Summary 2 EmployeeBonus.py - This program calculates an emplo productivity bonus. 3 In this lab, you complete a prewritten Python program that calculates an employee's productivity 4 5 # Initialize variables here. 6 BONUS_1 = 50.00 7 BONUS_2 = 75.00 bonus and prints the employee's name and bonus. Bonuses are calculated based on an employee's productivity score as shown in the table below. A 8 BONUS_3 = 100.00 productivity score is calculated by first dividing an employee's transactions' dollar value by the 9 BONUS_4 = 200.00 number of transactions and then dividing the result by the number of shifts worked. 10 11 employeeName = input("Enter employee's name: ") 12 shiftString = input("Enter number of shifts: ") 13 transactString = input("Enter number of transaction 14 dollarString = input("Enter transactions dollar val Productivity Score Bonus s30 $50.00 15 16 numShifts = float(shiftString) 17 numTransactions = float(transactString) 18 dollarValue = float(dollarString) 31 - 69 $75.00 70 - 199 $100.00 19 # Write your code here 2200 $200.00 20 21 # Output. 22 print("Employee Name: " + employeeName) 23 print("Employee Bonus: $" + str(bonus)) 24 Instructions 1. Make sure the file EmployeeBonus.py is selected and open. 2. Variables have been declared for you, and the input statements and print statements have been written. Read them over carefully before you proceed to the next step. 3. Design the logic and write the rest of the program using a nested if statement. 4. Execute the program by clicking the Run button at the bottom of the screen. 5. Enter the following as input:
Summary 2 EmployeeBonus.py - This program calculates an emplo productivity bonus. 3 In this lab, you complete a prewritten Python program that calculates an employee's productivity 4 5 # Initialize variables here. 6 BONUS_1 = 50.00 7 BONUS_2 = 75.00 bonus and prints the employee's name and bonus. Bonuses are calculated based on an employee's productivity score as shown in the table below. A 8 BONUS_3 = 100.00 productivity score is calculated by first dividing an employee's transactions' dollar value by the 9 BONUS_4 = 200.00 number of transactions and then dividing the result by the number of shifts worked. 