in the direction it is facing.
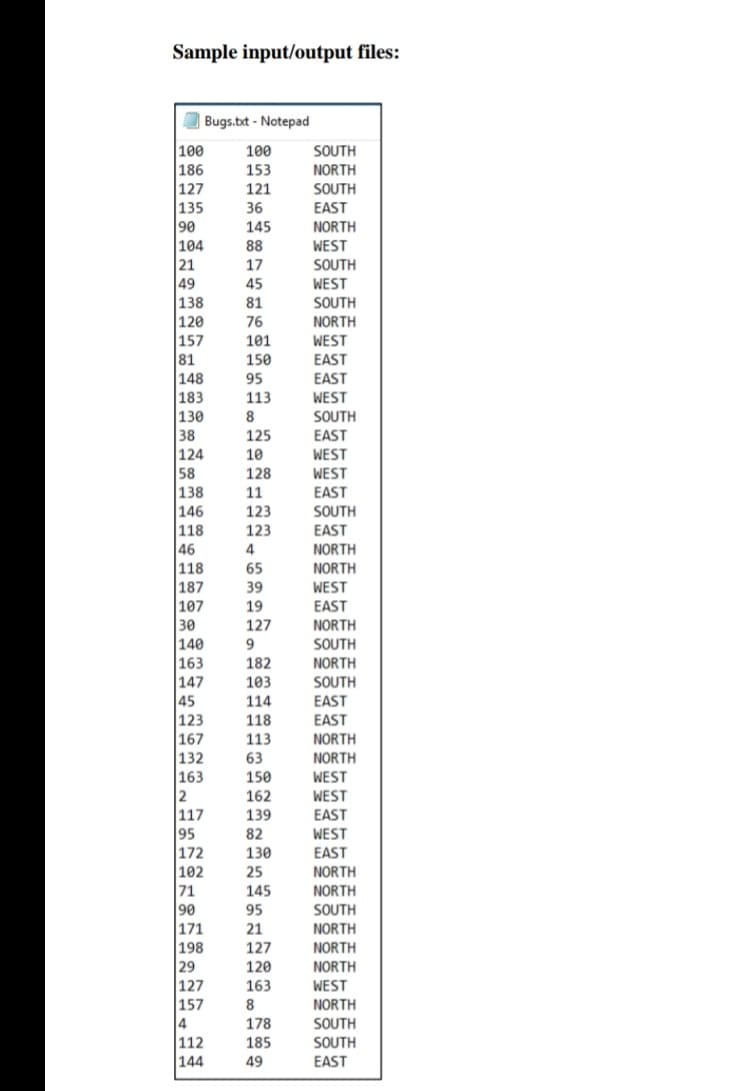
Transcribed Image Text:Sample input/output files:
O Bugs.bt - Notepad
100
186
127
135
90
104
|21
49
138
100
SOUTH
153
NORTH
121
SOUTH
36
EAST
NORTH
WEST
145
88
17
SOUTH
45
WEST
81
SOUTH
120
157
81
76
NORTH
101
WEST
150
EAST
148
95
EAST
183
113
WEST
130
38
|124
58
138
146
118
46
118
187
107
30
|140
8
SOUTH
125
EAST
10
WEST
128
WEST
11
EAST
123
SOUTH
EAST
NORTH
123
4
65
NORTH
WEST
EAST
39
19
NORTH
SOUTH
127
9
163
182
NORTH
147
45
123
167
132
163
103
SOUTH
EAST
EAST
NORTH
114
118
113
63
NORTH
150
WEST
WEST
EAST
162
117
95
|172
102
71
90
171
198
29
127
157
4
112
144
139
82
WEST
130
EAST
NORTH
NORTH
25
145
95
SOUTH
21
NORTH
127
NORTH
120
NORTH
163
WEST
8
NORTH
178
SOUTH
185
SOUTH
49
EAST
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage