I keep getting the error "undefined reference to 'main'". What am I doing wrong? Here is the code, it is in the language of C #include #include #include char * mult_digits (const int *digits, int n) { //impliment me char *product = (char *) malloc (sizeof (digits) * (n + 2)); char *productstring = (char *) malloc (sizeof (digits) * (n + 2)); int carry = 0; int previousnum = 1; int length; int k; int tempproduct; int tempremainder; for (length = length - 1; length >= 0; length--) { int tempnum = (digits[length]); tempproduct = (tempnum * previousnum) + carry; tempremainder = ((tempproduct) % 10); carry = ((tempproduct - tempremainder) / 10); previousnum = tempproduct; product[length] = (tempremainder) + '0'; } if (carry = 0) { for (k = 0; k <= strlen (product); k++) { productstring[0] = carry + '0'; productstring[k + 1] = product[k]; printf ("%s \n", productstring); return productstring; } } printf ("%s \n", product); return product; free (product); free (productstring); }
I keep getting the error "undefined reference to 'main'". What am I doing wrong?
Here is the code, it is in the language of C
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
char *
mult_digits (const int *digits, int n)
{
//impliment me
char *product = (char *) malloc (sizeof (digits) * (n + 2));
char *productstring = (char *) malloc (sizeof (digits) * (n + 2));
int carry = 0;
int previousnum = 1;
int length;
int k;
int tempproduct;
int tempremainder;
for (length = length - 1; length >= 0; length--)
{
int tempnum = (digits[length]);
tempproduct = (tempnum * previousnum) + carry;
tempremainder = ((tempproduct) % 10);
carry = ((tempproduct - tempremainder) / 10);
previousnum = tempproduct;
product[length] = (tempremainder) + '0';
}
if (carry = 0)
{
for (k = 0; k <= strlen (product); k++)
{
productstring[0] = carry + '0';
productstring[k + 1] = product[k];
printf ("%s \n", productstring);
return productstring;
}
}
printf ("%s \n", product);
return product;
free (product);
free (productstring);
}

#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main(){
char *
mult_digits (const int *digits, int n)
{
//impliment me
char *product = (char *) malloc (sizeof (digits) * (n + 2));
char *productstring = (char *) malloc (sizeof (digits) * (n + 2));
int carry = 0;
int previousnum = 1;
int length;
scanf("%d", &length);
int k;
int tempproduct;
int tempremainder;
for (length = length - 1; length >= 0; length--)
{
int tempnum = (digits[length]);
tempproduct = (tempnum * previousnum) + carry;
tempremainder = ((tempproduct) % 10);
carry = ((tempproduct - tempremainder) / 10);
previousnum = tempproduct;
product[length] = (tempremainder) + '0';
}
if (carry = 0)
{
for (k = 0; k <= strlen (product); k++)
{
productstring[0] = carry + '0';
productstring[k + 1] = product[k];
printf ("%s \n", productstring);
return productstring;
}
}
printf ("%s \n", productstring);
return product;
free (product);
free (productstring);
}
}
Step by step
Solved in 2 steps

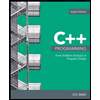
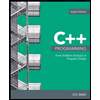