10 11 employeeName = input("Enter employee's name: ") 12 shiftString = input("Enter number of shifts: ") 13 transactString = input("Enter number of transaction 14 dollarString = input("Enter transactions dollar val Productivity Score Bonus s30 $50.00 15 16 numShifts = float(shiftString) 17 numTransactions = float(transactString) 18 dollarValue = float(dollarString) 31 - 69 $75.00 70 - 199 $100.00 19 # Write your code here 2200 $200.00 20 21 # Output. 22 print("Employee Name: " + employeeName) 23 print("Employee Bonus: $" + str(bonus)) 24 Instructions 1. Make sure the file EmployeeBonus.py is selected and open. 2. Variables have been declared for you, and the input statements and print statements have been written. Read them over carefully before you proceed to the next step. 3. Design the logic and write the rest of the program using a nested if statement. 4. Execute the program by clicking the Run button at the bottom of the screen. 5. Enter the following as input:
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
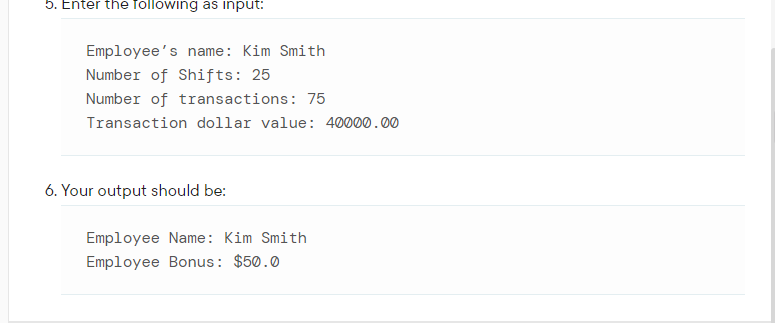
Transcribed Image Text:5. Enter the following as input:
Employee's name: Kim Smith
Number of Shifts: 25
Number of transactions: 75
Transaction dollar value: 40000.00
6. Your output should be:
Employee Name: Kim Smith
Employee Bonus: $50.0
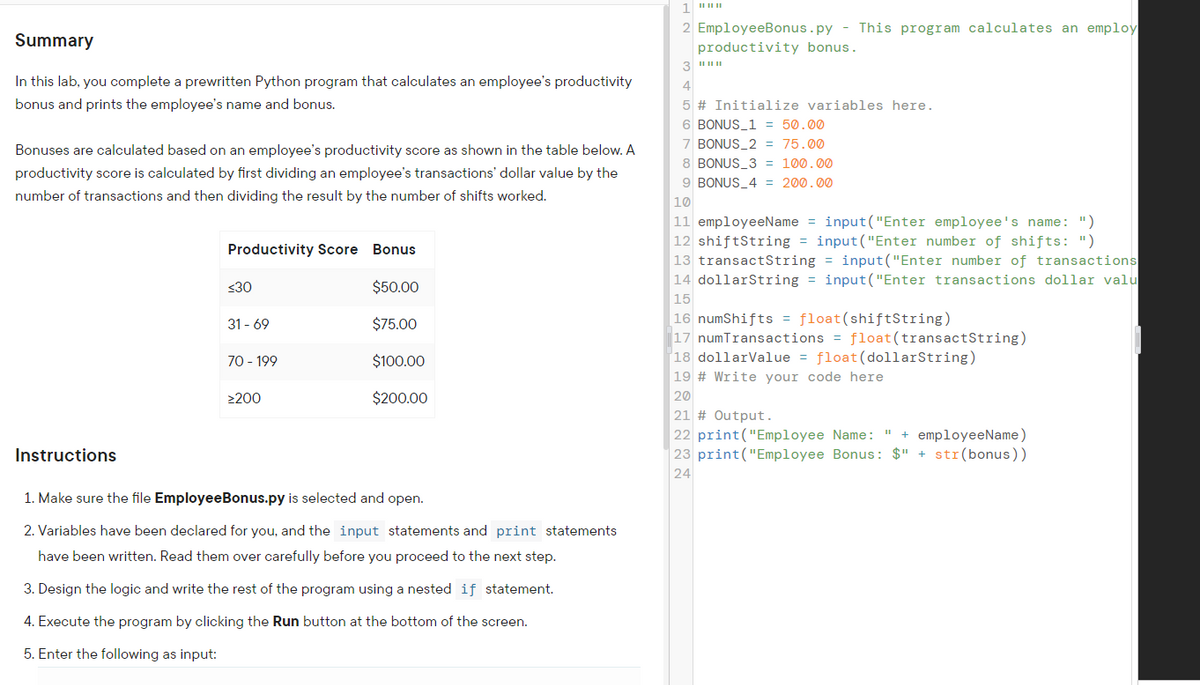
Transcribed Image Text:2 EmployeeBonus.py - This program calculates an employ
Summary
productivity bonus.
In this lab, you complete a prewritten Python program that calculates an employee's productivity
4
bonus and prints the employee's name and bonus.
5 # Initialize variables here.
6 BONUS_1 = 50.00
7 BONUS_2 = 75.00
Bonuses are calculated based on an employee's productivity score as shown in the table below. A
8 BONUS_3 = 100.00
productivity score is calculated by first dividing an employee's transactions' dollar value by the
9 BONUS_4 = 200.00
number of transactions and then dividing the result by the number of shifts worked.
10
11 employeeName = input("Enter employee's name: ")
12 shiftString = input("Enter number of shifts: ")
13 transactString = input("Enter number of transactions
14 dollarString = input("Enter transactions dollar valu
15
|16 numShifts = float(shiftString)
|17 numTransactions = float(transactString)
|18 dollarValue = float(dollarString)
Productivity Score Bonus
<30
$50.00
31 - 69
$75.00
70 - 199
$100.00
19 # Write your code here
20
21 # Output.
