b) Write the method evaluateLight, which computes and returns the status of a light at a given row and column based on the following rules. 1. If the light is on, return false if the number of lights in its column that are on is even including the current light. 2. If the light is off, return true if the number of lights in its column that are on is divisible by three. 3. Otherwise, return the light's current status. For example, suppose that LightBoard sin new LightBoard (7, 5) creates a light board with the initial state shown below, where true represents a light that is on and false represents a light that is off. Lights that are off are shaded. lights 0 1 2 3 4 0 true true false true true 1 true false false true false Sample calls to evaluateLight are shown below. Value Call to evaluateLight Explanation Returned sin.evaluateLight(0, 3); sin.evaluatelight(6, 8); sin.evaluateLight(4, 1); sin.evaluateLight(5, 4); false true false The light is on, and the number of lights that are on in its column is even. The light is off, and the number of lights that are on in its column is divisible by Returns the light's current status. true Returns the light's current status. Class information for this question public class LightBoard private boolean[][] lights public LightBoard(int nunRows, int numCols) public boolean evaluateLight(int row, int col) Complete the evaluateLight method below. /**Evaluates a light in row index row and column index col and returns a status ⚫as described in part (b). Precondition: row and col are valid indexes in lights.
b) Write the method evaluateLight, which computes and returns the status of a light at a given row and column based on the following rules. 1. If the light is on, return false if the number of lights in its column that are on is even including the current light. 2. If the light is off, return true if the number of lights in its column that are on is divisible by three. 3. Otherwise, return the light's current status. For example, suppose that LightBoard sin new LightBoard (7, 5) creates a light board with the initial state shown below, where true represents a light that is on and false represents a light that is off. Lights that are off are shaded. lights 0 1 2 3 4 0 true true false true true 1 true false false true false Sample calls to evaluateLight are shown below. Value Call to evaluateLight Explanation Returned sin.evaluateLight(0, 3); sin.evaluatelight(6, 8); sin.evaluateLight(4, 1); sin.evaluateLight(5, 4); false true false The light is on, and the number of lights that are on in its column is even. The light is off, and the number of lights that are on in its column is divisible by Returns the light's current status. true Returns the light's current status. Class information for this question public class LightBoard private boolean[][] lights public LightBoard(int nunRows, int numCols) public boolean evaluateLight(int row, int col) Complete the evaluateLight method below. /**Evaluates a light in row index row and column index col and returns a status ⚫as described in part (b). Precondition: row and col are valid indexes in lights.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please answer the question in the attachment. It is a past AP Computer Science frq question that has already been answered, but I would like to see another way to approach the problem.
![b) Write the method evaluate Light, which computes and returns the status of a light at a given
row and column based on the following rules.
1. If the light is on, return false if the number of lights in its column that are on is even,
including the current light.
2. If the light is off, return true if the number of lights in its column that are on is divisible by
three.
3. Otherwise, return the light's current status.
For example, suppose that LightBoard sim = new LightBoard (7, 5) creates a light board
with the initial state shown below, where true represents a light that is on and false represents
a light that is off. Lights that are off are shaded.
lights
Sample calls to evaluate light are shown below.
Value
Call to evaluateLight
Explanation
Returned
sim.evaluateLight(0, 3);
sim.evaluateLight(6, 0);
sim.evaluateLight(4, 1);
sim.evaluateLight(5, 4);
false
true
false
The light is on, and the number of lights that are on in its column is even.
The light is off, and the number of lights that are on in its column is divisible by 3.
Returns the light's current status.
true
Returns the light's current status.
0
1
2
3
4
Class information for this question
public class LightBoard
private boolean[] [] lights
public LightBoard(int numRows, int numCols)
public boolean evaluateLight (int row, int col)
Complete the evaluateLight method below.
0
true
true false
true
true
/** Evaluates a light in row index row and column index col and returns a status
×
as described in part (b).
1
true
false
false
true
false
* Precondition: row and col are valid indexes in lights.
public boolean evaluate Light (int row, int col)
2 true
3
true
false
false
false true
false false
4
5 true
true false false
true false
true
true
false true
true true
6 false false false false false](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3d8d9e88-2b16-4dd5-a739-61f31fec5755%2F03a3d244-e8d9-4a71-8bbf-25e0a50f5341%2F8aykmvi_processed.png&w=3840&q=75)
Transcribed Image Text:b) Write the method evaluate Light, which computes and returns the status of a light at a given
row and column based on the following rules.
1. If the light is on, return false if the number of lights in its column that are on is even,
including the current light.
2. If the light is off, return true if the number of lights in its column that are on is divisible by
three.
3. Otherwise, return the light's current status.
For example, suppose that LightBoard sim = new LightBoard (7, 5) creates a light board
with the initial state shown below, where true represents a light that is on and false represents
a light that is off. Lights that are off are shaded.
lights
Sample calls to evaluate light are shown below.
Value
Call to evaluateLight
Explanation
Returned
sim.evaluateLight(0, 3);
sim.evaluateLight(6, 0);
sim.evaluateLight(4, 1);
sim.evaluateLight(5, 4);
false
true
false
The light is on, and the number of lights that are on in its column is even.
The light is off, and the number of lights that are on in its column is divisible by 3.
