The Problem A Zeckendorf number is defined for all positive integers as the number of Fibonacci numbers which must be added to equal a given number k. So, the positive integer 28 is the sum of three Fibonacci numbers (21, 5, and 2), so the Zeckendorf number for k = 28 is three. Wikipedia is a satisfactory reference for our purposes for more details of the Zeckendorf and Fibonacci numbers. In both cases, you do not need to read the entire Wikipedia entry. Your assignment is to write two ARM assembly language functions which calculate Zeckendorf and Fibonacci numbers. Zeck function The first function, which should be named zeck, receives an integer parameter in register zero. This will be the variable k we discussed above. Your code should return the Zeckendorf number for k in register zero when complete. If the parameter k is zero, return zero. If the parameter k is negative, return minus 1. If the parameter k is too large to calculate, return minus 1. Fib function The other function, which should be named fib, receives an integer parameter in register zero. This will also be the variable k we discussed above. Your code should return the Fibonacci number which is less than or equal to k in register zero when complete. If the parameter k is zero, return zero. If the parameter k is negative, return minus 1. If the parameter k is too large to calculate, return minus 1. Notes Your program must only use positive integers for all calculations and the result variable. The zeck function should call the fib function to obtain the nearest Fibonacci number to k. You may find it easiest to develop the fib function first. It then subtracts the Fibonacci number from the current value of k, adds one to a counter (the Zeckendorf number), and continues calling fib() until k is zero. You may find it easiest to look up the Fibonacci numbers in a table within the fib function. The largest Fibonacci number that fits in 31 bits is 1836311903. Test Program Your functions should be tested within the following program: MOV R0,#33 ; this will be the value of k BL zeck END Requirements Correct numeric result for all cases Correct rejection of invalid input Following naming and message format standards Test Data You should test your program with various integer values. Include test cases of: k = one less than a given Fibonacci number, k = that Fibonacci number, k = one more than that Fibonacci number, zero, negative numbers, 1836311902 1836311903, and 1836311904 It appear
Operations
In mathematics and computer science, an operation is an event that is carried out to satisfy a given task. Basic operations of a computer system are input, processing, output, storage, and control.
Basic Operators
An operator is a symbol that indicates an operation to be performed. We are familiar with operators in mathematics; operators used in computer programming are—in many ways—similar to mathematical operators.
Division Operator
We all learnt about division—and the division operator—in school. You probably know of both these symbols as representing division:
Modulus Operator
Modulus can be represented either as (mod or modulo) in computing operation. Modulus comes under arithmetic operations. Any number or variable which produces absolute value is modulus functionality. Magnitude of any function is totally changed by modulo operator as it changes even negative value to positive.
Operators
In the realm of programming, operators refer to the symbols that perform some function. They are tasked with instructing the compiler on the type of action that needs to be performed on the values passed as operands. Operators can be used in mathematical formulas and equations. In programming languages like Python, C, and Java, a variety of operators are defined.
The Problem
A Zeckendorf number is defined for all positive integers as the number of Fibonacci numbers which must be added to equal a given number k. So, the positive integer 28 is the sum of three Fibonacci numbers (21, 5, and 2), so the Zeckendorf number for k = 28 is three.
Wikipedia is a satisfactory reference for our purposes for more details of the Zeckendorf and Fibonacci numbers. In both cases, you do not need to read the entire Wikipedia entry.
Your assignment is to write two ARM assembly language functions which calculate Zeckendorf and Fibonacci numbers.
Zeck function
The first function, which should be named zeck, receives an integer parameter in register zero. This will be the variable k we discussed above. Your code should return the Zeckendorf number for k in register zero when complete.
If the parameter k is zero, return zero.
If the parameter k is negative, return minus 1.
If the parameter k is too large to calculate, return minus 1.
Fib function
The other function, which should be named fib, receives an integer parameter in register zero. This will also be the variable k we discussed above. Your code should return the Fibonacci number which is less than or equal to k in register zero when complete.
If the parameter k is zero, return zero.
If the parameter k is negative, return minus 1.
If the parameter k is too large to calculate, return minus 1.
Notes
Your
The zeck function should call the fib function to obtain the nearest Fibonacci number to k. You may find it easiest to develop the fib function first. It then subtracts the Fibonacci number from the current value of k, adds one to a counter (the Zeckendorf number), and continues calling fib() until k is zero.
You may find it easiest to look up the Fibonacci numbers in a table within the fib function.
The largest Fibonacci number that fits in 31 bits is 1836311903.
Test Program
Your functions should be tested within the following program:
MOV R0,#33 ; this will be the value of k
BL zeck
END
Requirements
- Correct numeric result for all cases
- Correct rejection of invalid input
- Following naming and message format standards
Test Data
You should test your program with various integer values. Include test cases of:
- k = one less than a given Fibonacci number,
- k = that Fibonacci number,
- k = one more than that Fibonacci number,
- zero,
- negative numbers,
- 1836311902
- 1836311903, and
- 1836311904
It appears that the largest positive integer which fits into an ARM-modified immediate value is 2139095040.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

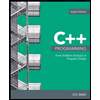
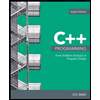