The starter code does contain formatting mistakes .The output must match the sample output exactly.The user must be prompted for a seed for the random number generator and then an indeterminate number of integer-guesses until the game terminates (by matching an integer-guess to the target-integer).Any integer may be used as the seed and as a guess.The program must not discriminate a user's guess. A guess that contradicts a hint ('too high' or 'too low') or is repeated is a valid guess.Instance variables/constants may not be used. In general, content not formally presented in the class heretofore cannot be used. main() method must call the following two programmer-defined methods (methods that you write):A process non-void method that returns the number of user-attempts to guess the random-number correctly and an output void method that displays the final results of the program (the number of guess required to correctly guess the random number).process method must call the following programmer-defined method (a method that you write): An input non-void method to (depending upon the String variable/literal used as a parameter (or argument) to specify the appropriate prompt to the user) will either be called once in order to return the user-defined seed or be called an indefinite number of times in order to return a user-defined guess. the main() method should only contain: a salutation (to the user), the constant called MAX that defines the maximum possible random number, the Scanner object and a primitive variable (used to store the number of guesses) declaration, the two method calls to the programmer-defined methods (the methods that you write), and one method call to the Scanner object (that does not read from the input buffer!). the main() method may either contain the loop to govern the game (and be subject to a deduction) or call a process non-void method that contains the loop to govern the game and return the number of guess required to correctly guess the random number. Use the most appropriate loop to manage the game. the only Scanner object must be declared in the main() method; do not move the Scanner object declaration nor declare another Scanner object in the program The constant called MAX must be declared atop the main() method and effectively used in the program to generate random numbers. In general, avoid using 'magic numbers' in code because 'magic numbers' reduce code-readability. An integer constant/variable should not appear in the code except for possibly common integers, such as 0 or 1. every method should be preceded with a comment that includes a description of the method purpose and the value returned (if applicable)! Comments should also be included in the body of each method. Development Strategy: Initially, complete the code entirely in the main() method. Only after the code is functional (using only the main() method) should the code be parsed into methods; add methods singularly (one at a time) and carefully vet the the code with the added method before adding the next method until all methods are added. Input-Method: In order to reduce the number of input-methods from three to one, simply use a String variable/literal as a parameter (or argument) that specifies the prompt needed for the particular user-defined integer (seed to the random number generator (RNG), a guess, or a response corresponding to a desire to repeat the game). String variable as a Parameter: Use a String variable (instead of a literal) for the input method to obtain the user-defined guesses to differentiate the first guess from the subsequent guesses that may be too low or too high. Random Object Scope: The process-method should contain the Random object.Hardcode the Target-Number: During program-development, hardcode the target-number in order to ensure the loop and logic ('too high' or 'too low') are correct. Then, after the loop and logic are functional, make the number random. Debug Print-Statements: Employ a debug print-statements that temporarily displays the target-number and the user-defined guesses during program-development to ensure program-integrity (especially regarding the hint ('too high' or 'too low'). Remove the debug print-statements before submitting the assignment! SAMPLE OUTPUT Welcome to the Guessing Game! Enter a seed: 33 Please enter a number between 1 and 100: 5 Too high. Guess again: 4 Congratulations. You guessed correctly! You needed 2 guesses. Welcome to the Guessing Game! Enter a seed: 50 Please enter a number between 1 and 100: 50 Too high. Guess again: 25 Too high. Guess again: 12 Too low. Guess again: 19 Too high. Guess again: 15 Too low. Guess again: 17 Too low. Guess again: 19 Too high. Guess again: 18 Congratulations. You guessed correctly! You needed 8 guesses. Welcome to the Guessing Game! Enter a seed: 0 Please enter a number between 1 and 100: 61 Congratulations. You guessed correctly! You needed only 1 guess.
The starter code does contain formatting mistakes .The output must match the sample output exactly.The user must be prompted for a seed for the random number generator and then an indeterminate number of integer-guesses until the game terminates (by matching an integer-guess to the target-integer).Any integer may be used as the seed and as a guess.The
-
-
- a salutation (to the user),
- the constant called MAX that defines the maximum possible random number,
- the Scanner object and a primitive variable (used to store the number of guesses) declaration,
- the two method calls to the programmer-defined methods (the methods that you write), and
- one method call to the Scanner object (that does not read from the input buffer!).
- the main() method may either
- contain the loop to govern the game (and be subject to a deduction) or
- call a process non-void method that contains the loop to govern the game and return the number of guess required to correctly guess the random number.
- Use the most appropriate loop to manage the game.
- the only Scanner object must be declared in the main() method; do not move the Scanner object declaration nor declare another Scanner object in the program
- The constant called MAX must be declared atop the main() method and effectively used in the program to generate random numbers. In general, avoid using 'magic numbers' in code because 'magic numbers' reduce code-readability. An integer constant/variable should not appear in the code except for possibly common integers, such as 0 or 1.