Returns the light's current status.
true
Returns the light's current status.
0
1
2
3
4
Class information for this question
public class LightBoard
private boolean[] [] lights
public LightBoard(int numRows, int numCols)
public boolean evaluateLight (int row, int col)
Complete the evaluateLight method below.
0
true
true false
true
true
/** Evaluates a light in row index row and column index col and returns a status
×
as described in part (b).
1
true
false
false
true
false
* Precondition: row and col are valid indexes in lights.
public boolean evaluate Light (int row, int col)
2 true
3
true
false
false
false true
false false
4
5 true
true false false
true false
true
true
false true
true true
6 false false false false false
![The LightBoard class models a two-dimensional display of lights, where each light is either on or
off, as represented by a Boolean value. You will implement a constructor to initialize the display and
a method to evaluate a light.
public boolean evaluateLight (int row, int col)
{ /* to be implemented in part (b) */ }
public class LightBoard
{
/** The lights on the board, where true represents on and false
represents off.
// There may be additional instance variables, constructors, and methods
not shown.
*/
private boolean ( ) ( ) lights;
/** Constructs a LightBoard object having numRows rows and numCols
columns.
* Precondition: numRows > 0, numCols > 0
/*
Postcondition: each light has a 40% probability of being set to on.
public LightBoard (int numRows, int numCols)
(a)
Write the constructor for the LightBoard class, which initializes lights so that each light is
set to on with a 40% probability. The notation lights [r] [c] represents the array element at row
I and column c.
Complete the LightBoard constructor below.
/** Constructs a LightBoard object having numRows rows and numCols
columns.
Precondition: numRows > 0, numCols > 0
* Postcondition: each light has a 40% probability of being set to on.
{
/* to be implemented in part (a) */ }
*/
/** Evaluates a light in row index row and column index col and returns
a status
*
as described in part (b).
* Precondition: row and col are valid indexes in lights.
public LightBoard (int numRows, int numCols)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3d8d9e88-2b16-4dd5-a739-61f31fec5755%2F03a3d244-e8d9-4a71-8bbf-25e0a50f5341%2Fxbnbzg6_processed.png&w=3840&q=75)
Transcribed Image Text:The LightBoard class models a two-dimensional display of lights, where each light is either on or
off, as represented by a Boolean value. You will implement a constructor to initialize the display and
a method to evaluate a light.
public boolean evaluateLight (int row, int col)
{ /* to be implemented in part (b) */ }
public class LightBoard
{
/** The lights on the board, where true represents on and false
represents off.
// There may be additional instance variables, constructors, and methods
not shown.
*/
private boolean ( ) ( ) lights;
/** Constructs a LightBoard object having numRows rows and numCols
columns.
* Precondition: numRows > 0, numCols > 0
/*
Postcondition: each light has a 40% probability of being set to on.
public LightBoard (int numRows, int numCols)
(a)
Write the constructor for the LightBoard class, which initializes lights so that each light is
set to on with a 40% probability. The notation lights [r] [c] represents the array element at row
I and column c.
Complete the LightBoard constructor below.
/** Constructs a LightBoard object having numRows rows and numCols
columns.
Precondition: numRows > 0, numCols > 0
* Postcondition: each light has a 40% probability of being set to on.
{
/* to be implemented in part (a) */ }
*/
/** Evaluates a light in row index row and column index col and returns
a status
*
as described in part (b).
* Precondition: row and col are valid indexes in lights.
public LightBoard (int numRows, int numCols)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
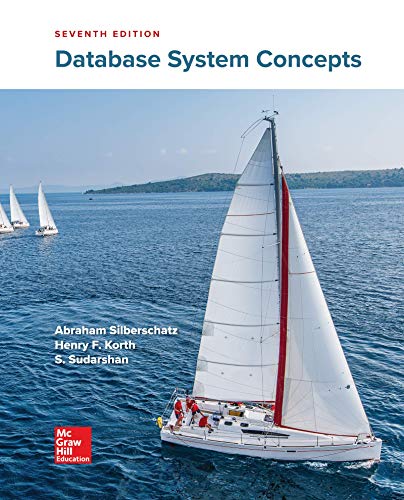
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
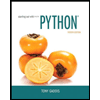
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
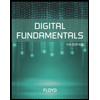
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
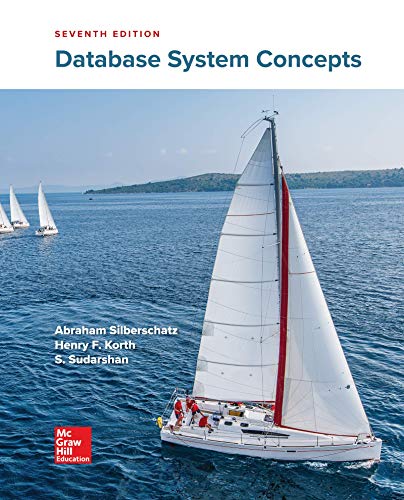
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
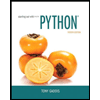
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
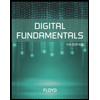
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
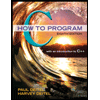
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
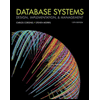
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
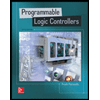
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education