The function prototype is unsigned wins(); It takes no parameter and it shall search the games_ vector and return the number of wins. Were supposed to not use the [] operator, but I dont know how else to do it.
Hello, I need help please because I am trying to make a function that will count the wins of a team and output the wins.
The function prototype is unsigned wins();
It takes no parameter and it shall search the games_
return the number of wins. Were supposed to not use the [] operator, but I dont know how else to do it.
Here is my code for context:
TEAMS.H FILE
#ifndef TEAMS_H
#define TEAMS_H
#include <vector>
#include <iostream>
#include <string>
#include "Game.h"
using namespace std;
class Teams {
private:
string team_name_;
vector <Game> games_;
public:
Teams(const string& new_team);
void addGame(const string&, const string&);
void removeGame(const string&);
void updateScores(const string&, unsigned, unsigned);
unsigned wins();
friend ostream& operator<<(ostream&, const Teams&);
};
#endif
TEAMS.CPP FILE:
#include <iostream>
#include <vector>
#include <string>
#include "Teams.h"
using namespace std;
Teams::Teams(const string& new_team) {
this-> team_name_ = new_team;
}
void Teams::addGame(const string& game_date, const string& opponent_name) {
this->games_.push_back(Game(game_date, opponent_name));
//addGame("01/12/2019", "Falcons");
}
void removeGame(const string&);
void updateScores(const string&, unsigned, unsigned);
unsigned wins();
ostream& operator<<(ostream& output, const Teams& a) {
if (a.games_.size() >0) {
output << a.games_.size();
}
else cout << "There haven't been any games for this team" << endl;
//cout << a; in the main
return output;
}
void Teams::updateScores(const string& game_date, unsigned score, unsigned opponentscore) {
Date game_date_(game_date);
for (int i=0; i < games_.size(); i++) {
if (game_date_ == games_[i].getDate()){
games_[i].setScores(score, opponentscore);
}
else
cout << "No matching date found" << endl;
}
}
(The following is what I have but it isnt working for me):
unsigned wins() {
int wins=0;
int losses=0;
for (int i=0; i < games_.size(); i++) {
//game_[i].setScores();
if (games_[i].own_score_ > games_[i].opponent_score_) {
wins++
}
else if (own_score_ < opponent_score) {
losses++
}
else cout << "The game is tied << endl;"
}
cout << "Wins total: " << wins << "\n" << "Losses total: " << losses << "\n";
}
GAME.CPP FILE
#include <iostream>
#include <string>
#include <sstream>
#include <iomanip>
#include "Game.h"
//#include "Date.h"
using namespace std;
Game::Game(const string& d, const string& name) : game_date_(d), opponent_(name) {
this->own_score_ = -1;
this->opponent_score_ = -1;
}
string Game::toString() const {
stringstream s;
s << left << setw(15) << this->game_date_.toString();
s << setw(25) << this->opponent_;
if (this->status() == 'X') {
s << "Scores not yet available.";
} else {
s << this->status() << ", ";
s << this->own_score_ << '-' << this->opponent_score_;
}
return s.str();
} //end of toString
//returns 'W' for win, 'L' for loss, 'T' for tie, and 'X' for scores not yet available
char Game::status() const {
if (this->own_score_ < 0) {
return 'X'; //game score not yet available
} else if (this->own_score_ > this->opponent_score_) {
return 'W'; //the game was a win.
} else if (this->own_score_ < this->opponent_score_) {
return 'L'; //the game was a loss
} else {
return 'T'; //the game was a tie
}
}
Date Game::getDate() const {
return this->game_date_;
}
void Game::setScores(unsigned own, unsigned opponent) {
this->own_score_ = own;
this->opponent_score_ = opponent;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Thank you this helped a lot. The only error is that now I do not know how to access the private data member
![C Teams.cpp X +
3 #include <string>
4 #include "Teams.h"
5
6
7
8
9
10
11
12 ▼
13
14
15
}
16 void removeGame(const string&);
17
18
19
20 ▼
using namespace std;
Teams :: Teams (const string& new_team) {
this-> team_name_ = new_team;
}
21 ▼
22
23
24
25
26
27
28
29 ▼
30
31 ▼
32 ▼
33
34
35
36
37
38
39
40▼
41
42
43
44 ▼
45
46
47
48▼
49
50
51 ▼
52
53
54
55
56
void Teams ::addGame(const string& game_date, const string& opponent_name) {
this->games_.push_back (Game (game_date, opponent_name));
//addGame("01/12/2019", "Falcons");
void updateScores (const string&, unsigned, unsigned);
unsigned wins();
ostream& operator<<(ostream& output, const Teams& a) {
if (a.games.size() >0) {
output << a.games_.size();
}
else cout << "There haven't been any games for this team" << endl;
//cout << a; in the main
return output;
}
void Teams::updateScores (const string& game_date, unsigned score, unsigned opponentscore) {
Date game_date_(game_date);
for (int i=0; i < games_.size(); i++) {
if (game_date_ == games_[i].getDate()){
games [i].setScores (score, opponentscore);
}
else
cout << "No matching date found" << endl;
}
}
unsigned Teams::wins() {
int wins=0;
int losses=0;
for (int i=0; i < games_.size(); i++)
if (games_[i].own_score_ > games_[i].opponent_score_) {
wins++;
}
else if (own_score_< opponent_score_)
{
losses++;
}
else {
cout << "The game is tied "<< endl;
}
cout << "Wins total: << wins << "\n" << "Losses total: " << losses << "\n";
}
II
> Console x
Shell X +
>sh -c make -s
./Teams.cpp:44:19: error: 'own_score_' is a private member of 'Game'
if (games_[i].own_score_ > games_[i].opponent_score_) {
./Game.h:12:9: note: declared private here
int own_score_, opponent_score_;
./Teams.cpp:44:42: error: 'opponent_score_' is a private member of 'Game'
if (games_[i].own_score_ > games_[i].opponent_score_) {
./Game.h:12:21: note: declared private here
int own_score_, opponent_score_;
./Teams.cpp:47:27: error: use of undeclared identifier 'opponent_score_'
else if (own_score_< opponent_score_)
./Teams.cpp:47:14: error: use of undeclared identifier 'own_score_'
else if (own_score_< opponent_score_)
4 errors generated.
make: *** [Makefile:9: main] Error 1
exit status 2](https://content.bartleby.com/qna-images/question/46c0fb16-724b-4726-818c-9156075ac6cc/2cf7d2ad-c809-43d0-bec5-94e3a461c40f/vmhggbd_thumbnail.jpeg)
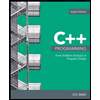
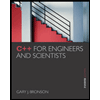
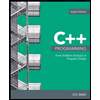
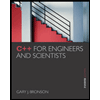