Task 1: Docking We would like to define a universal interface for our equipment and drones for docking. This way, when we develop new vehicles or stations we can implement the universal interface and know that it will behave in the same way. Instructions Create a Java interface called Dockable which has the following methods specified: a method called canDock that takes no parameters and returns a boolean a method called dock that takes a Drone as a parameter and returns an integer Task 2: Drones and Stations Now we want to test our docking interface, but first we want to define an abstract class for our drones from which we will model all of our designs after. Instructions Create an abstract class called Drone that has the following properties: a private attribute called name of type String a private attribute called weight of type double appropriate getters/setters for the attributes an abstract method called printInfo an abstract method called calculateTotalWeight that returns a double Create a subclass of Drone called AerialDockingStation that uses the Dockable interface Make sure that the AerialDockingStation class has the following properties: a private array of Drone objects that represents the docked drones The index of each object represents its docking number/bay Implement all of the necessary methods in AerialDockingStation canDock returns true if there is space for a drone to dock or false if the docking array is full dock must take a Drone as a parameter and add it to the array of docked drones: if the drone is added to the array, return the index of the drone if the drone cannot be added to the array return -1 calculateTotalWeight must return the weight of the AerialDockingStation that includes the weight of the docked drones if the AerialDockingStation weighs 2000 kg and it has one drone docked that weighs 400 kg then calculateTotalWeight should return 2400 printInfo must print out the name of the AerialDockingStation and a list of the docked drones (see sample output) if no drones are docked then it should print none Create a subclass of Drone called DeliveryDrone that implements the abstract methods of the Drone class: printInfo should print out the name and weight of the DeliveryDrone calculateTotalWeight should just return the weight of the DeliveryDrone Hint: the docking array may be partially filled. Sample Input/Output The following demo code produces the sample output: publicclass AssignmentDemo {publicstaticvoidmain(String[] args){ AerialDockingStation ads =newAerialDockingStation("ADS",4.0f,5); DeliveryDrone dragon =newDeliveryDrone("Dragon",0.9f);System.out.println("> Station info:"); ads.printInfo();System.out.println("> A drone can dock at the station: "+ ads.canDock());System.out.println("> Approaching drone info:"); dragon.printInfo();int bayNumber = ads.dock(dragon);System.out.println("> Drone docked in bay number "+ bayNumber);System.out.println("> Station info:"); ads.printInfo();System.out.println("> A drone can dock at the station: "+ ads.canDock());}}
Task 1: Docking We would like to define a universal interface for our equipment and drones for docking. This way, when we develop new vehicles or stations we can implement the universal interface and know that it will behave in the same way. Instructions Create a Java interface called Dockable which has the following methods specified: a method called canDock that takes no parameters and returns a boolean a method called dock that takes a Drone as a parameter and returns an integer Task 2: Drones and Stations Now we want to test our docking interface, but first we want to define an abstract class for our drones from which we will model all of our designs after. Instructions Create an abstract class called Drone that has the following properties: a private attribute called name of type String a private attribute called weight of type double appropriate getters/setters for the attributes an abstract method called printInfo an abstract method called calculateTotalWeight that returns a double Create a subclass of Drone called AerialDockingStation that uses the Dockable interface Make sure that the AerialDockingStation class has the following properties: a private array of Drone objects that represents the docked drones The index of each object represents its docking number/bay Implement all of the necessary methods in AerialDockingStation canDock returns true if there is space for a drone to dock or false if the docking array is full dock must take a Drone as a parameter and add it to the array of docked drones: if the drone is added to the array, return the index of the drone if the drone cannot be added to the array return -1 calculateTotalWeight must return the weight of the AerialDockingStation that includes the weight of the docked drones if the AerialDockingStation weighs 2000 kg and it has one drone docked that weighs 400 kg then calculateTotalWeight should return 2400 printInfo must print out the name of the AerialDockingStation and a list of the docked drones (see sample output) if no drones are docked then it should print none Create a subclass of Drone called DeliveryDrone that implements the abstract methods of the Drone class: printInfo should print out the name and weight of the DeliveryDrone calculateTotalWeight should just return the weight of the DeliveryDrone Hint: the docking array may be partially filled. Sample Input/Output The following demo code produces the sample output: publicclass AssignmentDemo {publicstaticvoidmain(String[] args){ AerialDockingStation ads =newAerialDockingStation("ADS",4.0f,5); DeliveryDrone dragon =newDeliveryDrone("Dragon",0.9f);System.out.println("> Station info:"); ads.printInfo();System.out.println("> A drone can dock at the station: "+ ads.canDock());System.out.println("> Approaching drone info:"); dragon.printInfo();int bayNumber = ads.dock(dragon);System.out.println("> Drone docked in bay number "+ bayNumber);System.out.println("> Station info:"); ads.printInfo();System.out.println("> A drone can dock at the station: "+ ads.canDock());}}
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Task 1: Docking
We would like to define a universal interface for our equipment and drones for docking. This way, when we develop new vehicles or stations we can implement the universal interface and know that it will behave in the same way.
