The program must read through a timesheet file (Timesheets.csv) and process the payroll by reading the employee file (Employees.csv) and process each timesheet with the appropriate employee. EmpNo FirstName LastName Position Mgr. Logistias Proje ct Mgr. Pay Rate Exempt 72.12 Y EmpNo Hours 10011 Femi Adu 10011 10002 Zanab Ali 74.52 Y 10002 73.08 Y 144.23 Y Asaad 10007 Fadi 10012 Helen Accountant 10007 40 Billingsly CIO 10012 40 An employee gets overtime when they have worked more than 40 hours Overtime pay is calculated only for nonexempt employees using this formula: Gross Pay = (40'Wage) + ((Hours-40) *Wage"1.5) Regular pay is calculated using this formula: Gross Pay = (Hours"Wage) for exempt employees and Gross Pay = (40"Wage) for exempt employees The files are provided below. Since I used the first rows of each datafile as a header, to name the fields, you need to skip that row to process the data. That can be done many ways. You must research formatting output in Python to get the proper output. This will be the only material you can use that was not covered in class. The output should look like this: Emp No First 10011 Femi 10002 Zanab 10007 Fadl Gross Pay 45.00 72.12 3,425.70 40.00 74.52 2,980.80 40.00 73.08 2,923.20 Billingsly 40.00 144.23 5,769.20 Hours Wage Last Adu Ali Asaad 10012 Helen
The program must read through a timesheet file (Timesheets.csv) and process the payroll by reading the employee file (Employees.csv) and process each timesheet with the appropriate employee. EmpNo FirstName LastName Position Mgr. Logistias Proje ct Mgr. Pay Rate Exempt 72.12 Y EmpNo Hours 10011 Femi Adu 10011 10002 Zanab Ali 74.52 Y 10002 73.08 Y 144.23 Y Asaad 10007 Fadi 10012 Helen Accountant 10007 40 Billingsly CIO 10012 40 An employee gets overtime when they have worked more than 40 hours Overtime pay is calculated only for nonexempt employees using this formula: Gross Pay = (40'Wage) + ((Hours-40) *Wage"1.5) Regular pay is calculated using this formula: Gross Pay = (Hours"Wage) for exempt employees and Gross Pay = (40"Wage) for exempt employees The files are provided below. Since I used the first rows of each datafile as a header, to name the fields, you need to skip that row to process the data. That can be done many ways. You must research formatting output in Python to get the proper output. This will be the only material you can use that was not covered in class. The output should look like this: Emp No First 10011 Femi 10002 Zanab 10007 Fadl Gross Pay 45.00 72.12 3,425.70 40.00 74.52 2,980.80 40.00 73.08 2,923.20 Billingsly 40.00 144.23 5,769.20 Hours Wage Last Adu Ali Asaad 10012 Helen
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
how do i add a tuple to this code?
![#open file to read data of employees
empFile = open ('Employees.csv')
#copy all line into list emp
emp = empFile.readlines ()
empHeading = emp[0]
#delete first line from line list
del emp [0]
empHeading = empHeading.strip ().split (",")
timesheetFile = open ('Timesheets.csv')
timesheet = timesheetFile.readlines ()
timesheetHeading = timesheet [0]
del timesheet [0]
timesheetHeading = timesheetHeading.strip ().split(",")
#declare a list to hold data
empInfo = []
#it will iterate each row in Employees.csv
for row in range (len (emp)) :
#remove ' \n' from end
data = emp[row].strip ()
#split into list
data = data.split(",")
timeData = timesheet [row].strip ()
timeData = timeData.split (",")
#append empNo, first, last, hours,wage and gross pay
temp = []
temp.append (data [0])
temp.append (data[1])
temp.append (data [2])
temp.append (timeData [1])
temp.append (data [4])
#calculate gross pay by checking Exempt
if (data [5] == 'Y'):
gpay = (40*float (data[4]))+((float(timeData[1]) - 40) *float (data [4])*1.5)
else:
gpay = float (timeData[1]) * float (data [ 4] )
temp.append (gpay)
#add perticullar employee into empInfo list
empInfo.append (temp)
#display header of employee
print ("{:<7}{:<12}{:<12}{:<7}{:<7}{:<10}".format ("Emp No", "First","Last","Hours","Wage","Gross Pay"))
#it will display information of employee
for e in empInfo:
