The names and student numbers of students are save in a text file called stnumbers.txt. Example of the content of the text file: Peterson 20570856 Johnson 12345678 Suku 87654321 Westley 12345678 Venter 87654321 Mokoena 79012400 Makubela 29813360 Botha 30489059 Bradley 30350069 Manana 30530679 Shabalala 28863496 Smith 87873909 Nilsson 30989698 Makwela 30256607 Govender 30048117 Ntumba 30598303 Ramsamy 29952239 Skosana 29982995 Jameson 30228484 Xulu 29092248 Wasserman 27469352 Bester 28615425 Babane 27154033 Maboya 29897890 Mahlangu 30031338 Majavu 30165970 Myene 30954177 Motaung 30907276 Ramaroka 30804507 Radebe 30007674 Sekake 30017416 Zwane 30038227 Shuro 30238072 Viljoen 28881389 Sithole 45688555 Write a function called displayData() to receive the array and number of elements as parameters and display the names and student numbers of the students with a heading and neatly spaced. Write a function, isValid(), which receives a number as parameter and determines whether the number is a valid student number or not. Use the following validity test to determine the validity of a student number: Student number: 20570856 sum = (2*8)+(0*7)+(5*6)+(7*5)+(0*4)+(8*3)+(5*2)+(6*1) result = sum%11 If the result equals 0, the number is a valid student number; if the result is not equal to 0, the number is an invalid student number. The function MUST use a loop to analyse the number by looking at each individual digit in the number. The function returns the Boolean value true (valid) or the Boolean value false (invalid) to the calling statement. Hint: Repeatedly use integer division (/) with 10 and the remainder after integer division (%) with 10 to isolate the individual digits of the number. Write a function, writeToFiles(), which receives the array of Student structs and the number of elements in the array. If the student number is valid, the name and student number must be written to an output file, validNumbers.txt. If the student number is invalid, the name and student number must be written to an output file, invalidNumbers.txt. Display the number of valid and the number of invalid number. The function returns no value to the calling statement. The above functions (readFile() and writeToFile()) must now be implemented in the main function (main()) as follows: 1. Declare a Student struct with a name field (string or array of char) and a stNumber field (integer). 2. Create an array of type Student struct and make provision for a maximum of 100 elements. 3. Call the readFile() function to read the contents of the text file “numbers.txt” to the array and return the number of elements read to the array. 4. Call the writeToFile() function to write the names and valid students numbers and names and invalid student numbers to two separate text files.
The names and student numbers of students are save in a text file called stnumbers.txt. Example of the content of the text file:
Peterson
20570856
Johnson
12345678
Suku
87654321
Westley
12345678
Venter
87654321
Mokoena
79012400
Makubela
29813360
Botha
30489059
Bradley
30350069
Manana
30530679
Shabalala
28863496
Smith
87873909
Nilsson
30989698
Makwela
30256607
Govender
30048117
Ntumba
30598303
Ramsamy
29952239
Skosana
29982995
Jameson
30228484
Xulu
29092248
Wasserman
27469352
Bester
28615425
Babane
27154033
Maboya
29897890
Mahlangu
30031338
Majavu
30165970
Myene
30954177
Motaung
30907276
Ramaroka
30804507
Radebe
30007674
Sekake
30017416
Zwane
30038227
Shuro
30238072
Viljoen
28881389
Sithole
45688555
Write a function called displayData() to receive the array and number of elements as parameters and display the names and student numbers of the students with a heading and neatly spaced.
Write a function, isValid(), which receives a number as parameter and determines whether the number is a valid student number or not. Use the following validity test to determine the validity of a student number: Student number: 20570856 sum = (2*8)+(0*7)+(5*6)+(7*5)+(0*4)+(8*3)+(5*2)+(6*1) result = sum%11 If the result equals 0, the number is a valid student number; if the result is not equal to 0, the number is an invalid student number. The function MUST use a loop to analyse the number by looking at each individual digit in the number. The function returns the Boolean value true (valid) or the Boolean value false (invalid) to the calling statement. Hint: Repeatedly use integer division (/) with 10 and the remainder after integer division (%) with 10 to isolate the individual digits of the number.
Write a function, writeToFiles(), which receives the array of Student structs and the number of elements in the array. If the student number is valid, the name and student number must be written to an output file, validNumbers.txt. If the student number is invalid, the name and student number must be written to an output file, invalidNumbers.txt. Display the number of valid and the number of invalid number. The function returns no value to the calling statement.
The above functions (readFile() and writeToFile()) must now be implemented in the main function (main()) as follows: 1. Declare a Student struct with a name field (string or array of char) and a stNumber field (integer). 2. Create an array of type Student struct and make provision for a maximum of 100 elements. 3. Call the readFile() function to read the contents of the text file “numbers.txt” to the array and return the number of elements read to the array. 4. Call the writeToFile() function to write the names and valid students numbers and names and invalid student numbers to two separate text files.
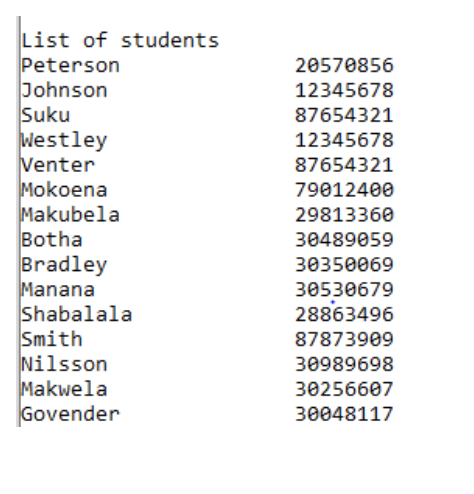

Step by step
Solved in 2 steps with 1 images

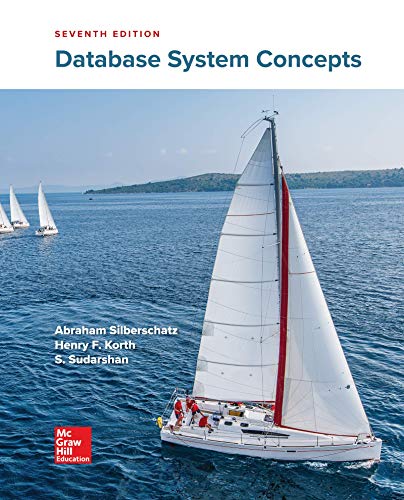
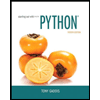
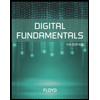
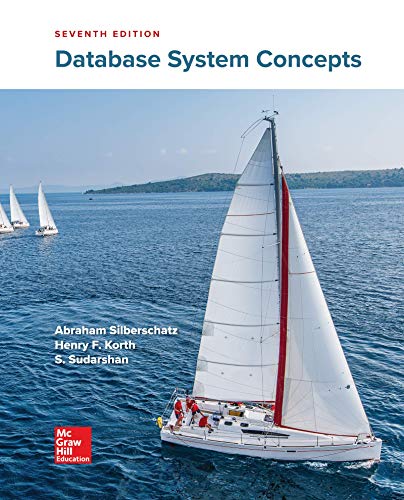
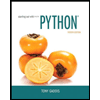
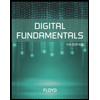
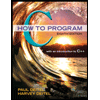
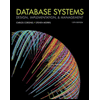
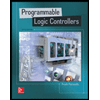