Instructions
- Create a Java interface called Dockable which has the following methods specified:
- a method called canDock that takes no parameters and returns a boolean
- a method called dock that takes a Drone as a parameter and returns an integer
Task 2: Drones and Stations
Now we want to test our docking interface, but first we want to define an abstract class for our drones from which we will model all of our designs after.
Instructions
- Create an abstract class called Drone that has the following properties:
- a private attribute called name of type String
- a private attribute called weight of type double
- appropriate getters/setters for the attributes
- an abstract method called printInfo
- an abstract method called calculateTotalWeight that returns a double
- Create a subclass of Drone called AerialDockingStation that uses the Dockable interface
- Make sure that the AerialDockingStation class has the following properties:
- a private array of Drone objects that represents the docked drones
- The index of each object represents its docking number/bay
- a private array of Drone objects that represents the docked drones
- Implement all of the necessary methods in AerialDockingStation
- canDock returns true if there is space for a drone to dock or false if the docking array is full
- dock must take a Drone as a parameter and add it to the array of docked drones:
- if the drone is added to the array, return the index of the drone
- if the drone cannot be added to the array return -1
- calculateTotalWeight must return the weight of the AerialDockingStation that includes the weight of the docked drones
- if the AerialDockingStation weighs 2000 kg and it has one drone docked that weighs 400 kg then calculateTotalWeight should return 2400
- printInfo must print out the name of the AerialDockingStation and a list of the docked drones (see sample output)
- if no drones are docked then it should print none
- Create a subclass of Drone called DeliveryDrone that implements the abstract methods of the Drone class:
- printInfo should print out the name and weight of the DeliveryDrone
- calculateTotalWeight should just return the weight of the DeliveryDrone
Hint: the docking array may be partially filled.
Sample Input/Output
The following demo code produces the sample output:
publicclass AssignmentDemo {publicstaticvoidmain(String[] args){ AerialDockingStation ads =newAerialDockingStation("ADS",4.0f,5); DeliveryDrone dragon =newDeliveryDrone("Dragon",0.9f);System.out.println("> Station info:"); ads.printInfo();System.out.println("> A drone can dock at the station: "+ ads.canDock());System.out.println("> Approaching drone info:"); dragon.printInfo();int bayNumber = ads.dock(dragon);System.out.println("> Drone docked in bay number "+ bayNumber);System.out.println("> Station info:"); ads.printInfo();System.out.println("> A drone can dock at the station: "+ ads.canDock());}}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
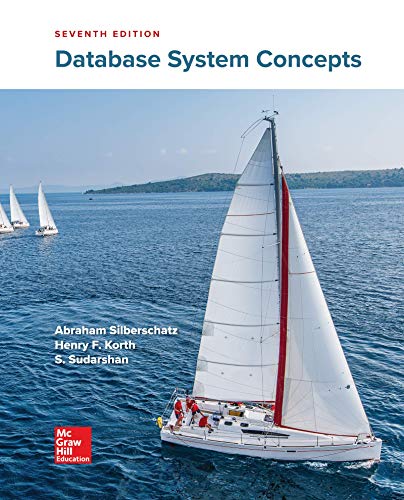
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
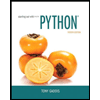
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
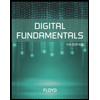
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
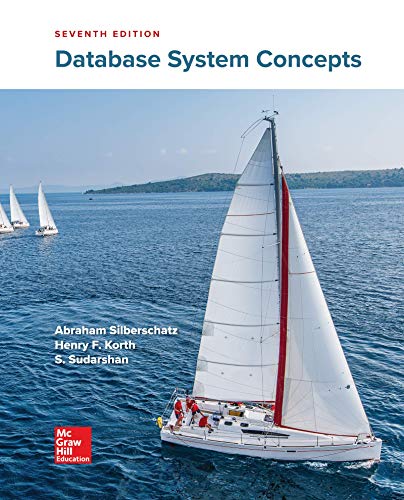
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
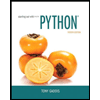
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
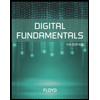
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
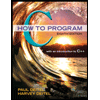
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
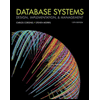
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
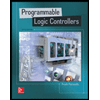
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education