print ("{:<7}{:<12}{:<12}{:<7}{:<7}{:<10}".format (e [0], e[1],e[2],"%.2f"%float(e[3]),"%.2f"%float (e[4]),'{:,.2f}'.format (e[5])))](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fac7f0f6e-6d20-42e1-81bb-7961a6d3ee59%2F14a71d5f-0980-4008-924d-11e8d29d0968%2Fq3nxy3_processed.png&w=3840&q=75)
Transcribed Image Text:#open file to read data of employees
empFile = open ('Employees.csv')
#copy all line into list emp
emp = empFile.readlines ()
empHeading = emp[0]
#delete first line from line list
del emp [0]
empHeading = empHeading.strip ().split (",")
timesheetFile = open ('Timesheets.csv')
timesheet = timesheetFile.readlines ()
timesheetHeading = timesheet [0]
del timesheet [0]
timesheetHeading = timesheetHeading.strip ().split(",")
#declare a list to hold data
empInfo = []
#it will iterate each row in Employees.csv
for row in range (len (emp)) :
#remove ' \n' from end
data = emp[row].strip ()
#split into list
data = data.split(",")
timeData = timesheet [row].strip ()
timeData = timeData.split (",")
#append empNo, first, last, hours,wage and gross pay
temp = []
temp.append (data [0])
temp.append (data[1])
temp.append (data [2])
temp.append (timeData [1])
temp.append (data [4])
#calculate gross pay by checking Exempt
if (data [5] == 'Y'):
gpay = (40*float (data[4]))+((float(timeData[1]) - 40) *float (data [4])*1.5)
else:
gpay = float (timeData[1]) * float (data [ 4] )
temp.append (gpay)
#add perticullar employee into empInfo list
empInfo.append (temp)
#display header of employee
print ("{:<7}{:<12}{:<12}{:<7}{:<7}{:<10}".format ("Emp No", "First","Last","Hours","Wage","Gross Pay"))
#it will display information of employee
for e in empInfo:
print ("{:<7}{:<12}{:<12}{:<7}{:<7}{:<10}".format (e [0], e[1],e[2],"%.2f"%float(e[3]),"%.2f"%float (e[4]),'{:,.2f}'.format (e[5])))
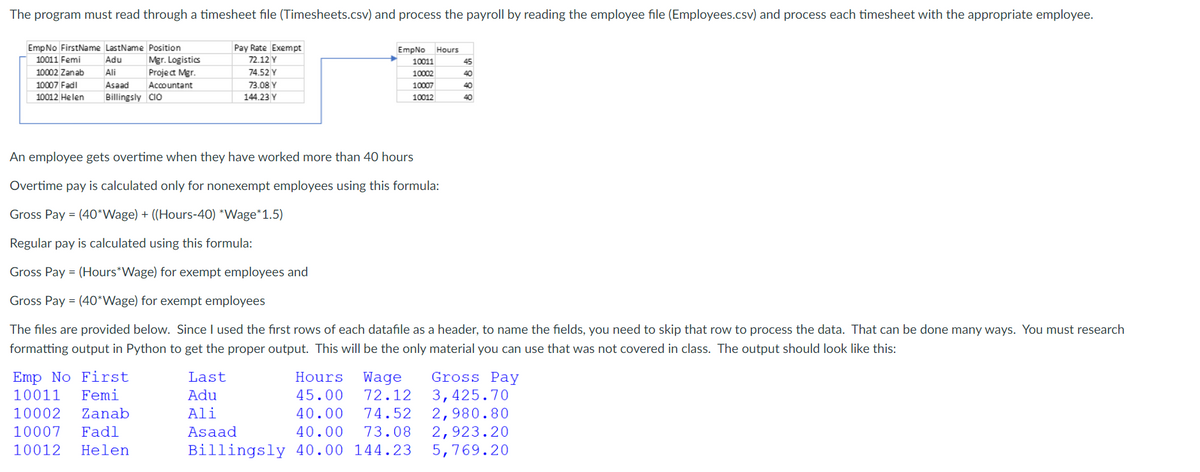
Transcribed Image Text:The program must read through a timesheet file (Timesheets.csv) and process the payroll by reading the employee file (Employees.csv) and process each timesheet with the appropriate employee.
EmpNo FirstName LastName Position
Mgr. Logistics
Project Mgr.
Pay Rate Exempt
EmpNo Hours
10011 Femi
Adu
72.12 Y
10011
45
10002 Zanab
Ali
74.52 Y
10002
40
10007 Fadl
Asaad
Accountant
73.08 Y
10007
40
10012 Helen
Billingsly CIo
144.23 Y
10012
40
An employee gets overtime when they have worked more than 40 hours
Overtime pay is calculated only for nonexempt employees using this formula:
Gross Pay = (40*Wage) + ((Hours-40) *Wage*1.5)
Regular pay is calculated using this formula:
Gross Pay = (Hours*Wage) for exempt employees and
Gross Pay = (40*Wage) for exempt employees
The files are provided below. Since I used the first rows of each datafile as a header, to name the fields, you need to skip that row to process the data. That can be done many ways. You must research
formatting output in Python to get the proper output. This will be the only material you can use that was not covered in class. The output should look like this:
Emp No First
10011
Femi
Hours
Gross Pay
Wage
72.12
Last
3,425.70
2,980.80
2,923.20
5,769.20
Adu
45.00
10002
Zanab
Ali
40.00
74.52
10007
Fadl
Asaad
40.00
73.08
10012
Helen
Billingsly 40.00 144.23
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 6 steps with 5 images

Recommended textbooks for you
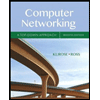
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
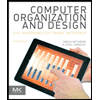
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
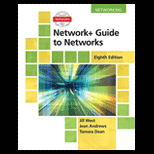
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
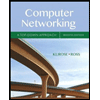
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
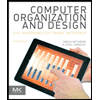
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
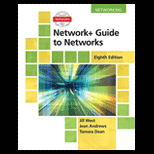
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
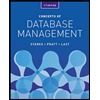
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
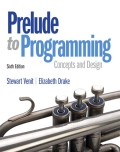
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
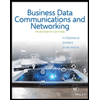
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY