Study the scenario and complete the question(s) that follow: Checkers is a retail company with many shops across South Africa’s nine provinces. They would like an application that they can use for stock taking of the products they sell. They have approached you as an experienced Java developer to aid them in developing an application they can use at their warehouses when they receive stock of new products. The application should implement a client server architecture where the server acts as the back end between the client and the database. The application should allow the user to enter product details on the client side, and the server side should receive these details and store them in a database. Create a Java application for Checkers called CheckersStockTakingApp. The application should consist of a client side, a server side and a database. Question Create a database called checkersproducts using MySQL or any relational database management software you are comfortable with. The database should have a table called products that consists of the following columns and properties: prodID AUTO NUMBER (primary) prodName VARCHAR (30) prodType VARCHAR (30) prodPrice DECIMAL (6,2) Also Create a server class that will do the following: • Run on port 8000 and listen to a client • Initially output a “server is starting” message to the screen • Make a connection to the database • Receive product details from the client • Add product details to products table • Send a message back to client to inform whether or not the product was added • Handle any exceptions • Close the server when the user types "stop" on the client side and output a message "Server stopping...." (see Figure 6 attached) Also Create a client class that will do the following: • Create a socket to connect to the server using port 8000 • Initially show a welcome message (see Figure 5 attached) • Prompt the user to enter product name, type and price • Send product details to server • Receive response from server to indicate whether product has been added to database or not • Allow user to add more products • Close the client when the user types “stop” for the product name and output a message “Exiting Checkers Stock Taking App, Good bye
Study the scenario and complete the question(s) that follow:
Checkers is a retail company with many shops across South Africa’s nine provinces. They would like an application that they can use for stock taking of the products they sell. They have approached you as an experienced Java developer to aid them in developing an application they can use at their warehouses when they receive stock of new products. The application should implement a client server architecture where the server acts as the back end between the client and the
Create a Java application for Checkers called CheckersStockTakingApp. The application
should consist of a client side, a server side and a database.
Question Create a database called checkersproducts using MySQL or any relational database management software you are comfortable with. The database should have a table called products that consists of the following columns and properties:
prodID AUTO NUMBER (primary)
prodName VARCHAR (30)
prodType VARCHAR (30)
prodPrice DECIMAL (6,2)
Also Create a server class that will do the following:
• Run on port 8000 and listen to a client
• Initially output a “server is starting” message to the screen
• Make a connection to the database
• Receive product details from the client
• Add product details to products table
• Send a message back to client to inform whether or not the product was added
• Handle any exceptions
• Close the server when the user types "stop" on the client side and output a message
"Server stopping...." (see Figure 6 attached)
Also Create a client class that will do the following:
• Create a socket to connect to the server using port 8000
• Initially show a welcome message (see Figure 5 attached)
• Prompt the user to enter product name, type and price
• Send product details to server
• Receive response from server to indicate whether product has been added to database
or not
• Allow user to add more products
• Close the client when the user types “stop” for the product name and output a message
“Exiting Checkers Stock Taking App, Good bye!"
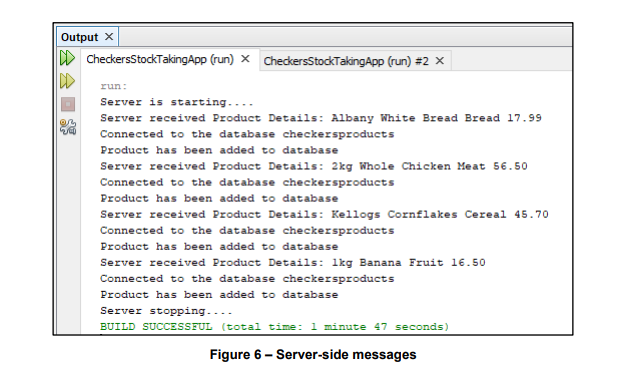
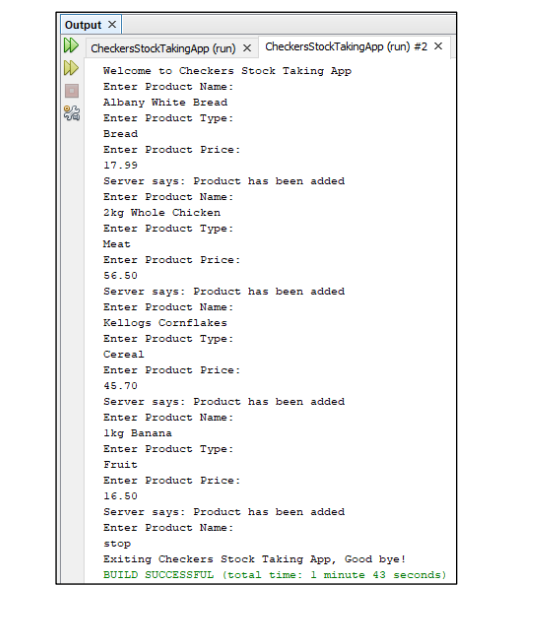

Step by step
Solved in 2 steps with 4 images

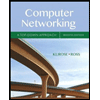
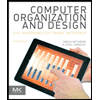
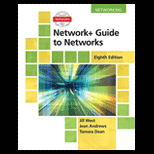
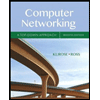
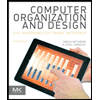
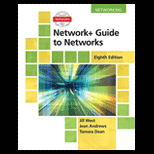
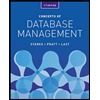
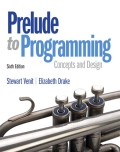
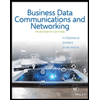