The local taqueria wants you to write a program which tracks the number of burritos they sell each day and help them analyze their business. Unlike last time, they don't know the types of burritos they will sell, or how many types of burritos there are, until the day of sale. Your main() should: - ask the user for the number of different burrito types sold - get the names of types from the user, e.g. "carnitas", "chicken", "vegetarian", etc., and ask the user for the number of burritos sold of each type of that day. To store all this, you must use two dynamically allocated arrays (i.e. use new to allocate memory for them, and delete [] to delete them when finished). You need one string array to store the names of the burrito types, and one int array to store the number of burritos sold. Finally, create a function which will take a string pointer, an int pointer, and an int indicating the number of types as parameters. void printReport(string *burritosPtr, int *salesPtr, int numTypes) The string pointer burritosPtr points to the first element of an array of burrito type strings, the int pointer salesPtr points to the first element an array of sales per type, and the int numTypes is how many elements in each array. The function prints out a daily report listing sales for each burrito type and total number of burritos sold. Call this function from main() with your pointers to demonstrate their usage. Hint: assuming variable numTypes contains the number of burrito types, here is how to dynamically allocate an array of type string with numTypes elements: string *names = new string[numTypes]; I will leave it to you to figure out how to dynamically allocate an array of type int with numTypes elements.
Operations
In mathematics and computer science, an operation is an event that is carried out to satisfy a given task. Basic operations of a computer system are input, processing, output, storage, and control.
Basic Operators
An operator is a symbol that indicates an operation to be performed. We are familiar with operators in mathematics; operators used in computer programming are—in many ways—similar to mathematical operators.
Division Operator
We all learnt about division—and the division operator—in school. You probably know of both these symbols as representing division:
Modulus Operator
Modulus can be represented either as (mod or modulo) in computing operation. Modulus comes under arithmetic operations. Any number or variable which produces absolute value is modulus functionality. Magnitude of any function is totally changed by modulo operator as it changes even negative value to positive.
Operators
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
Write a C++
The local taqueria wants you to write a program which tracks the number of burritos they sell each day and help them analyze their business. Unlike last time, they don't know the types of burritos they will sell, or how many types of burritos there are, until the day of sale. Your main() should:
- ask the user for the number of different burrito types sold
- get the names of types from the user, e.g. "carnitas", "chicken", "vegetarian", etc., and ask the user for the number of burritos sold of each type of that day. To store all this, you must use two dynamically allocated arrays (i.e. use new to allocate memory for them, and delete [] to delete them when finished). You need one string array to store the names of the burrito types, and one int array to store the number of burritos sold.
Finally, create a function which will take a string pointer, an int pointer, and an int indicating the number of types as parameters.
void printReport(string *burritosPtr, int *salesPtr, int numTypes)
The string pointer burritosPtr points to the first element of an array of burrito type strings, the int pointer salesPtr points to the first element an array of sales per type, and the int numTypes is how many elements in each array. The function prints out a daily report listing sales for each burrito type and total number of burritos sold. Call this function from main() with your pointers to demonstrate their usage.
Hint: assuming variable numTypes contains the number of burrito types, here is how to dynamically allocate an array of type string with numTypes elements:
string *names = new string[numTypes];
I will leave it to you to figure out how to dynamically allocate an array of type int with numTypes elements.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

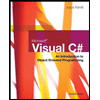
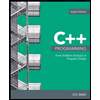
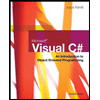
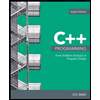