- every method should be preceded with a comment that includes a description of the method purpose and the value returned (if applicable)! Comments should also be included in the body of each method.
-
Development Strategy: Initially, complete the code entirely in the main() method. Only after the code is functional (using only the main() method) should the code be parsed into methods; add methods singularly (one at a time) and carefully vet the the code with the added method before adding the next method until all methods are added.
Input-Method: In order to reduce the number of input-methods from three to one, simply use a String variable/literal as a parameter (or argument) that specifies the prompt needed for the particular user-defined integer (seed to the random number generator (RNG), a guess, or a response corresponding to a desire to repeat the game).
String variable as a Parameter: Use a String variable (instead of a literal) for the input method to obtain the user-defined guesses to differentiate the first guess from the subsequent guesses that may be too low or too high.
Random Object Scope: The process-method should contain the Random object.Hardcode the Target-Number: During program-development, hardcode the target-number in order to ensure the loop and logic ('too high' or 'too low') are correct. Then, after the loop and logic are functional, make the number random.
Debug Print-Statements: Employ a debug print-statements that temporarily displays the target-number and the user-defined guesses during program-development to ensure program-integrity (especially regarding the hint ('too high' or 'too low'). Remove the debug print-statements before submitting the assignment!
SAMPLE OUTPUT
Welcome to the Guessing Game!
Enter a seed:
33
Please enter a number between 1 and 100:
5
Too high. Guess again:
4
Congratulations. You guessed correctly!
You needed 2 guesses.
Welcome to the Guessing Game!
Enter a seed:
50
Please enter a number between 1 and 100:
50
Too high. Guess again:
25
Too high. Guess again:
12
Too low. Guess again:
19
Too high. Guess again:
15
Too low. Guess again:
17
Too low. Guess again:
19
Too high. Guess again:
18
Congratulations. You guessed correctly!
You needed 8 guesses.
Welcome to the Guessing Game!
Enter a seed:
0
Please enter a number between 1 and 100:
61
Congratulations. You guessed correctly!
You needed only 1 guess.
![2 import java.util.Scanner;
3
4 public class GuessANumber_Part1 {
5 public static void main(String[] args) {
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31 }
// ========== INSERT YOUR CODE HERE ==========
//Declare a Constant
????
//Declare the Scanner object.
//Note: Only one Scanner object may exist in a program and the Scanner
declaration must remain in the main () method but be used in an
"input()" method.
// Call the following TWO (2) methods (and then write the method
// implementations) that:
// 1) uses the Scanner object and the constant to play the game and get the
//
number of user-attempts to guess the random-number correctly.
//
Note: This method will
//
//
//
//
//
Note: Methods can indeed call other methods!
// 2) uses a local variable that stores the number of guesses to print the
program results.
//
???
???
A) call the input-method once to obtain the seed for the random
number generator (RNG) and
B) call the input-method an indeterminate number of times to
obtain the user-defined guesses.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fe163e186-9e90-41af-8d77-4602b0f64151%2F6b365ad8-c3a3-4d00-af19-3c6fb41846fd%2Fl1v971p_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

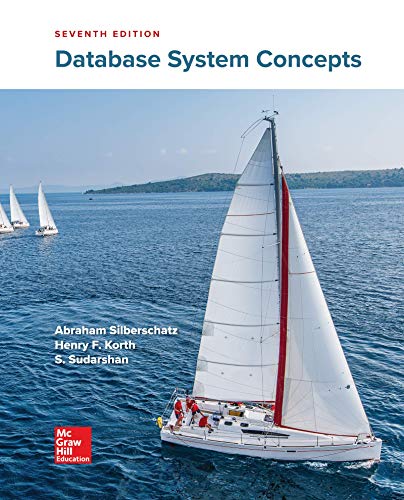
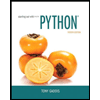
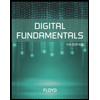
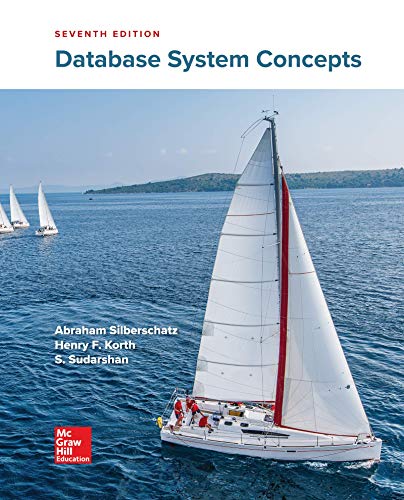
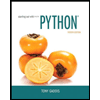
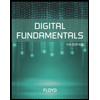
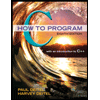
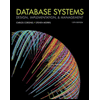
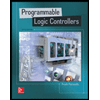