22 print("Employee Name: " + employeeName)
23 print("Employee Bonus: $" + str(bonus))
24
2200
$200.00
Instructions
1. Make sure the file EmployeeBonus.py is selected and open.
2. Variables have been declared for you, and the input statements and print statements
have been written. Read them over carefully before you proceed to the next step.
3. Design the logic and write the rest of the program using a nested if statement.
4. Execute the program by clicking the Run button at the bottom of the screen.
5. Enter the following as input:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
While the answer works correctly, when it's checking for the "nested if" statements, it fails. Any other ways to do this? I've tried this way and the nested part passes but the returned answers are incorrect
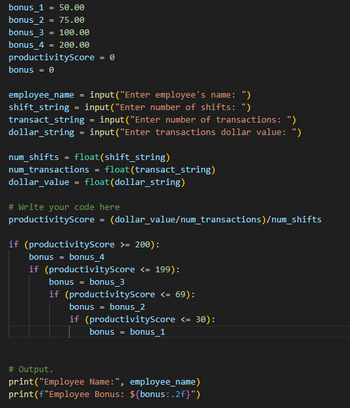
Transcribed Image Text:bonus_1
=
50.00
bonus_2 = 75.00
bonus_3 = 100.00
bonus 4 = 200.00
productivityScore = 0
bonus = 0
employee_name = input("Enter employee's name: ")
shift_string = input("Enter number of shifts: ")
transact_string = input("Enter number of transactions: ")
dollar_string = input("Enter transactions dollar value: ")
num_shifts = float(shift_string)
num_transactions = float(transact_string)
dollar_value = float(dollar_string)
# Write your code here
productivityScore = (dollar_value/num_transactions)/num_shifts
if (productivityScore >= 200):
bonus = bonus_4
if (productivityScore <= 199):
bonus = bonus_3
if (productivityScore <= 69):
bonus = bonus_2
if (productivityScore <= 30):
bonus = bonus_1
# Output.
print("Employee Name:", employee_name)
print("Employee Bonus: ${bonus: .2f}")
Solution
Follow-up Question
cant find the error
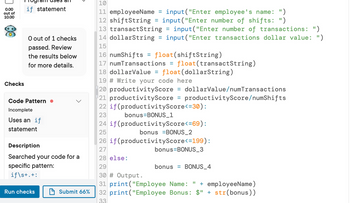
Transcribed Image Text:0.00
out of
10.00
Checks
if statement
O out of 1 checks
passed. Review
the results below
for more details.
Code Pattern
Incomplete
Uses an if
statement
Description
Searched your code for a
specific pattern:
if\s+.+:
Run checks
Submit 66%
10
11 employeeName
input("Enter employee's name: ")
12 shiftString = input("Enter number of shifts: ")
13 transactString = input ("Enter number of transactions: ")
14 dollarString
input("Enter transactions dollar value: ")
15
=
=
16 numShifts = float (shiftString)
17 numTransactions = float(transactString)
18 dollarValue = float(dollarString)
19 # Write your code here
20 productivity Score = dollarValue/numTransactions
=
productivityScore/numShifts
21 productivity Score
22 if (productivityScore<=30):
23
bonus BONUS_1
24 if (productivityScore<=69):
25
bonus =BONUS_2
26 if (productivity Score <=199):
27
bonus=BONUS_3
28 else:
29
30 # Output.
II
31 print("Employee Name: + employeeName)
32 print("Employee Bonus: $" + str(bonus))
33
bonus = BONUS_4
Solution
Recommended textbooks for you
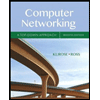
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
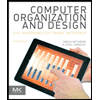
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
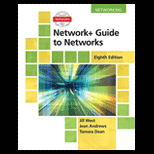
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
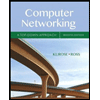
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
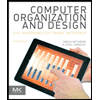
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
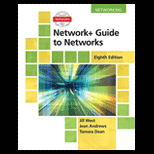
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
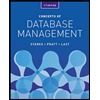
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
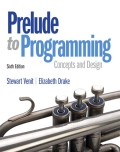
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
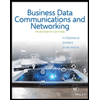
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY